Convert uppercase and lowercase in JavaScript
22,938
Solution 1
You could run over each character, and then covert it to lowercase if it's in uppercase, to uppercase if it's in lowercase or take it as is if it's neither (if it's a comma, colon, etc):
str = 'Hi, Stack Overflow.';
res = '';
for (var i = 0; i < str.length; ++i) {
c = str[i];
if (c == c.toUpperCase()) {
res += c.toLowerCase();
} else if (c == c.toLowerCase()) {
res += c.toUpperCase();
} else {
res += c;
}
}
Solution 2
You can try this simple solution using map()
var a = 'Hi, Stack Overflow!'
var ans = a.split('').map(function(c){
return c === c.toUpperCase()
? c.toLowerCase()
: c.toUpperCase()
}).join('')
console.log(ans)
Solution 3
<!DOCTYPE html>
<html>
<head>
<title>hello</title>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/1.12.4/jquery.min.js"></script>
</head>
<body>
<script>
$(document).ready(function(){
var x = 'Hi, Stack Overflow.';
alert(caseAlter(x));
function caseAlter(txt){
var output = "";
for(var i = 0; i < txt.length; i++){
if(txt[i] == txt[i].toUpperCase()){
output += txt[i].toLowerCase();
}else{
output += txt[i].toUpperCase();
}
}
return output;
}
});
</script>
</body>
</html>
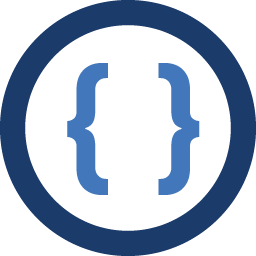
Author by
Admin
Updated on February 18, 2022Comments
-
Admin about 2 years
I want to make code that converts uppercase and lowercase in JavaScript.
For example, 'Hi, Stack Overflow.' → 'hI, sTACK oVERFLOW'
How can I do it?