Saving Bitmap to File - Xamarin, Monodroid
20,946
Solution 1
This here is a slim way to export a Bitmap
as PNG
-file to the sd-card using only C#
stuff:
void ExportBitmapAsPNG(Bitmap bitmap)
{
var sdCardPath = Android.OS.Environment.ExternalStorageDirectory.AbsolutePath;
var filePath = System.IO.Path.Combine(sdCardPath, "test.png");
var stream = new FileStream(filePath, FileMode.Create);
bitmap.Compress(Bitmap.CompressFormat.Png, 100, stream);
stream.Close();
}
Solution 2
Change:
String filePath = storageDirectory.ToString() + "APPNAME.png";
To:
String filePath = Path.Combine(storageDirectory.ToString(), "APPNAME.png");
Your original code appends the file name to the last folder in the path name without adding a path separator. For example, path of \data\data\sdcard01
would create a file path of \data\data\sdcard01APPNAME.png
. Using Path.Combine()
ensures that a path separator is used when appending directories.
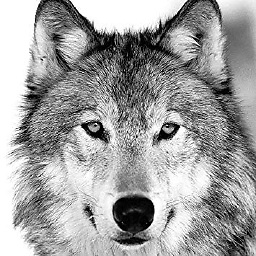
Author by
Coova
Updated on July 09, 2022Comments
-
Coova 11 months
I am trying to save a bitmap image to a directory (gallery) inside my phone. The app is being developed in Xamarin so the code is C#.
I cant seem to figure out how to make a directory, and save a Bitmap. Any suggestions?
public void createBitmap(View view){ view.DrawingCacheEnabled = true; view.BuildDrawingCache (true); Bitmap m_Bitmap = view.GetDrawingCache(true); String storagePath = Android.OS.Environment.ExternalStorageDirectory.AbsolutePath; Java.IO.File storageDirectory = new Java.IO.File(storagePath); //storageDirectory.mkdirs (); //save the bitmap //MemoryStream stream = new MemoryStream (); //m_Bitmap.Compress (Bitmap.CompressFormat.Png, 100, stream); //stream.Close(); try{ String filePath = storageDirectory.ToString() + "APPNAME.png"; FileOutputStream fos = new FileOutputStream (filePath); BufferedOutputStream bos = new BufferedOutputStream(fos); m_Bitmap.Compress (Bitmap.CompressFormat.Png, 100, bos); bos.Flush(); bos.Close(); } catch (Java.IO.FileNotFoundException e) { System.Console.WriteLine ("FILENOTFOUND"); } catch (Java.IO.IOException e) { System.Console.WriteLine ("IOEXCEPTION"); }
-
gonzobrains almost 7 yearshow do you write out the raw bmp data without compressing?
-
kaolick almost 7 years@gonzobrains I don't know if or how that's possible. Sorry.
-
Wessam El Mahdy over 5 yearsYou should release steam and bitmap from memory by using ".Dispose()" or it may be better to use "using(){}".
-
Fnr over 5 yearswhen I compress it I'm losing the quality of the image. is there a way to save it without passing to this compress function? Also, the post @testing showed only shows to convert to byte array by passing it to the compress function.
-
testing over 5 years@Fabiotk: Have you tried
CopyPixelsToBuffer()
? Here is a post about this. -
xanderh almost 4 years@Fabiotk another option you might try is GetByteArrayFromImage().