CAPL writing to Text file
12,860
Solution 1
Here is a simple example of writing the value of variable as it is incremented.
variables
{
dword logFile; // logfile handle
int i;
char results[1024]; // store our formatted string
}
on start
{
setFilePath("C:\\Demo\\Results", 2); // 2 = read & write
logFile = openFileWrite("Results.txt", 2); // 2 = append file
for (i = 0; i < 10; i++ )
{
snprintf(results, 1024, "The value of 'i' is %d\n", i);
filePutString(results, 1024, logFile);
}
fileClose(logFile);
}
Solution 2
Open your file in a different access mode.
OpenFileWrite ("Log.txt",2);
If mode=0 writing can be executed in ASCII mode;
if mode=1 writing can be executed in binary mode. An already existing file will be overwritten.
mode=2 to append data at the end of the file use for ASCII mode.
mode=3 to append data at the end of the file for binary mode.
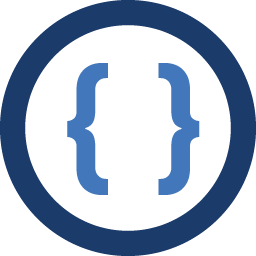
Author by
Admin
Updated on June 07, 2022Comments
-
Admin almost 2 years
I am pretty new to the CAPL language. Hence, I am having problems with writing data to .txt file. This is the code I wrote:
includes { } variables { message Generate_Num Gen; message Logger Logs; msTimer tim_100ms; msTimer tim_500ms; int time_over; // 500 ms flag. dword handle=0; float val=0; long index=0; int val_arr[512]; } on start { time_over=0; setWritePath("C:\\Users\\Türker\\Desktop\\soft_pro"); handle= openFileWrite("Log.txt",1); Gen.Num_Data=1; } on envVar Logger_Sw { if(@this) { setTimer(tim_500ms,500); setTimer(tim_100ms,100); } else { cancelTimer(tim_500ms); cancelTimer(tim_100ms); } } on envVar Save_txt { if(@Save_txt==1) { int i; float val_n; for(i=0;i<elcount(val_arr);i++) { val_n=val_arr[i]; writeProfileFloat("1","1",val_n,"Log.txt"); } } } on timer tim_100ms { output(Gen); Gen.Num_Data++; setTimer(tim_100ms,100); } on message Generate_Num { if(time_over==1) { val=this.Num_Data; val_arr[index]=val; Logs.Logged_Val=val; output(Logs); index++; time_over=0; } } on timer tim_500ms { time_over=1; setTimer(tim_500ms,500); }
I store the sampled data in "val_arr" array. I want this to be written in a text file. However, it always overwrites the written data. The "Save_txt" environmental variable does the writing job.
Cheers.