change UITextField accessibility description
Solution 1
My advice would be to not try to outsmart the system on build-in Voice Over outputs.
"Text field is editing" is to a blind user the audible equivalent to "This item is selected and there is a blinking cursor in it".
Same as you are unlikely to remove/change the blinking cursor from your UITetxtField you shouldn't try to remove/change that built in output.
Feel free to use the accessibilityLabel
and accessibilityHint
to add more context to this field.
Solution 2
How to Quiet "Text Field" & Options to Replace It
I posted a related answer that illustrates a few ways to stop VoiceOver from announcing "text field" for a UITextField.
This is the a code excerpt from that answer.
Quieting "Text Field"
let textField = UITextField()
textField.accessibilityTraits = UIAccessibilityTraitStaticText
Replacement Text
You could use the accessibilityHint for the alternate control name you want "code verification field"
textField.accessibilityHint = "code verification field"
accessibilityLabel
would also work. The practical difference is that accessibilityLabel
is read first, then textField.text
(the text entered in the UITextField), then the accessibilityHint
.
The following is read as: "label, text, (short pause...) hint"
textField.accessibilityTraits = UIAccessibilityTraitStaticText
textField.accessibilityLabel = "label"
textField.text = "text"
textField.accessibilityHint = "hint"
You can find out more about UIAccessibility Element properties in Apple's API reference.
Solution 3
I believe you have to set the field's accessibilityLabel
property (part of the UIAccessibility
protocol). Perhaps you also have to play around with the accessibilityTraits
property to override the UIKit labeling.
Solution 4
As per my knowledge, Voice over reads the accessibilityLabel, accessibilityHint, "text field is editing" when it is a textfield. There is no way to change this until you change the trait.
YOU can change the trait to UIAccessibilityTraitNone
, so that it does not read as "textfield is editing".
Solution 5
Updating this question with some current advice.
It is not appropriate to override UIKit's default accessibility handling beyond the accessibility properties it provides. Use accessibilityLabel, accessibilityHint, accessibilityTraits, etc.
UITextField has built in accessibility and handles role and state information to provide a consistent and robust experience to VoiceOver users. You can suppress certain VoiceOver announcements using accessibilityTraits, but that does not mean that you should.
The most appropriate thing to do in such a situation is to simply set a suitable label: myTextField.accessibilityLabel = "Code verification"
This will result in VoiceOver announcing, "Code verification, text field, is editing", which is what users will expect to hear.
Note that accessibilityHint can be turned off by users, so this property should not be used for essential messaging or information.
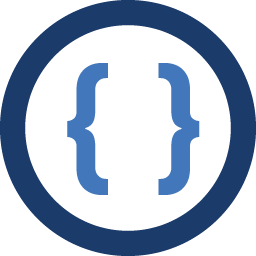
Admin
Updated on June 25, 2022Comments
-
Admin almost 2 years
Is there a way to set the accessibility label of UITextField to be something other than the "text field". So instead of calling "text field", I want to name it "code verification field".
-
Admin over 12 yearsThank you, Ole Begemann! I tried to change accessibilityLabel and accessibilityTraits. So if I set both of them I have voice over pronouncing: <accessibilityLabel>, <accessibilityHint> "text field is editing". But I want voice over instead of "text field" to say "code verification field". what can I do with accessibilityTraits?