Converting A String To Hexadecimal In Java
Solution 1
Here's a short way to convert it to hex:
public String toHex(String arg) {
return String.format("%040x", new BigInteger(1, arg.getBytes(/*YOUR_CHARSET?*/)));
}
Solution 2
To ensure that the hex is always 40 characters long, the BigInteger has to be positive:
public String toHex(String arg) {
return String.format("%x", new BigInteger(1, arg.getBytes(/*YOUR_CHARSET?*/)));
}
Solution 3
import org.apache.commons.codec.binary.Hex;
...
String hexString = Hex.encodeHexString(myString.getBytes(/* charset */));
http://commons.apache.org/codec/apidocs/org/apache/commons/codec/binary/Hex.html
Solution 4
Use DatatypeConverter.printHexBinary()
:
public static String toHexadecimal(String text) throws UnsupportedEncodingException
{
byte[] myBytes = text.getBytes("UTF-8");
return DatatypeConverter.printHexBinary(myBytes);
}
Example usage:
System.out.println(toHexadecimal("Hello StackOverflow"));
Prints:
48656C6C6F20537461636B4F766572666C6F77
Note: This causes a little extra trouble with Java 9
and newer since the API is not included by default. For reference e.g. see this GitHub
issue.
Solution 5
The numbers that you encode into hexadecimal must represent some encoding of the characters, such as UTF-8. So first convert the String to a byte[] representing the string in that encoding, then convert each byte to hexadecimal.
public static String hexadecimal(String input, String charsetName) throws UnsupportedEncodingException {
if (input == null) throw new NullPointerException();
return asHex(input.getBytes(charsetName));
}
private static final char[] HEX_CHARS = "0123456789abcdef".toCharArray();
public static String asHex(byte[] buf)
{
char[] chars = new char[2 * buf.length];
for (int i = 0; i < buf.length; ++i)
{
chars[2 * i] = HEX_CHARS[(buf[i] & 0xF0) >>> 4];
chars[2 * i + 1] = HEX_CHARS[buf[i] & 0x0F];
}
return new String(chars);
}
Related videos on Youtube
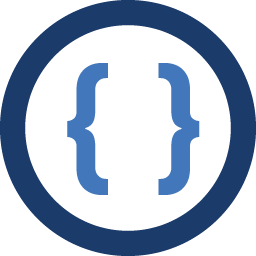
Admin
Updated on July 08, 2022Comments
-
Admin almost 2 years
I am trying to convert a string like "testing123" into hexadecimal form in java. I am currently using BlueJ.
And to convert it back, is it the same thing except backward?
-
rodion almost 15 yearsThanks for the correction, Software Monkey. I was pretty tired when I wrote the answer, and my test for 'raw[i] <= 9' is clearly insufficient.
-
Eduardo Costa almost 13 years+1 to the most pure sample of 3vilness I ever saw: using a BigInteger to convert from a byte[]...
-
laher over 12 yearsLove it! No loops and no bit-flipping. I want to give you 0xFF upvotes :)
-
Ron over 12 yearsto ensure 40 Characters, you should add zero padding: return String.format("%040x", new BigInteger(arg.getBytes(/*YOUR_CHARSET?*/)));
-
Roman Rdgz over 12 yearswhat about converting negative values which use 8 Characters and want them to fit in only 4?
-
hudolejev about 12 yearsThis method is actually the correct one. Try
byte[] data = { -1, 1 };
-- code in this answer works fine, whereas that with 17 upvotes fails. -
artaxerxe about 12 years@Kaleb Have you idea if possible to convert resulted String back? If, yes, can you give me some hints? Thanks!
-
Federico Zancan over 11 yearsInteresting, if you don't want to reinvent the wheel.
-
Ben Holland over 11 yearsJust add if(arg.equals("")){return ""} before the return line.
-
Kaleb Pederson over 11 yearsIs it possible to get a byte with value
-1
out of a string (as was requested in the example)? -
mike jones about 11 yearsNice but I would use
format("%02x")
so format() always uses 2 chars. Even though ASCII is double digit hex ie A=0x65 -
Joni over 10 yearsYou have to use the
BigInteger(int,byte[])
constructor; otherwise if the first byte is negative you get a negative BigInteger. -
Walter Tross almost 10 yearsI had a
byte[] bytes
as input, and landed on this answer. This is what you need in this case:return bytes.length == 0 ? "" : String.format("%0" + (bytes.length * 2) + "x", new BigInteger(1, bytes));
-
BxlSofty over 9 years@MelNicholson there's a decodeHex function in Hex to go to a byte[]. You need to use that because nothing guarantees that a random HEX string can be converted to a string in your encoding.
-
Jasper over 8 yearsHow to do the reverse - hex to String?
-
Admin over 8 yearsThis works really good, is there a way to reverse the generated hex back to string again?
-
Viswanath Lekshmanan almost 8 yearsWhere is str in this ?
-
Jonathan Rosenne over 5 yearsThis is fine if what you want is to see the internal representation of the Java characters, which is UTF-16, a specific representation of Unicode.
-
Nic over 5 years@KalebPederson Yes. It's not even very hard.. If your chosen encoding ever uses the most significant bit of any character (say, like UTF-* do), you have negative
byte
s in your array. -
skomisa about 5 yearsThis is not a solution. The question is asking how to get the hex representation of the content of an arbitrary String, and specifically provided "testing123" as an example.
-
Boris over 4 yearsThis is an interesting solution and one that strikes at the core of digital representation of data. Could you please explain what you are doing though, and what the "magic numbers" in your solution represent? A newcomer may not know what the >>> operator means, why we use the bitwise-and & along with a mask of 0xF0, or why the chars array is of size [2 * buf.length].
-
spikeyang over 4 yearsNice! Only on line 13, ">>>" should be ">>"
-
BluEOS over 3 yearsPlease don't use this "solution", it's by far the slowest way to do this kind of conversion.
-
Martin over 3 yearsThis prints leading zeroes even if they are not actually in memory. Java does not always use UTF-16 anymore.
-
KeyKi over 2 yearsIt can lose the first
0
, for example for SHA512(SHA512("hello")) it starts with592a
while should start with0592
.