Change/update Firebase notification token or instance id forcefully via code?
Solution 1
Now i got my answer after facing many troubles for generating new or change token of firebase for push notification.
1) Delete old Firebase token
let instance = FIRInstanceID.instanceID()
_ = FIRInstanceID.delete(instance)
FIRInstanceID.instanceID().delete { (err:Error?) in
if err != nil{
print(err.debugDescription);
} else {
print("Token Deleted");
}
}
2) Request new Firebase token
if let token = FIRInstanceID.instanceID().token() {
print("Token \(token) fetched");
} else {
print("Unable to fetch token");
}
FIRMessaging.messaging().connect { (error) in
if (error != nil) {
print("Error connecting to FCM. \(error.debugDescription)")
} else {
print("Connected to FCM.")
}
}
UPDATE FOR SWIFT 4 & Firebase 4.8.2 (Follow simple two steps)ππ
1) Delete old Token
let instance = InstanceID.instanceID()
instance.deleteID { (error) in
print(error.debugDescription)
}
2) Request for new token
if let token = InstanceID.instanceID().token() {
print("Token : \(token)");
} else {
print(βError: unable to fetch token");
}
Messaging.messaging().shouldEstablishDirectChannel = true
You can get updated token in MessagingDelegate method didReceiveRegistrationToken
and in Refresh Token.
func messaging(_ messaging: Messaging, didReceiveRegistrationToken fcmToken: String) {
print("Firebase Token : \(fcmToken)")
}
Solution 2
UPDATED FOR FIREBASE MESSAGING 7.3.0
class func regenerateFCM(){
Installations.installations().delete { (err) in
if let err = err {
print(err)
}else{
Installations.installations().authTokenForcingRefresh(true) { (result,err) in
if let result = result {
print(result)
Messaging.messaging().deleteToken { (err) in
if let err = err {
print(err)
}else{
print("FCM TOKEN DELETED")
Messaging.messaging().token { (token, err) in
if let token = token {
print("NEW FCM TOKEN GENERATED")
print(token)
}
if let err = err {
print("ERROR WHILE GENERATING NEW FCM TOKEN")
print(err)
}
}
}
}
}else if let err = err {
print(err)
}
}
}
}
}
UPDATE FOR FIREBASE 8.5.0
Messaging.messaging().deleteToken { err in
if let err = err {
print("Error while generating new FCM Token")
print(err)
}else{
Messaging.messaging().token { token, err in
if let token = token {
print("NEW FCM TOKEN GENERATED")
print(token)
}
}
}
}
Solution 3
for now InstanceID.instanceID().token()
is deprecated.
You should use this:
let instance = InstanceID.instanceID()
instance.deleteID { (error) in
print(error.debugDescription)
}
instance.instanceID { (result, error) in
if let error = error {
print("Error fetching remote instange ID: \(error)")
} else {
print("Remote instance ID token: \(String(describing: result?.token))")
}
}
Messaging.messaging().shouldEstablishDirectChannel = true
Then in AppDelegate:
extension AppDelegate: MessagingDelegate {
func messaging(_ messaging: Messaging, didReceiveRegistrationToken fcmToken: String) {
//Here is your new FCM token
print("Registered with FCM with token:", fcmToken)
}
Solution 4
Updated Answer for Swift 4, FireBase 4.8.2, FirebaseMessaging (2.0.8)
debugPrint("Existing Token :- \(Messaging.messaging().fcmToken!)")
let instance = InstanceID.instanceID()
instance.deleteID { (error) in
print(error.debugDescription)
}
if let token = InstanceID.instanceID().token() {
print("Token \(token) fetched");
} else {
print("Unable to fetch token");
}
Messaging.messaging().shouldEstablishDirectChannel = true
We receive this updated token in MessagingDelegate method as well as in Refresh Token
func messaging(_ messaging: Messaging, didReceiveRegistrationToken fcmToken: String) {
print("Firebase registration token: \(fcmToken)")
}
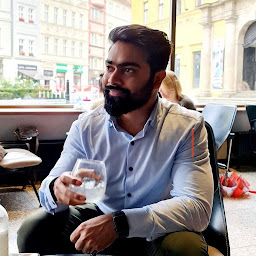
PRAVEEN
β Excellence in Swift programming with professional experience in developing iOS applications. β Proficient in Implementing Security and Data protection measures. β Developed Custom Framework and Distribute it through cocoapods. β Extensive knowledge of working with XCode, UIKit. β Proficient in using local storage including SQLite. β Experience in Social API integration, RestFul Web APIs integration, Web Views, APNS. β Integrate with backend services (JSON, REST, etc.) to delivering a great mobile user the experience that is super-fast for end users. β Writing good quality code. β Applying best practices, and software architecture guidelines. β Strong knowledge of Object Oriented Programming, design patterns and app architectures (MVC) β Experience with Source Control. eg. git
Updated on June 17, 2022Comments
-
PRAVEEN almost 2 years
What should I do that for changing or requesting the token in firebase? the unique token generated by firebase on the basis of device information.
-
Daniel Arantes Loverde over 6 yearsThis "messaging().connect" is needed for renewed token ?
-
PRAVEEN over 6 yearsyes because there is no way to renew the token, first we have to delete the token and after that requesting for a new token, So "messaging().connect" required. if you will find another way, suggestions are always welcome.
-
Daniel Arantes Loverde over 6 yearsThis "delete" is not used anymore, we have to use "shouldEstablishDirectChannel" boolean, and it is not refreshing the token, keep the same. When i resolve it, i will put here. Thanks!
-
Szekspir over 6 yearsfor Swift 3: let instance = InstanceID.instanceID() instance.deleteID { (error) in print(error.debugDescription) }
-
Mike Flynn over 5 yearsHow can this be done with regular objective C and the older firebase SDK?
-
Nikunj Jadav about 4 yearsIts working at first time only, then not receive notification from firebase console and apps. Permission is allowed and token is refreshed and tried with refresh token. Any idea for push notification are not coming?
-
Harsh Pipaliya over 3 yearsin "instance.instanceID" result?.token return old token. therefore no need to call this method.
-
Tal Zion almost 3 yearsDid you get it to work? @Shahzaib I got new tokens but they don't work with FCM. Only the initial token works