casting NSInteger into NSString
Solution 1
A string and an integer are fundamentally different data types, and casting in Objective-C won't do a conversion for you in this case, it'll just lie to the compiler about what's happening (so it compiles) but at runtime it blows up.
You can embed an integer directly into a format string by using %d
instead of %@
:
tempString = [NSString stringWithFormat:@"%@%d%@%d", part1,num1, part2, num2];
NSInteger is just a fancy name for a regular "int" (number). An NSString is an object reference to a string object. Some numeric types (int and floating point) can be sort of converted between eachother directly in C like this, but these two aren't inter-operable at all. It sounds like you might be coming from a more permissive language? :)
Solution 2
answered Feb 22 '12 at 22:34, Ben Zotto:
A string and an integer are fundamentally different data types, and casting in Objective-C won't do a conversion for you in this case, it'll just lie to the compiler about what's happening (so it compiles) but at runtime it blows up.
You can embed an integer directly into a format string by using %d instead of %@:
tempString = [NSString stringWithFormat:@"%@%d%@%d", part1,num1, part2, num2];
NSInteger is just a fancy name for a regular "int" (number). An NSString is an object reference to a string object. Some numeric types (int and floating point) can be sort of converted between eachother directly in C like this, but these two aren't inter-operable at all. It sounds like you might be coming from a more permissive language? :)
"Keep in mind that @"%d" will only work on 32 bit. Once you start using NSInteger for compatibility if you ever compile for a 64 bit platform, you should use @"%ld" as your format specifier." by Marc Charbonneau
So, solution:
tempString = [NSString stringWithFormat:@"%@%ld%@%ld", part1, (long)num1, part2, (long)num2];
Source: String Programming Guide for Cocoa - String Format Specifiers (Requires iPhone developer registration)
Solution 3
You have to use %d
for integer and not %@
.
So, [NSString stringWithFormat:@"%@%d%@%d", part1,num1, part2, num2];
is the correct code to format your string.
Hope it helps.
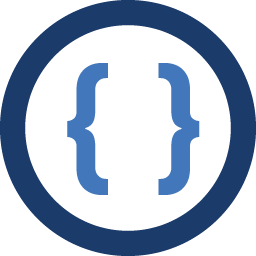
Admin
Updated on January 22, 2020Comments
-
Admin over 4 years
I'm trying to make one string out of several different parts. This below is what i have right now. I don't get any errors in my code but as soon as i run this and do the event that triggers it i get
EXC_BAD_ACCESS
. Is there another way of casting thisNSInteger
into aNSString
?NSString *part1, *part2, *tempString; NSInteger num1; NSInteger num2; part1=@"some"; part2=@"text"; tempString = [NSString stringWithFormat:@"%@%@%@%@", part1, (NSString *)num1, part2, (NSString *)num2];
-
mcandre over 10 yearsAlmost correct. You don't need to explicitly cast the numbers to long, if you simply use the
%ld
formatters. -
Alex Shaffer almost 10 yearsWith Xcode 5.1.1, a warning is generated if NSInteger values aren't cast to (long) when using %ld formatters.