Convert QByteArray to std::vector<unsigned char>
Solution 1
I'm not familiar with Qt, but surely you just want
std::vector<unsigned char> bufferToCompress(
byteArrayBuffer.begin(), byteArrayBuffer.end());
Note: strlen
is not particularly useful in C++; it tells you the length of a C-style null-terminated string (by searching memory until it either finds either finds a zero-valued byte, or falls off the end of accessible memory and crashes), but can't tell you the size of an array, which is what you'd need here. Also, using evil C-style casts to force invalid code to compile is never a good idea.
Solution 2
As I see here http://qt-project.org/doc/qt-4.8/qbytearray.html QByteArray does not have begin/end methods. But have data/length. Result code may be looks like this:
const unsigned char* begin = reinterpret_cast<unsigned char*>(byteArrayBuffer.data());
const unsigned char* end = begin + byteArrayBuffer.length();
std::vector<unsigned char> bufferToCompress( begin, end );
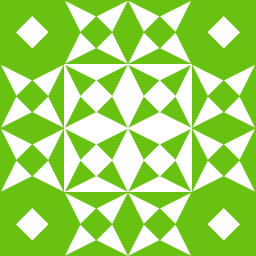
Jacob Krieg
Updated on July 18, 2022Comments
-
Jacob Krieg 5 months
I tried to convert
QByteArray
tostd::vector<unsigned char>
using this code:unsigned char* buffer = (unsigned char*)byteArrayBuffer.constData(); std::vector<unsigned char>::size_type size = strlen((const char*)buffer); std::vector<unsigned char> bufferToCompress(buffer, buffer + size);
but, assuming that
byteArrayBuffer
is aQByteArray
filled with data, I think it doesn't work well on lineunsigned char* buffer = (unsigned char*)byteArrayBuffer.constData();
becausebyteArrayBuffer.size()
returns a different value thanbufferToCompress.size()
.How can I get it working?