Cannot create files on Android with Xamarin
Solution 1
I finally realized that File.create()
was not the problem. I had code like this:
string tmpFilePath = FilesDir.AbsolutePath.stringByAppendingPath (f.Path);
Java.IO.File tmpFile = new Java.IO.File( tmpFilePath);
tmpFile.Mkdirs ();
Yet, Mkdirs()
does not only create all intermediate directories – as I had assumed – but also creates a directory at the file path itself. So the file could not be created because there already was a directory with the same name.
The correct way is:
string tmpFile = FilesDir.AbsolutePath.stringByAppendingPath (f.Path);
Java.IO.File tmpParentFolder = new Java.IO.File(tmpFile).getParentFile();
tmpParentFolder.Mkdirs ();
In my defense, an FileExistsAndIsDirectory
exception would have been much more helpful than the UnauthorizedAccessException
Solution 2
You should use Environment or IsolatedStorage. For example:
var path = Environment.GetFolderPath(Environment.SpecialFolder.ApplicationData);
var filename = Path.Combine(path, "newFile.png");
Solution 3
I am coding Xamarin with VS2013. I had the access denied error for a directory created with the application I am writing. My application creates a directory called /storage/emulated/0/POIApp
by concatenating via:
System.IO.Path.Combine(Android.OS.Environment.ExternalStorageDirectory.Path, "POIApp");
I found that I had to use VS2013 to edit the "properties" of my application (POIApp), i.e., right-click the project icon in the solution explorer; choose properties from the pop-up menu. A new tab appears in the main VS2013 window. On the left there are a few choices, e.g., Application, Android Manifest, Android Options, Build, etc. Choose "Android Manifest". At the bottom of the main panel is a section "required permissions". My problem was solved when I checked "READ_EXTERNAL_STORAGE" and "WRITE_EXTERNAL_STORAGE".
Solution 4
Add the following permission to Android.Manifest file
<uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE" />
Solution 5
Using Mono, I think must be the same as in Xamarin Studio.
var path = Environment.GetFolderPath(Environment.SpecialFolder.ApplicationData);
File.Create(path + "newFile.png");
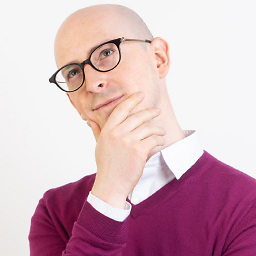
codingFriend1
📓 Developer of Loqbooq, a Slack and web app for decision logging and ADR. 💬 Maker of the vocabulary learning app Wokabulary for iPhone, iPad and Mac. 🧁 Developer of Macarons, a chat roulette app for Slack. 📝 Contributor to the open source text editor Tincta for Mac. 🛡 Blogging on security for most people on passwort1.de
Updated on July 11, 2020Comments
-
codingFriend1 over 3 years
I have a Xamarin-Studio App for Android and I simply want to download files and save them locally. But when I try to create a file in the
files
folder I get an exception:File.Create("data/data/com.company.app/files/newFile.png");
gives me:
System.UnauthorizedAccessException Access to the path 'data/data/com.company.app/files/newFile.png' is denied.
What am I doing wrong?
-
codingFriend1 almost 10 yearsDoesn't that give me the file path to the SD-Card? I need to write the the internal storage within the Apps bundle.
-
Krumelur almost 10 yearsTry
Environment.GetFolderPath(Environment.SpecialFolder.MyDocuments);
-
Ashish-BeJovial about 7 yearsSystem.Environment Does not contain the method GetFolderPath
-
Ashish-BeJovial about 7 years