Catalina C++: Using <cmath> headers yield error: no member named 'signbit' in the global namespace
Solution 1
I'm curious: What compiler are you using? What's the value of CMAKE_OSX_SYSROOT
?
I'm fairly convinced this is the result of a wrong CMAKE_OSX_SYSROOT
. I had the problem you're describing when using python bindings for clang (where CMake doesn't manage the compiler call), but I managed to recreate the error in CMake by doing:
set(CMAKE_OSX_SYSROOT "") # Reset.
I solved my problem by following the answers to this question: Cannot compile R packages with c++ code after updating to macOS Catalina.
To summarise: On Catalina, /usr/include
is purged and protected by SIP. Thus, any project that expects the C headers to be found there will fail to compile. If I remember correctly, Apple recommends to file bug reports to projects that expect C headers in /usr/include
.
You must point the build system of the code you're trying to compile to the right headers:
(1) Make sure Xcode is up to date. There's no telling what an outdated Xcode on Catalina might do to your build environment.
(2) Use the -isysroot /sdk/path
compiler flag, where /sdk/path
is the result of xcrun --show-sdk-path
. I'm not sure what CMake's best practice is, but try doing
set(CMAKE_OSX_SYSROOT /sdk/path)
or
set(CMAKE_CXX_FLAGS "[...] -isysroot /sdk/path")
If that solves the problem, you may want to look for a better way to do this in CMake.
Of course, if you're adventurous, you could also disable SIP, as suggested in the answer to my question: /usr/include missing on macOS Catalina (with Xcode 11)
Solution 2
Using the command:
gcc -Wp,-v -E -
my #include <...> search sequence:
/usr/local/include
/Applications/Xcode.app/Contents/Developer/Toolchains/XcodeDefault.xctoolchain/usr/lib/clang/10.0.1/include
/Applications/Xcode.app/Contents/Developer/Toolchains/XcodeDefault.xctoolchain/usr/include
/Library/Developer/CommandLineTools/SDKs/MacOSX10.15.sdk/usr/include
/Library/Developer/CommandLineTools/SDKs/MacOSX10.15.sdk/System/Library/Frameworks (framework directory)
The reason of #include error is described below:
-
#include<cmath>
resides in/Applications/Xcode.app/Contents/Developer/Toolchains/XcodeDefault.xctoolchain/usr/include/c++/v1
- It includes
<math.h>
. - It searches
/usr/local/include
directory as this is the first directory to search. There is amath.h
in/usr/local/include/c++/9.3.0/
directory - It tries to use this.
- But expectation was to use the
math.h
of the same directory/Applications/Xcode.app/Contents/Developer/Toolchains/XcodeDefault.xctoolchain/usr/include/c++/v1
- The
math.h
of/Applications/Xcode.app/Contents/Developer/Toolchains/XcodeDefault.xctoolchain/usr/include/c++/v1
includemath.h
of/usr/local/include
using#include_next<math.h>
- As wrong
math.h
is included/linked with/Applications/Xcode.app/Contents/Developer/Toolchains/XcodeDefault.xctoolchain/usr/include/c++/v1/cmath
, the compilation error happens
The fix:
-
If we can alter the search order of
#include<...>
to search/Applications/Xcode.app/Contents/Developer/Toolchains/XcodeDefault.xctoolchain/usr/include/c++/v1
at first, it can be fixed. -
Using
#include</Applications/Xcode.app/Contents/Developer/Toolchains/XcodeDefault.xctoolchain/usr/include/c++/v1/math.h>
instead of<math.h>
in/Applications/Xcode.app/Contents/Developer/Toolchains/XcodeDefault.xctoolchain/usr/include/c++/v1/cmath
I have followed the #2 option and build is successful now!
And thanks to solodon for the detailed answer. I followed the answer to fix the issue.
Solution 3
You may try to use the CommandLineTools SDK rather than the XCode.app SDK.
I fix this problem when I compiling PointCloudLibrary (PCL)
#Check the current sdk
xcrun --show-sdk-path
#Change sdk
sudo xcode-select -s /Library/Developer/CommandLineTools #Using CommandLineTools SDK
sudo xcode-select -s /Applications/Xcode.app/Contents/Developer #Using XCode.app SDK
Also, reinstall XCode.app and CommandLineTools may help.
Solution 4
I am having the same problem while trying to target iOS (both on my MacBook Air and on GitHub Actions runner) and here are a few more thoughts on the problem even though I'm not familiar enough with Apple's ecosystem to suggest a proper solution. The original command line was coming from CMake in cpprestsdk, but once I boiled it down to essentials, here is a short repro.
- Create file
cmath-bug.cpp
with the only line in it:
#include <cmath>
- Run (newlines in front of some arguments are for reading convenience, remove them)
clang -v -x c++ -target arm64-apple-ios13.2 -fcolor-diagnostics -std=c++11 -stdlib=libc++
-isysroot /Applications/Xcode.app/Contents/Developer/Platforms/iPhoneOS.platform/Developer/SDKs/iPhoneOS13.2.sdk
-isystem /Applications/Xcode.app/Contents/Developer/Platforms/iPhoneOS.platform/Developer/SDKs/iPhoneOS13.2.sdk/usr/include
-c cmath-bug.cpp
When I run it, i get the familiar to many facing the same issue:
Apple clang version 11.0.0 (clang-1100.0.33.16)
Target: arm64-apple-ios13.2
Thread model: posix
InstalledDir: /Applications/Xcode.app/Contents/Developer/Toolchains/XcodeDefault.xctoolchain/usr/bin
"/Applications/Xcode.app/Contents/Developer/Toolchains/XcodeDefault.xctoolchain/usr/bin/clang" -cc1 -triple arm64-apple-ios13.2.0 -Wdeprecated-objc-isa-usage -Werror=deprecated-objc-isa-usage -Werror=implicit-function-declaration -emit-obj -mrelax-all -disable-free -disable-llvm-verifier -discard-value-names -main-file-name cmath-bug.cpp -mrelocation-model pic -pic-level 2 -mthread-model posix -mdisable-fp-elim -fno-strict-return -masm-verbose -munwind-tables -target-sdk-version=13.2 -target-cpu cyclone -target-feature +fp-armv8 -target-feature +neon -target-feature +crypto -target-feature +zcm -target-feature +zcz -target-feature +sha2 -target-feature +aes -target-abi darwinpcs -fallow-half-arguments-and-returns -dwarf-column-info -debugger-tuning=lldb -ggnu-pubnames -target-linker-version 530 -v -coverage-notes-file /Users/myuser/Projects/C++/cmath-bug.gcno -resource-dir /Applications/Xcode.app/Contents/Developer/Toolchains/XcodeDefault.xctoolchain/usr/lib/clang/11.0.0 -isysroot /Applications/Xcode.app/Contents/Developer/Platforms/iPhoneOS.platform/Developer/SDKs/iPhoneOS13.2.sdk -isystem /Applications/Xcode.app/Contents/Developer/Platforms/iPhoneOS.platform/Developer/SDKs/iPhoneOS13.2.sdk/usr/include -stdlib=libc++ -internal-isystem /Applications/Xcode.app/Contents/Developer/Toolchains/XcodeDefault.xctoolchain/usr/bin/../include/c++/v1 -Wno-framework-include-private-from-public -Wno-atimport-in-framework-header -Wno-extra-semi-stmt -Wno-quoted-include-in-framework-header -std=c++11 -fdeprecated-macro -fdebug-compilation-dir /Users/myuser/Projects/C++ -ferror-limit 19 -fmessage-length 204 -stack-protector 1 -fstack-check -mdarwin-stkchk-strong-link -fblocks -fencode-extended-block-signature -fregister-global-dtors-with-atexit -fobjc-runtime=ios-13.2.0 -fcxx-exceptions -fexceptions -fmax-type-align=16 -fdiagnostics-show-option -fcolor-diagnostics -o cmath-bug.o -x c++ cmath-bug.cpp
clang -cc1 version 11.0.0 (clang-1100.0.33.16) default target x86_64-apple-darwin19.0.0
ignoring nonexistent directory "/Applications/Xcode.app/Contents/Developer/Platforms/iPhoneOS.platform/Developer/SDKs/iPhoneOS13.2.sdk/usr/include/c++/v1"
ignoring nonexistent directory "/Applications/Xcode.app/Contents/Developer/Platforms/iPhoneOS.platform/Developer/SDKs/iPhoneOS13.2.sdk/usr/local/include"
ignoring nonexistent directory "/Applications/Xcode.app/Contents/Developer/Platforms/iPhoneOS.platform/Developer/SDKs/iPhoneOS13.2.sdk/Library/Frameworks"
ignoring duplicate directory "/Applications/Xcode.app/Contents/Developer/Platforms/iPhoneOS.platform/Developer/SDKs/iPhoneOS13.2.sdk/usr/include"
#include "..." search starts here:
#include <...> search starts here:
/Applications/Xcode.app/Contents/Developer/Platforms/iPhoneOS.platform/Developer/SDKs/iPhoneOS13.2.sdk/usr/include
/Applications/Xcode.app/Contents/Developer/Toolchains/XcodeDefault.xctoolchain/usr/bin/../include/c++/v1
/Applications/Xcode.app/Contents/Developer/Toolchains/XcodeDefault.xctoolchain/usr/lib/clang/11.0.0/include
/Applications/Xcode.app/Contents/Developer/Toolchains/XcodeDefault.xctoolchain/usr/include
/Applications/Xcode.app/Contents/Developer/Platforms/iPhoneOS.platform/Developer/SDKs/iPhoneOS13.2.sdk/System/Library/Frameworks (framework directory)
End of search list.
In file included from cmath-bug.cpp:1:
/Applications/Xcode.app/Contents/Developer/Toolchains/XcodeDefault.xctoolchain/usr/bin/../include/c++/v1/cmath:318:9: error: no member named 'signbit' in the global namespace
using ::signbit;
~~^
/Applications/Xcode.app/Contents/Developer/Toolchains/XcodeDefault.xctoolchain/usr/bin/../include/c++/v1/cmath:319:9: error: no member named 'fpclassify' in the global namespace
using ::fpclassify;
~~^
/Applications/Xcode.app/Contents/Developer/Toolchains/XcodeDefault.xctoolchain/usr/bin/../include/c++/v1/cmath:320:9: error: no member named 'isfinite' in the global namespace; did you mean 'finite'?
using ::isfinite;
~~^
The only 2 include directories I pass on my original command line exist and are:
$ ls -alF /Applications/Xcode.app/Contents/Developer/Platforms/iPhoneOS.platform/Developer/SDKs/iPhoneOS13.2.sdk
lrwxr-xr-x 1 root wheel 12B Dec 17 11:54 /Applications/Xcode.app/Contents/Developer/Platforms/iPhoneOS.platform/Developer/SDKs/iPhoneOS13.2.sdk@ -> iPhoneOS.sdk
$ ls -alF /Applications/Xcode.app/Contents/Developer/Platforms/iPhoneOS.platform/Developer/SDKs/iPhoneOS13.2.sdk/usr/include
total 2160
drwxr-xr-x 169 root wheel 5.3K Dec 17 12:07 ./
drwxr-xr-x 5 root wheel 160B Nov 4 19:22 ../
...
-rw-r--r-- 9 root wheel 32K Nov 4 19:52 math.h
...
But the interesting bits here are the non-existing include directories it reports as well as the include directories it ends up searching eventually and their order. My guess is that additional directories not mentioned on the command line are inserted by Apple Clang's driver based on some Apple-specific logic.
You can see from the reported error that the <cmath>
header is found at: /Applications/Xcode.app/Contents/Developer/Toolchains/XcodeDefault.xctoolchain/usr/include/c++/v1/cmath
and at line 304 of it you can see:
#include <__config> // Line 304
#include <math.h> // This one ends up causing troubles
#include <__cxx_version>
Judging from the fact that in that same folder /Applications/Xcode.app/Contents/Developer/Toolchains/XcodeDefault.xctoolchain/usr/include/c++/v1/
there is a file math.h
that provides the necessary definitions, e.g.:
#include <__config>
#if !defined(_LIBCPP_HAS_NO_PRAGMA_SYSTEM_HEADER)
#pragma GCC system_header
#endif
#include_next <math.h>
#ifdef __cplusplus
// We support including .h headers inside 'extern "C"' contexts, so switch
// back to C++ linkage before including these C++ headers.
extern "C++" {
#include <type_traits>
#include <limits>
// signbit
#ifdef signbit
template <class _A1>
_LIBCPP_INLINE_VISIBILITY
bool
__libcpp_signbit(_A1 __lcpp_x) _NOEXCEPT
{
return signbit(__lcpp_x);
}
#undef signbit
template <class _A1>
inline _LIBCPP_INLINE_VISIBILITY
typename std::enable_if<std::is_floating_point<_A1>::value, bool>::type
signbit(_A1 __lcpp_x) _NOEXCEPT
{
return __libcpp_signbit((typename std::__promote<_A1>::type)__lcpp_x);
}
...
#elif defined(_LIBCPP_MSVCRT)
...
#endif // signbit
the authors of <cmath>
were expecting the math.h
from that same folder be included first and then the #include_next <math.h>
directive find the system-specific math.h
. That is not what's happening in reality however.
If you look at the first 2 entries in searched directories:
#include <...> search starts here:
/Applications/Xcode.app/Contents/Developer/Platforms/iPhoneOS.platform/Developer/SDKs/iPhoneOS13.2.sdk/usr/include
/Applications/Xcode.app/Contents/Developer/Toolchains/XcodeDefault.xctoolchain/usr/bin/../include/c++/v1
you see that system-specific include directory ends up being above the Clang-injected standard library directory, which is why the system-specific math.h
is being found, not the one in the same folder as the rest of the standard library headers. This is likely the case because if I explicitly add the standard library include directory to my command line BEFORE the other two directories -isystem /Applications/Xcode.app/Contents/Developer/Toolchains/XcodeDefault.xctoolchain/usr/bin/../include/c++/v1
the problem goes away and I am able to compile the file. That's not what Clang's driver or whatever else is involved here does automatically: it adds that standard library directory via -internal-system
(not sure what's the semantics of that internal flag) and it adds it AFTER the system directory.
Now if you look at the list of ignored directories, the very first entry in that list is:
ignoring nonexistent directory "/Applications/Xcode.app/Contents/Developer/Platforms/iPhoneOS.platform/Developer/SDKs/iPhoneOS13.2.sdk/usr/include/c++/v1"
of which the trailing c++/v1
part does not exist on my machine, making me wonder whether the iPhone SDK installation was supposed to create a symbolic link c++
inside the existing part of the path to point to /Applications/Xcode.app/Contents/Developer/Toolchains/XcodeDefault.xctoolchain/usr/include/c++
directory to make the whole thing work.
Anyways, this what I think is happening and I wonder if anyone knows how to properly fix this?
Thank you!
P.S. For the context:
$ xcode-select -p
/Applications/Xcode.app/Contents/Developer
$ xcrun --show-sdk-path -sdk iphoneos13.2
/Applications/Xcode.app/Contents/Developer/Platforms/iPhoneOS.platform/Developer/SDKs/iPhoneOS13.2.sdk
Solution 5
It is possible your copy of Xcode is corrupted. Check with codesign:
codesign --verify /Applications/Xcode.app
This happened to me, and the problem was corrupted Xcode. Reinstalling fixed it.
Something had modified the following:
file added: /Applications/Xcode-11.3.1-.app/Contents/Developer/Library/Frameworks/Python3.framework/Versions/3.7/lib/python3.7/json/__pycache__/scanner.cpython-37.pyc
file added: /Applications/Xcode-11.3.1-.app/Contents/Developer/Library/Frameworks/Python3.framework/Versions/3.7/lib/python3.7/json/__pycache__/decoder.cpython-37.pyc
file added: /Applications/Xcode-11.3.1-.app/Contents/Developer/Library/Frameworks/Python3.framework/Versions/3.7/lib/python3.7/json/__pycache__/encoder.cpython-37.pyc
file added: /Applications/Xcode-11.3.1-.app/Contents/Developer/Library/Frameworks/Python3.framework/Versions/3.7/lib/python3.7/json/__pycache__/__init__.cpython-37.pyc
file modified: /Applications/Xcode-11.3.1-.app/Contents/Developer/Platforms/AppleTVOS.platform/Developer/SDKs/AppleTVOS.sdk/usr/include/math.h
file modified: /Applications/Xcode-11.3.1-.app/Contents/Developer/Platforms/iPhoneOS.platform/Developer/SDKs/iPhoneOS.sdk/usr/include/math.h
file modified: /Applications/Xcode-11.3.1-.app/Contents/Developer/Platforms/WatchOS.platform/Developer/SDKs/WatchOS.sdk/usr/include/math.h
file modified: /Applications/Xcode-11.3.1-.app/Contents/Developer/Platforms/MacOSX.platform/Developer/SDKs/MacOSX.sdk/usr/include/math.h
file modified: /Applications/Xcode-11.3.1-.app/Contents/Developer/Platforms/MacOSX.platform/Developer/SDKs/MacOSX.sdk/System/Library/Frameworks/Kernel.framework/Versions/A/Headers/math.h
file modified: /Applications/Xcode-11.3.1-.app/Contents/Developer/Platforms/MacOSX.platform/Developer/SDKs/DriverKit19.0.sdk/System/DriverKit/usr/include/math.h
file modified: /Applications/Xcode-11.3.1-.app/Contents/Developer/Platforms/WatchSimulator.platform/Developer/SDKs/WatchSimulator.sdk/usr/include/math.h
file modified: /Applications/Xcode-11.3.1-.app/Contents/Developer/Platforms/AppleTVSimulator.platform/Developer/SDKs/AppleTVSimulator.sdk/usr/include/math.h
file modified: /Applications/Xcode-11.3.1-.app/Contents/Developer/Platforms/iPhoneSimulator.platform/Developer/SDKs/iPhoneSimulator.sdk/usr/include/math.h
math.h
was empty in all the above places.
Related videos on Youtube
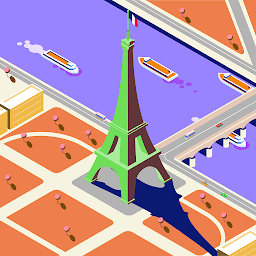
roman Sztergbaum
Updated on May 25, 2021Comments
-
roman Sztergbaum almost 3 years
After upgrading to Catalina from Mojave, Setuping: /Applications/Xcode.app/Contents/Developer/Platforms/MacOSX.platform/Developer/SDKs/MacOSX10.15.sdk in the env.
I'm unable to compile a program that use
<cmath>
header.I tried changing CFLAGS, CCFLAGS, CXXFLAGS to point to the MacOSSDK Location that change nothing
Scanning dependencies of target OgreMain /Applications/Xcode.app/Contents/Developer/usr/bin/make -f OgreMain/CMakeFiles/OgreMain.dir/build.make OgreMain/CMakeFiles/OgreMain.dir/build [ 0%] Building CXX object OgreMain/CMakeFiles/OgreMain.dir/src/OgreASTCCodec.cpp.o cd /Users/roman/Downloads/ogre-1.12.2/build/OgreMain && /Applications/Xcode.app/Contents/Developer/Toolchains/XcodeDefault.xctoolchain/usr/bin/c++ -DOgreMain_EXPORTS -D__ASSERT_MACROS_DEFINE_VERSIONS_WITHOUT_UNDERSCORES=0 -I/Users/roman/Downloads/ogre-1.12.2/OgreMain/src/OSX -I/Users/roman/Downloads/ogre-1.12.2/OgreMain/include/Threading -I/Users/roman/Downloads/ogre-1.12.2/OgreMain/src -I/Users/roman/Downloads/ogre-1.12.2/build/Dependencies/include -I/Users/roman/Downloads/ogre-1.12.2/OgreMain/include -I/Users/roman/Downloads/ogre-1.12.2/build/include -I/Users/roman/Downloads/ogre-1.12.2/OgreMain -isystem /usr/local/include -Wall -Winit-self -Wcast-qual -Wwrite-strings -Wextra -Wundef -Wmissing-declarations -Wno-unused-parameter -Wshadow -Wno-missing-field-initializers -Wno-long-long -Wno-inconsistent-missing-override -msse -O3 -DNDEBUG -arch x86_64 -isysroot /Applications/Xcode.app/Contents/Developer/Platforms/MacOSX.platform/Developer/SDKs/MacOSX10.15.sdk -fPIC -fvisibility=hidden -fvisibility-inlines-hidden -std=c++11 -o CMakeFiles/OgreMain.dir/src/OgreASTCCodec.cpp.o -c /Users/roman/Downloads/ogre-1.12.2/OgreMain/src/OgreASTCCodec.cpp In file included from /Users/roman/Downloads/ogre-1.12.2/OgreMain/src/OgreASTCCodec.cpp:29: In file included from /Users/roman/Downloads/ogre-1.12.2/OgreMain/src/OgreStableHeaders.h:40: In file included from /Users/roman/Downloads/ogre-1.12.2/OgreMain/include/OgrePrerequisites.h:309: In file included from /Users/roman/Downloads/ogre-1.12.2/OgreMain/include/OgreStdHeaders.h:10: /Applications/Xcode.app/Contents/Developer/Toolchains/XcodeDefault.xctoolchain/usr/bin/../include/c++/v1/cmath:314:9: error: no member named 'signbit' in the global namespace using ::signbit; ~~^ /Applications/Xcode.app/Contents/Developer/Toolchains/XcodeDefault.xctoolchain/usr/bin/../include/c++/v1/cmath:315:9: error: no member named 'fpclassify' in the global namespace using ::fpclassify; ~~^ /Applications/Xcode.app/Contents/Developer/Toolchains/XcodeDefault.xctoolchain/usr/bin/../include/c++/v1/cmath:316:9: error: no member named 'isfinite' in the global namespace; did you mean 'finite'? using ::isfinite;
for example the macro:
isless
is present in the global namespace and on my computer:➜ cat math.h | grep "isless" #define isless(x, y) __builtin_isless((x),(y)) #define islessequal(x, y) __builtin_islessequal((x),(y)) #define islessgreater(x, y) __builtin_islessgreater((x),(y)) ➜ pwd /usr/local/include ➜
Even the cmath header include it:
➜ cat /Applications/Xcode.app/Contents/Developer/Toolchains/XcodeDefault.xctoolchain/usr/bin/../include/c++/v1/cmath | grep "math.h" #include <math.h>
And my command line have the option
-isystem /usr/local/include
This should work...
-
Eljay over 4 yearsDoes
xcode-select -p
match where Xcode is located? Can you change the code tousing std::signbit;
, likewise for the others? Are you compiling as C++11 or later? -
roman Sztergbaum over 4 yearsCompiling as C++ 11. I cannot change the code, it's an external dependencies ! yes
xcode-select -p
match whereXCode
is located. -
Eljay over 4 yearsThat's not good. The code is trying to do
using ::signbit;
and the symbol isn't in the global namespace, it is instd::
namespace. I presume likewise with the others (I didn't chase them down).
-
-
mkl about 4 years
set(CMAKE_OSX_SYSROOT ...)
goes intoCMakeLists.txt
, not the shell. -
kkaefer about 4 yearsI ran into this issue as well. It turns out that some
pkg-config
files (e.g. libcurl) from Homebrew add this path automatically, even if you have Xcode installed. This was fixed in Homebrew 2.2.13. More details in github.com/Homebrew/brew/issues/5068; PR that fixes this is in github.com/Homebrew/brew/pull/7331. TL;DR: Update homebrew -
pahjbo about 4 yearsYes it was an old homebrew pkg_config for zlib that was still somehow lying around, that was causing this for me - despite having an up to date homebrew and not currently having a zlib installed anyway
-
Tiago Martins Peres almost 4 yearsHi Niloy Datta! I suggest you to edit this answer removing the question from this answer and include only what answers. If you have a question, then ask separately in the proper way questions should be asked in this community.
-
Niloy Datta almost 4 years@TiagoMartinsPeres李大仁, removed it. Thanks.
-
Hector Esteban almost 4 yearsThanks man, it worked for me. Do you know a cleaner way to deal with this problem though?
-
Niloy Datta almost 4 years@VictorSanchez, still trying to figure it out. Will notify once I get it.
-
Hector Esteban almost 4 yearsThe problem is that when using PCL I have to do the change but other times I just import cmath I have to reverse it
-
jbm almost 4 yearsAre there any recommendations on how to fix this? I tried uninstalling both the command line tools and Xcode (11.4.1), but the
#include<...> search
order is still wrong. -
Ryan H. almost 4 yearsThe only thing I can think of is to look in your login scripts such as
~/.bash_profile
,~/.zshrc
etc. for things that can affect the search order such as compiler environment variables (in my case it wasCPLUS_INCLUDE_PATH
) or the order of directories in yourPATH
. -
hunzter almost 4 yearsThank you for your details explaination. But sadly no solution seems to work for my machine. I am about to goes for the final option to reinstall Catalina. Damn Apple, why they make things so difficult?
-
JerryN almost 4 yearsI followed these directions, from above, to hard code a path to math.h. That worked fine for a while but I suspect that a newer version of Xcode broke this and I was getting compilation errors involving math.h. So don't do this
Using #include</Applications/Xcode.app/Contents/Developer/Toolchains/XcodeDefault.xctoolchain/usr/include/c++/v1/math.h> instead of <math.h> in /Applications/Xcode.app/Contents/Developer/Toolchains/XcodeDefault.xctoolchain/usr/include/c++/v1/cmath
!!! -
JerryN almost 4 yearssee my comment above about how hard coding a path to math.h is a bad idea.
-
Jake Cobb almost 4 yearsCause is same as @solodon mentioned. Got this with MacOSX SDK as described here, picked up the SDK
usr/include
include due to CMakefind_package
getting e.g. zlib from it. Fix was to put the toolchainusr/include/c++/v1
folder onto the system includes (-isystem
to clang, orinclude_directories(SYSTEM ...)
in CMake). I deduced the toolchain directory off the CMAKE_LINKER path rather than hard-code it. -
burlyearly over 3 yearsInstead of 2, consider changing <cmath> to do
#include "./math.h"
. This avoids having to put the fully-qualified filename in there and still works (for me, at least). -
Vlad over 3 yearsOn my side the problem was fixed by removing directory
/Library/Developer/CommandLineTools
. I was building Swift for Android on macOS Catalina. -
Cris Luengo over 3 yearsNo matter how I changed the include path order, I couldn't get <cmath> to load the right <math.h>.
#include "./math.h"
didn't work either. Only including the full path solved the issue for me. I hate that, it's going to give me headaches later on, but it's the only thing that worked for me. libclang must be doing something weird with the search path. -
WeakPointer over 3 yearsThis answer was very helpful for me too. In my case, I had had such an explicit include in my Go CGO_CPPFLAGS environment variable, left over from a project that hasn't been updated to know where the Darwin stdio.h file could be found but llvm wasn't being used explicitly in that one, and I carried that over to a project that was already up to date with building from the latest Darwin but now, needing to have the LLVM c++/v1 files consistent with themselves, that explicit help of where to find Darwin headers was a problem. This was a huge help.
-
PacificSky over 3 yearsThis IS the fix. Other suggestions like CMAKE_OSX_SYSROOT or setting CPATH did not help.
-
zkoza over 3 yearsYour method is easy, but dangerous, because it breaks the compiler system (OP may need it for other purposes than Catalina, and will certainly very soon forget about system changes he made). Moreover, it may work only until the next system upgrade. I would not touch the files that come with a compiler, and
/usr/local/include/math.h
is one of such files. -
chunkyguy about 3 yearsI had a similar issue due to a file called Math.h in
llvm/include/llvm/CodeGen/PBQP/Math.h
which gets indirectly referenced from cstdlib. -
Some Guy on the Internet over 2 yearsI'm using Cmake. It worked for me to add
set(CMAKE_OSX_SYSROOT /Applications/Xcode.app/Contents/Developer/Platforms/MacOSX.platform/Developer/SDKs/MacOSX10.14.sdk)
-
Michael Roswell about 2 yearsI recently reinstalled command line tools, macos 12.2, ran
brew upgrade
and still had to do this! Option 2 above totally worked for me today, but why hasn't this been fixed upstream of me? -
Michael Roswell about 2 yearsPerhaps useful to someone: to edit this file I ran
sudo vi /Library/Developer/CommandLineTools/SDKs/MacOSX.sdk/usr/include/c++/v1/cmath1
, added the new#include
line, and closed withesc
followed by:x!