Convert color video to grayscale video in MATLAB
Solution 1
Your first problem is due to trying to assign the 2D output of rgb2gray into a 3D array. You can fix this by converting the gray image back to RGB format:
frames(:,:,:,i)=repmat(rgb2gray(frames(:,:,:,i)),[1 1 3]);
Solution 2
%% convert a RGB video to a grayscale one.
videoFReader = vision.VideoFileReader('xylophone.mpg');
videoFWriter = vision.VideoFileWriter('xylophone_gray.avi',...
'FrameRate',videoFReader.info.VideoFrameRate);
while ~isDone(videoFReader)
videoFrame = step(videoFReader);
step(videoFWriter, rgb2gray(videoFrame));
end
release(videoFReader);
release(videoFWriter);
Solution 3
Try it this way. This should do the trick. The code is self-explanatory.
vid = VideoReader('xylophone.mpg');
numImgs = get(vid, 'NumberOfFrames');
frames = read(vid);
obj=VideoWriter('somefile.avi');
open(obj);
for i=1:numImgs
movie(i).cdata=rgb2gray(frames(:,:,:,i));
movie(i).colormap=gray;
end
writeVideo(obj,movie);
close(obj);
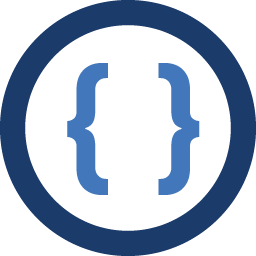
Admin
Updated on June 28, 2022Comments
-
Admin almost 2 years
I am trying to do some operations on color video in MATLAB however, I am facing 2 problems:
I am getting an error while converting color video to grayscale video. I mean I need to convert color video to grayscale video and write it back to .avi file
How can I perform some operation (say edge detection) on grayscale frames (extracted from color video) and then can write back the result of edge detection in .avi video format?
My incomplete code (which consist of color format conversion) is as follows:
vid = VideoReader('Big_buck_bunny_480p_Cut.avi'); numImgs = get(vid, 'NumberOfFrames'); frames = read(vid); for i=1:numImgs frames(:,:,:,i)=rgb2gray(frames(:,:,:,i)); end
Any pointer to fix these two problems?