Send Message/ Inject a message with CAPL
Solution 1
Sending message in CAPL
You can send a message (or multiple messages) on any event, for example, a keypress, the reception of another message, the reception of an error frame, or a timer expiring. Sending an event message involves creating an event procedure, declaring a message to send, and sending the message in the event procedure. The message can be declared as a global variable so that it can be accessed in any event procedure. As shown in the message object section, you can declare a message’s structure in your program or you can use an associated database. In this example, we will declare one of each in the Global Variables window
variables
{
message EngineData msg1; // Defined in database
message 0x101 msg2; // Standard message 101 (hex)
message 0x105x msg3; // Extended message 105 (hex)
}
Now, to send a message, we only need to put one of these lines into an event procedure:
output(msg1);
output(msg2);
Of course, we can also add data to the message before we send it. The EngineData message has signals defined in the database, but the other message does not. Therefore, we have to use two different methods to add data to the messages.
msg1.EngSpeed.phys = 1000;
msg1.EngTemp.phys = 150;
msg1.IdleRunning = 1;
output(msg1);
msg2.DLC = 4; // Allocate 4 data bytes in msg2
msg2.byte(0) = 0x16; // First word is 16 hex
msg2.byte(1) = 7; // Second word is 7 decimal
output(msg2);
Responding Message
on message 0x101 // This is the identifier of your response
{
if(this.byte(0) == 0x16)
{
write("Response is the expected!");
}
}
or
on message msg2 // This is the identifier of your response
{
if(this.byte(0) == 0x16)
{
write("Response is the expected!");
}
}
Solution 2
You can use as given below,
variables
{
message BCMmsg01 msg_BCMmsg01; // declaration of message into a variable
}
on key 'z'
{
msg_BCMmsg01.C_AutoLockCmd = 3; // assign the value to the message
output(msg_BCMmsg01); //send the message to the CAN bus
}
Hopefully I clarified your question. Let me know if you need more clarification.
Solution 3
Joe showed the way the message (in this case the hex value) is sent. If you want to see the response, you need to know the response ID (for example 0x62C)
on message 0x62C /* This is the identifier of your response */
{
if(this.byte(X) == 0xYY) { /* X is the byte of the response you are interested and Y the value of that byte*/
write("Response is the expected!");
}
I hope this answers your question.
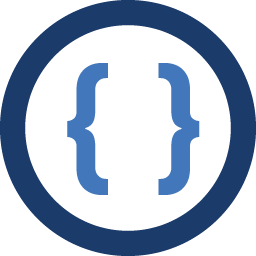
Admin
Updated on July 28, 2022Comments
-
Admin almost 2 years
I am new in CANoe, and also CAPL language. But I want to ask you: How can I send a message with CAPL on the network of ECU's. For example: I want to send an Hex number( it is a question for an ECU) and after that I want to see the response of this question.
I don't know if I was very clear but, if you have any answers I would be grateful.