Check if a binary number has a '0' or a '1' at a specific position
Solution 1
This will filter out the bit you're looking for:
number & (1 << position)
If you really need a 1 or 0 response, you can use this to make it a boolean value:
!!(number & (1 << position))
Or even better (thanks Vadim K.):
(number >> position) & 1
Solution 2
This expression evaluates to the value of the bit you're looking for, either 0
or 1
:
(number >> position) & 1
Solution 3
Warning: This code is not Standards-compliant. Bjarne Stroustrup says, and he oughta' know, that, "Obviously, it's illegal to write one member and then read another..." Nevertheless I leave this code up for educational purposes...
Here's an alternative that is useful when, say, reading a proprietary binary protocol off of a socket:
#include <cstdlib>
union Value
{
struct
{
unsigned char a_ : 1;
unsigned char b_ : 1;
unsigned char c_ : 1;
unsigned char d_ : 1;
unsigned char e_ : 1;
unsigned char f_ : 1;
unsigned char g_ : 1;
unsigned char h_ : 1;
} bits_;
unsigned char charVal_;
};
int main()
{
unsigned char someValue = static_cast<unsigned char>(0x42);
Value val;
val.charVal_ = someValue;
bool isBitDSet = val.bits_.d_;
return 0;
}
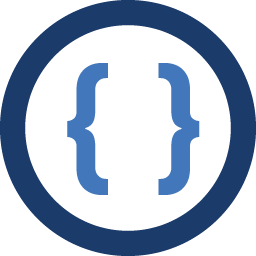
Admin
Updated on June 11, 2022Comments
-
Admin about 2 years
I'd like to check if a binary number has a '0' or a '1' at a specific position.
example:
if the binary number is: 101000100
- checking at position zero (that is at the rightmost '0') should result in '0'.
- checking at position 2 should result in '1'.
- checking at position 3 should result in '0'.
checking at position 6 should result in '1'.
etc...
I'm coding in C, so obviously I could use sprintf / scanf and the likes, but I guess there must be something better (read: more time efficient / easier)!
What would be a good mechanism to do this?