Check if documents exists in Firebase and return according icon
Depending on your usecase you can either use a FutureBuilder, or you keep it simple and create a variable to deal with the logic:
Inside your Widget:
bool isLiked = false;
Call your function from within initState():
@override
void initState() {
super.initState();
checkIfLikedOrNot();
}
Change your logic to modify your variable and tell Flutter to rerender:
checkIfLikedOrNot() async{
DocumentSnapshot ds = await reference.collection("likes").document(currentUser.uid).get();
this.setState(() {
isLiked = ds.exists;
});
}
Draw the corresponding Icon:
Icon(isLiked?Icons.favorite:FontAwesomeIcons.heart,
color: isLiked?Colors.red:null)
Edit: Since you are apparently dealing with a list of values, it makes sense to encapsulate them in an Object or use the FutureBuilder.
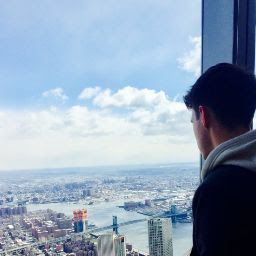
Martin Seubert
Updated on December 11, 2022Comments
-
Martin Seubert over 1 year
I want to check if a specific document exists in a Firebase collection. According to that, my app should display a colored icon or a grey one. I'm trying to tackle the problem with a method, which returns a boolean. In my Build Widget, I call that method and assign the right icon.
Here is my method which checks if the document exists:
checkIfLikedOrNot(reference) async{ DocumentSnapshot ds = await reference.collection("likes").document(currentUser.uid).get(); print(ds.exists); return ds.exists; }
The print shows the right values in the console, but my build widget seems to ignore the fact, that the boolean is true and always returns the icon which should be displayed if there is no document in the collection.
Here is the part of my Build Widget:
GestureDetector( child: checkIfLikedOrNot(list[index].reference) == true ? Icon( Icons.favorite, color: Colors.red, ) : Icon( FontAwesomeIcons.heart, color: null, ), )
Where is the problem with this statement? Any ideas?