Could not find com.google.firebase:firebase-crashlytics flutter
3,385
Solution 1
You need to implement the dependencies in app-level gradle file app/build.gradle
// Add the Firebase Crashlytics SDK.
implementation 'com.google.firebase:firebase-crashlytics:17.2.2'
// Recommended: Add the Google Analytics SDK.
implementation 'com.google.firebase:firebase-analytics:18.0.0'
The dependencies section should look like this
dependencies {
implementation "org.jetbrains.kotlin:kotlin-stdlib-jdk7:$kotlin_version"
implementation 'com.android.support:multidex:1.0.3'
// Add the Firebase Crashlytics SDK.
implementation 'com.google.firebase:firebase-crashlytics:17.2.2'
// Recommended: Add the Google Analytics SDK.
implementation 'com.google.firebase:firebase-analytics:18.0.0'
}
I hope this will fix your issue.
Solution 2
try changing the version in android/build.gradle:
classpath 'com.google.gms:google-services:4.3.3'
classpath 'com.google.firebase:firebase-crashlytics-gradle:2.2.0'
I hope I've helped
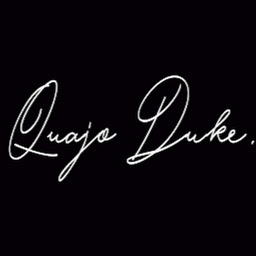
Author by
Quajo Duke
Updated on December 25, 2022Comments
-
Quajo Duke over 1 year
I am not experienced in flutter but been doing it for a while. My current project is using firebase so I'm using flutterfire package and it has to be my worst nightmare so far. Build fail upon build fail. Note IOS is working though. The recent is this
FAILURE: Build failed with an exception. * What went wrong: Could not determine the dependencies of task ':firebase_crashlytics:compileDebugAidl'. > Could not resolve all task dependencies for configuration ':firebase_crashlytics:debugCompileClasspath'. > Could not find com.google.firebase:firebase-crashlytics:. Required by: project :firebase_crashlytics * Try: Run with --stacktrace option to get the stack trace. Run with --info or --debug option to get more log output. Run with --scan to get full insights. * Get more help at https://help.gradle.org BUILD FAILED in 1s Exception: Gradle task assembleDebug failed with exit code 1
android/build.gradle
buildscript { ext.kotlin_version = '1.3.50' repositories { google() jcenter() } dependencies { classpath "com.android.tools.build:gradle:3.5.0" classpath "org.jetbrains.kotlin:kotlin-gradle-plugin:$kotlin_version" classpath "com.google.gms:google-services:4.3.4" classpath "com.google.firebase:firebase-crashlytics-gradle:2.3.0" } } allprojects { repositories { google() jcenter() } } rootProject.buildDir = '../build' subprojects { project.buildDir = "${rootProject.buildDir}/${project.name}" } subprojects { project.evaluationDependsOn(':app') gradle.projectsEvaluated { tasks.withType(JavaCompile) { options.compilerArgs << "-Xlint:deprecation" << "-Xlint:unchecked" } } project.configurations.all { resolutionStrategy.eachDependency { details -> if (details.requested.group == 'androidx.core' && !details.requested.name.contains('androidx')) { details.useVersion "1.0.1" } } } } task clean(type: Delete) { delete rootProject.buildDir }
app/build.gradle
def localProperties = new Properties() def localPropertiesFile = rootProject.file('local.properties') if (localPropertiesFile.exists()) { localPropertiesFile.withReader('UTF-8') { reader -> localProperties.load(reader) } } def flutterRoot = localProperties.getProperty('flutter.sdk') if (flutterRoot == null) { throw new GradleException("Flutter SDK not found. Define location with flutter.sdk in the local.properties file.") } def flutterVersionCode = localProperties.getProperty('flutter.versionCode') if (flutterVersionCode == null) { flutterVersionCode = '1' } def flutterVersionName = localProperties.getProperty('flutter.versionName') if (flutterVersionName == null) { flutterVersionName = '1.0' } apply plugin: 'com.android.application' apply plugin: 'kotlin-android' apply from: "$flutterRoot/packages/flutter_tools/gradle/flutter.gradle" apply plugin: 'com.google.gms.google-services' apply plugin: 'com.google.firebase.crashlytics' android { compileSdkVersion 28 defaultConfig { minSdkVersion 21 targetSdkVersion 28 multiDexEnabled true } sourceSets { main.java.srcDirs += 'src/main/kotlin' } lintOptions { disable 'InvalidPackage' } defaultConfig { // TODO: Specify your own unique Application ID (https://developer.android.com/studio/build/application-id.html). applicationId "com.tendo.app" minSdkVersion 17 targetSdkVersion 28 versionCode flutterVersionCode.toInteger() versionName flutterVersionName multiDexEnabled true } buildTypes { release { // TODO: Add your own signing config for the release build. // Signing with the debug keys for now, so `flutter run --release` works. signingConfig signingConfigs.debug } } } rootProject.ext { set('FlutterFire', [ FirebaseSDKVersion: '21.1.0' ]) } flutter { source '../..' } dependencies { implementation "org.jetbrains.kotlin:kotlin-stdlib-jdk7:$kotlin_version" implementation 'com.android.support:multidex:1.0.3' implementation platform('com.google.firebase:firebase-bom:25.12.0') }
-
Quajo Duke over 3 yearsI have done that over and over the current version you are seeing is what i got from the official doc of firebase. I know this version is from the docs from fluttterfire. Yet still i tried your answer and still build fail :(
-
dipakbari4 over 3 yearsI also would like to recommend you to follow this Initialize Crashlytics to specific dependencies setup for Java / Kotlin
-
Quajo Duke over 3 yearsI tried it and I just did this and still same build failure and same message :(
-
dipakbari4 over 3 yearsI've update the code. Once try to update in your code. Hope this will work.