Changing Images continously with button click Android
You do not want to set multiple onClickListeners for each of the different Images you have. You would just need to keep track of the current image so that when you click the button it knows which is the next image.
This code should give you and example of what I mean.
import android.app.Activity;
import android.os.Bundle;
import android.view.View;
import android.view.View.OnClickListener;
import android.widget.Button;
import android.widget.ImageView;
public class Main extends Activity {
private ImageView hImageViewSemafor;
private Button hButton;
private int currentImage = 0;
private int numImages = 10;
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
hImageViewSemafor = (ImageView)findViewById(R.id.idImageViewSemafor);
hButton = (Button) findViewById(R.id.idBtnChangeImage);
//Just set one Click listener for the image
hButton.setOnClickListener(aButtonChangeImageListener);
}
View.OnClickListener aButtonChangeImageListener = new OnClickListener() {
public void onClick(View v) {
//Increase Counter to move to next Image
currentImage++;
currentImage = currentImage % numImages
//Set the image depending on the counter.
switch (currentImage) {
case 0: hImageViewSemafor.setImageResource(R.drawable.r1);
break;
case 1: hImageViewSemafor.setImageResource(R.drawable.r2);
break;
case 2: hImageViewSemafor.setImageResource(R.drawable.r3);
break;
case 3: hImageViewSemafor.setImageResource(R.drawable.r4);
break;
case 4: hImageViewSemafor.setImageResource(R.drawable.r5);
break;
case 5: hImageViewSemafor.setImageResource(R.drawable.r6);
break;
case 6: hImageViewSemafor.setImageResource(R.drawable.r7);
break;
case 7: hImageViewSemafor.setImageResource(R.drawable.r8);
break;
case 8: hImageViewSemafor.setImageResource(R.drawable.r9);
break;
case 9: hImageViewSemafor.setImageResource(R.drawable.r10);
break;
default: hImageViewSemafor.setImageResource(R.drawable.r1);
}
}
};
}
Hopefully this will work, but I have not tested it.
UPDATE
Removed the big switch block.
import android.app.Activity;
import android.os.Bundle;
import android.view.View;
import android.view.View.OnClickListener;
import android.widget.Button;
import android.widget.ImageView;
public class Main extends Activity {
private ImageView hImageViewSemafor;
private Button hButton;
private int currentImage = 0;
int[] images = { R.drawable.r1, R.drawable.r2, R.drawable.r3, R.drawable.r4, R.drawable.r5, R.drawable.r6, R.drawable.r7, R.drawable.r8, R.drawable.r9, R.drawable.r10 };
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
hImageViewSemafor = (ImageView)findViewById(R.id.idImageViewSemafor);
hButton = (Button) findViewById(R.id.idBtnChangeImage);
//Just set one Click listener for the image
hButton.setOnClickListener(aButtonChangeImageListener);
}
View.OnClickListener aButtonChangeImageListener = new OnClickListener() {
public void onClick(View v) {
//Increase Counter to move to next Image
currentImage++;
currentImage = currentImage % images.length;
hImageViewSemafor.setImageResource(images[currentImage]);
}
};
}
UPDATE 2
The problem is in
currentImage--;
currentImage = currentImage % images.length;
as, when going the previous image from the first image, currentImage becomes less than 0. And it is not possible to MOD (%) a negative number. This will be causing your error. So by changing it to this.
currentImage--;
currentImage = (currentImage + images.length) % images.length;
We have fixed the problem of it becoming less than 0 by adding the total amount of images to the currentImage number.
This code below should be the fixed code.
package com.galerionsekiz;
import android.app.Activity;
import android.os.Bundle;
import android.view.View;
import android.view.View.OnClickListener;
import android.widget.Button;
import android.widget.ImageView;
public class Main extends Activity {
private ImageView hImageViewPic;
private Button iButton, gButton;
private int currentImage = 0;
int[] images = { R.drawable.r1, R.drawable.r2, R.drawable.r3, R.drawable.r4, R.drawable.r5, R.drawable.r6, R.drawable.r7, R.drawable.r8, R.drawable.r9, R.drawable.r10 };
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
hImageViewPic = (ImageView)findViewById(R.id.idImageViewPic);
iButton = (Button) findViewById(R.id.bIleri);
gButton = (Button) findViewById(R.id.bGeri);
//Just set one Click listener for the image
iButton.setOnClickListener(iButtonChangeImageListener);
gButton.setOnClickListener(gButtonChangeImageListener);
}
View.OnClickListener iButtonChangeImageListener = new OnClickListener() {
public void onClick(View v) {
//Increase Counter to move to next Image
currentImage++;
currentImage = currentImage % images.length;
hImageViewPic.setImageResource(images[currentImage]);
}
};
View.OnClickListener gButtonChangeImageListener = new OnClickListener() {
public void onClick(View v) {
//Increase Counter to move to next Image
currentImage--;
currentImage = (currentImage + images.length) % images.length;
hImageViewPic.setImageResource(images[currentImage]);
}
};
}
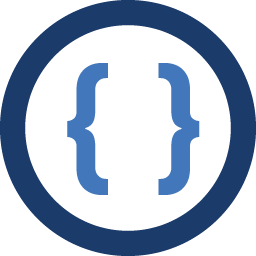
Admin
Updated on August 06, 2020Comments
-
Admin almost 4 years
I hereby thanks to you all who helps me out.
I am trying to make an app which has 10 pictures in it, only one imageview on the main layout and one button.
when i pressed the button, image changes to next one. when i press the button again, it changes to other one.
I couldnt do it for some days. I am trying this;
Main.java;
package com.example.denemeemre; import android.app.Activity; import android.os.Bundle; import android.view.View; import android.view.View.OnClickListener; import android.widget.Button; import android.widget.ImageView; public class Main extends Activity { private ImageView hImageViewSemafor; public Button hButton; public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.main); hImageViewSemafor = (ImageView)findViewById(R.id.idImageViewSemafor); hButton = (Button) findViewById(R.id.idBtnChangeImage); hButton.setOnClickListener(aButtonChangeImageListener); } View.OnClickListener aButtonChangeImageListener = new OnClickListener() { public void onClick(View v) { // setImageResource will change image in ImageView hImageViewSemafor.setImageResource(R.drawable.r1); } }; public void onCreate1(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.main); hImageViewSemafor = (ImageView)findViewById(R.id.idImageViewSemafor); hButton = (Button) findViewById(R.id.idBtnChangeImage); hButton.setOnClickListener(bButtonChangeImageListener); } View.OnClickListener bButtonChangeImageListener = new OnClickListener() { public void onClick(View v) { // setImageResource will change image in ImageView hImageViewSemafor.setImageResource(R.drawable.r2); } }; View.OnClickListener cButtonChangeImageListener = new OnClickListener() { public void onClick(View v) { // setImageResource will change image in ImageView hImageViewSemafor.setImageResource(R.drawable.r3); } }; View.OnClickListener dButtonChangeImageListener = new OnClickListener() { public void onClick(View v) { // setImageResource will change image in ImageView hImageViewSemafor.setImageResource(R.drawable.r4); } }; View.OnClickListener eButtonChangeImageListener = new OnClickListener() { public void onClick(View v) { // setImageResource will change image in ImageView hImageViewSemafor.setImageResource(R.drawable.r5); } }; }
and main.xml;
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="fill_parent" android:layout_height="fill_parent" android:orientation="vertical" > <ImageView android:id="@+id/idImageViewSemafor" android:layout_width="match_parent" android:layout_height="342dp" android:layout_weight="1.04" android:adjustViewBounds="true" android:background="#66FFFFFF" android:maxHeight="91dip" android:maxWidth="47dip" android:padding="10dip" android:src="@drawable/r0" /> <Button android:id="@+id/idBtnChangeImage" style="?android:attr/buttonStyleSmall" android:layout_width="match_parent" android:layout_height="wrap_content" android:text="Sonraki" /> </LinearLayout>
UPDATE;
Now its like this, crashing by going backwards from the first picture;
the code;
package com.galerionsekiz; import android.app.Activity; import android.os.Bundle; import android.view.View; import android.view.View.OnClickListener; import android.widget.Button; import android.widget.ImageView; public class Main extends Activity { private ImageView hImageViewPic; private Button iButton, gButton; private int currentImage = 0; int[] images = { R.drawable.r1, R.drawable.r2, R.drawable.r3, R.drawable.r4, R.drawable.r5, R.drawable.r6, R.drawable.r7, R.drawable.r8, R.drawable.r9, R.drawable.r10 }; public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.main); hImageViewPic = (ImageView)findViewById(R.id.idImageViewPic); iButton = (Button) findViewById(R.id.bIleri); gButton = (Button) findViewById(R.id.bGeri); //Just set one Click listener for the image iButton.setOnClickListener(iButtonChangeImageListener); gButton.setOnClickListener(gButtonChangeImageListener); } View.OnClickListener iButtonChangeImageListener = new OnClickListener() { public void onClick(View v) { //Increase Counter to move to next Image currentImage++; currentImage = currentImage % images.length; hImageViewPic.setImageResource(images[currentImage]); } }; View.OnClickListener gButtonChangeImageListener = new OnClickListener() { public void onClick(View v) { //Increase Counter to move to next Image currentImage--; currentImage = currentImage % images.length; hImageViewPic.setImageResource(images[currentImage]); } }; }
the main;
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="fill_parent" android:layout_height="fill_parent" android:gravity="fill" android:orientation="vertical" android:weightSum="100" > <ImageView android:id="@+id/idImageViewPic" android:layout_width="match_parent" android:layout_height="0dp" android:layout_weight="100" android:adjustViewBounds="true" android:background="#66FFFFFF" android:maxHeight="91dip" android:maxWidth="47dip" android:padding="10dip" android:src="@drawable/r0" /> <LinearLayout android:id="@+id/linearLayout1" android:layout_width="fill_parent" android:layout_height="wrap_content" > <Button android:id="@+id/bGeri" android:layout_width="0dp" android:layout_height="wrap_content" android:layout_weight="1" android:text="Önceki" > </Button> <Button android:id="@+id/bIleri" android:layout_width="0dp" android:layout_height="wrap_content" android:layout_weight="1" android:text="Sonraki" > </Button> </LinearLayout> </LinearLayout>
any suggestions?
-
Seraphim's about 11 yearsyes please paste the code for us.
-
Admin about 11 yearsThanks Mocialov Boris :)
-
Admin about 11 yearsit only changes to the 2nd pic, and stop there :( if i could manage how to do it, i might add a previous and next button.
-
Admin about 11 yearsI did it, it now works well, except one crash issue. I have added a back button, and assing the code. But it crashes when going backwards from the first pic. It is ok for next pic to start over the first picture but the back button doesnt :(
-
-
Pawel Cala about 11 yearsWhy not keep references in int[] and remove huge switch block ? In my opinion it would be a lot cleaner than 10 element switch...
int[] images = { R.drawable.r1, R.drawable.r2.... }; imageview.setImageResource( images[i] );
Hope it helps ;) -
James Grant about 11 years@Pawel Cala I have now removed the big switch block and added and update. I only wanted to do it that way to keep it simple and easy to understand.
-
Admin about 11 yearsGreat, now its better. I have added a back button, and now im trying to solve the counter minus problem, let us see if i can do it :)
-
Admin about 11 yearsI did it, it now works well, except one crash issue. I have added a back button, and assing the code. But it crashes when going backwards from the first pic. It is ok for next pic to start over the first picture but the back button doesnt :(
-
James Grant about 11 years@eistanbullu I have now added a 2nd update with a fix for your previous button. This should now work as intended :)
-
Admin about 11 years@JamesG thank you very much! And this is a general question maybe, but, is there any easy way to make this app run in Java phones? In eclipse, export -> JAR file?
-
James Grant about 11 yearsIf you plug an android phone into your computer you should be able to run it directly on the phone from eclipse. There is some information about this here - developer.android.com/tools/building/building-eclipse.html
-
James Grant about 11 yearsAlso if you my answer has sufficiently answered you problem could you mark it as the best/correct answer :) Thanks
-
James Grant about 11 yearsTo accept an answer you need to click the little arrow on the left next to the top of My answer. This will then show anyone else who is trying to find out the same thing as you that this answer works :) Hope you app is going well
-
TobiMcNamobi over 9 yearsPlease elaborate a bit. Why is this a solution to the OP's question? What are key lines in your code?