Translating a String containing a binary value to Hex
Solution 1
Try using Integer.parseInt(binOutput, 2)
instead of Integer.parseInt(binOutput)
Solution 2
Ted Hopp beat me to it, but here goes anyway:
[email protected]:/tmp$ cat test.java; java test 000010001010011
public class test {
public static void main(String[] args) {
for (int i = 0; i < args.length; i++) {
System.out.println("The value of " + args[i] + " is " +
Integer.toHexString(Integer.parseInt(args[i], 2)));
}
}
}
The value of 000010001010011 is 453
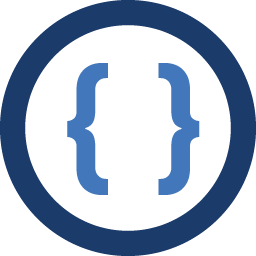
Author by
Admin
Updated on June 15, 2022Comments
-
Admin about 2 months