how to re-enable default after doing event.preventDefault()
Solution 1
I'm not sure this is the right way to handle it.
A better way to approach this problem would be to put some kind of check inside your document.keypress instructions.. like..
var enableKeys = false;
$(document).keypress(
function (event) {
// Pressing Up or Right: Advance to next video
if (event.keyCode == 40 || event.keyCode == 39 && enableKeys) {
event.preventDefault();
$(".current").next().click();
}
// Pressing Down or Left: Back to previous video
else if (event.keyCode == 38 || event.keyCode == 37 && enableKeys) {
event.preventDefault();
$(".current").prev().click();
}
}
);
Then control the enablekeys wherever you feel necessary, either with a hover, or something along those lines.
Solution 2
Adding a new handler doesn't replace the previous one, it adds a new one. You may be looking for jQuery#unbind
if you're trying to remove the previous handler, but if you're going to be turning this on and off a lot, you probably would be better off with a flag telling you whether to prevent the default or not in your existing handler.
Adding, and later removing, a handler looks like this:
function keypressHandler() { /* ... */};
$('#thingy').keypress(keypressHandler);
// ...elsewhere...
$('#thingy').unbind('keypress', keypressHandler);
Solution 3
function(e){ e.preventDefault(); }
and its opposite
function(e){ return true; }
Related videos on Youtube
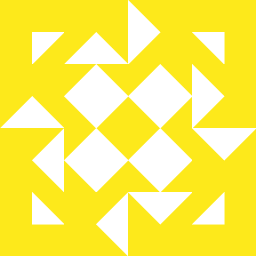
Matt
Updated on June 04, 2022Comments
-
Matt 4 months
I know this exact question was asked here, but the answer didn't work for what I needed to do so I figured I'd give some example code and explain a bit...
$(document).keypress( function (event) { // Pressing Up or Right: Advance to next video if (event.keyCode == 40 || event.keyCode == 39) { event.preventDefault(); $(".current").next().click(); } // Pressing Down or Left: Back to previous video else if (event.keyCode == 38 || event.keyCode == 37) { event.preventDefault(); $(".current").prev().click(); } } );
It basically disables the arrow keys to use them for something else, but doing:
$(document).keypress(function () { });
doesn't enable the default function again... I need it to scroll the page without having to create a scroll function for it...
Any ideas?
Thanks,
Matt -
Matt over 12 yearsWhat do you mean? How would that help re-enable the default action?
-
Diodeus - James MacFarlane over 12 yearsIt would bypass "preventdefault()", would it not?
-
Jørn Schou-Rode over 12 years+1 I am not sure if this is "a better way", but it is definitely a viable alternative if, for whatever reason, T.J. Crowder's solution is unusable in some application.
-
Matt over 12 yearsYour answer seemed to be a little closer to the proper way to do it, but I couldn't get it to work and danp's worked right away.
-
Matt over 12 yearsWorked right away, guess it helped that it matched up exactly to my code. Thanks!
-
T.J. Crowder over 12 years@Matt: Glad it got sorted. Actually, danp's answer is the same as the earlier one by Diodeus, it's just a lot more thoroughly described (which counts for a lot!). :-)