Connecting to MongoDB using jdbc driver
Solution 1
You can checkout this project:
https://github.com/erh/mongo-jdbc
There are two examples given.
But in general I would recommend to use the MongoDB Client or some Spring Data abstraction.
Solution 2
If you are getting a ClassNotFoundException, the issue is that the jar containing the mongodb.jdbc.MongoDriver
class is not on your classpath. If you're not sure what JAR this class is in, I would reccomend getting 7-Zip so that you can inspect the contents of the jar and see for yourself if the class is there.
The correct way to connect to MongoDB with your approach is:
Class.forName("mongodb.jdbc.MongoDriver");
String URL = "jdbc:mongo://<servername>:<port>/<databaseName>";
Connection jdbcConn = DriverManager.getConnection(url,"user","pass");
But MongoDB isn't really meant to be used with JDBC, so if your requirements allow, I would reccomend getting a connection the "mongodb" way.
MongoClient client = new MongoClient("localhost");
For details on how to do it this way, see the MongoDB docs
Solution 3
I know its very late to answer but might help someone else. If you are compiling and running your code from cmd then before compilation set classpath for mongo.jar like below :
set classpath=C:\DemoProject\java db\Mongo\mongo.jar;
then run your code.
or if you are using editor like eclipse then add this jar to your lib folder.
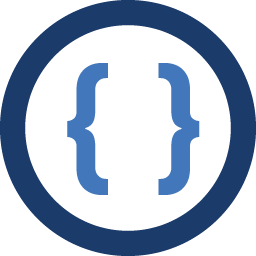
Admin
Updated on December 15, 2020Comments
-
Admin over 3 years
Purpose is to connect MongoDB remote server through JAVA:
URL = "jdbc:mongo://" + serverIP + ":" + port+ "/" +databaseName; Class.forName("mongodb.jdbc.MongoDriver"); dbConn = getConnection(URL,mongo1, mongo1);
Tried Unity_trial.Jar, mongo_version.jar files but the error comes is 'mongodb.jdbc.MongoDriver' classNameNotFound.
If I comment the class.forname line, the next error is
URL = "jdbc:mongo://" + serverIP + ":" + port + "/" +databaseName;
is not in correct format. Not sure about where I am making the mistake. Thanks for your help in advance.