Could not find matching function to call loaded from the SavedModel
Solution 1
Note: I think your problem is on Predict Model part. In that part you have used x_test[0] which is not matching with the pre-trained model array dimension. You have to use x_test instead of x_test[0]. enter image description here
#Use This Code TO Solve Your Problem
import tensorflow as tf # deep learning library. Tensors are just multi-dimensional arrays
mnist = tf.keras.datasets.mnist # mnist is a dataset of 28x28 images of handwritten digits and their labels
(x_train, y_train),(x_test, y_test) = mnist.load_data() # unpacks images to x_train/x_test and labels to y_train/y_test
x_train = tf.keras.utils.normalize(x_train, axis=1) # scales data between 0 and 1
x_test = tf.keras.utils.normalize(x_test, axis=1) # scales data between 0 and 1
model = tf.keras.models.Sequential() # a basic feed-forward model
model.add(tf.keras.layers.Flatten()) # takes our 28x28 and makes it 1x784
model.add(tf.keras.layers.Dense(128, activation=tf.nn.relu)) # a simple fully-connected layer, 128 units, relu activation
model.add(tf.keras.layers.Dense(128, activation=tf.nn.relu)) # a simple fully-connected layer, 128 units, relu activation
model.add(tf.keras.layers.Dense(10, activation=tf.nn.softmax)) # our output layer. 10 units for 10 classes. Softmax for probability distribution
model.compile(optimizer='adam', # Good default optimizer to start with
loss='sparse_categorical_crossentropy', # how will we calculate our "error." Neural network aims to minimize loss.
metrics=['accuracy']) # what to track
model.fit(x_train, y_train, epochs=3) # train the model
val_loss, val_acc = model.evaluate(x_test, y_test) # evaluate the out of sample data with model
print(val_loss) # model's loss (error)
print(val_acc) # model's accuracy
--------------------------Save Model----------------------------------------
model.save('epic_num_reader.model') # save the model
--------------------------Load Model----------------------------------------
new_model = tf.keras.models.load_model('epic_num_reader.model') # Load the model
--------------------------Predict Model-------------------------------------
predictions = new_model.predict(x_test)
print(predictions)
--------------------------visualize Prediction------------------------------
plt.imshow(x_test[0],cmap=plt.cm.binary)
plt.show()
-------------------------- Validated Prediction-----------------------------
import numpy as np
print(np.argmax(predictions[0]))
Solution 2
Had the same issue. Try:
pip install tf-nightly
Solution is from here: https://github.com/tensorflow/tensorflow/issues/35446 - comment from oanush.
But this may break calling of tensorboards if you have one. Step by step solution for this is below:
pip uninstall tensorflow
pip uninstall tensorboard
pip install -q tf-nightly
pip install --ignore-installed tf-nightly
Got if from here: https://github.com/tensorflow/tensorboard/issues/2226 - comment from mmehedin, 29 Jun 2019.
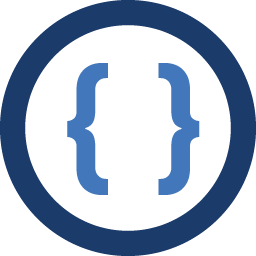
Admin
Updated on June 05, 2022Comments
-
Admin almost 2 years
I wrote below code and it suppose to load a model followed by a predictive run of an element from MNIST dataset. At the beginning of the execution the code works fine and I get my desired prediction, but then suddenly I did get the below error following error and I'm not sure if this could be related to
.predict arguments
.My code:
# importing libraries import tensorflow as tf # deep learning library. Tensors are just multi-dimensional arrays import gzip,sys,pickle # dataset manipulation library # importing MNIST dataset f = gzip.open('mnist.pkl.gz', 'rb') if sys.version_info < (3,): data = pickle.load(f) else: data = pickle.load(f, encoding='bytes') f.close() (x_train, _), (x_test, _) = data print("-----------------------dataset ready-----------------------") # using an expample from x_test / to remove later # preprocessing x_test = tf.keras.utils.normalize(x_test, axis=1) # scales data between 0 and 1 # importing model new_model = tf.keras.models.load_model('epic_num_reader.model') print("-----------------------model ready-----------------------") # getting prediction predictions = new_model.predict(x_test[0]) import numpy as np print("-----------------------predection ready-----------------------") print(np.argmax(predictions))
The error message:
-----------------------dataset ready----------------------- 2019-10-27 00:36:58.767359: I tensorflow/core/platform/cpu_feature_guard.cc:142] Your CPU supports instructions that this TensorFlow binary was not compiled to use: AVX2 -----------------------model ready----------------------- Traceback (most recent call last): File "c:\Users\lotfi\Desktop\DigitsDetector\main1.py", line 24, in <module> predictions = new_model.predict(x_test[0]) File "C:\Users\lotfi\Anaconda3\envs\tf\lib\site-packages\tensorflow_core\python\keras\engine\training.py", line 909, in predict use_multiprocessing=use_multiprocessing) File "C:\Users\lotfi\Anaconda3\envs\tf\lib\site-packages\tensorflow_core\python\keras\engine\training_v2.py", line 462, in predict steps=steps, callbacks=callbacks, **kwargs) File "C:\Users\lotfi\Anaconda3\envs\tf\lib\site-packages\tensorflow_core\python\keras\engine\training_v2.py", line 444, in _model_iteration total_epochs=1) File "C:\Users\lotfi\Anaconda3\envs\tf\lib\site-packages\tensorflow_core\python\keras\engine\training_v2.py", line 123, in run_one_epoch batch_outs = execution_function(iterator) File "C:\Users\lotfi\Anaconda3\envs\tf\lib\site-packages\tensorflow_core\python\keras\engine\training_v2_utils.py", line 86, in execution_function distributed_function(input_fn)) File "C:\Users\lotfi\Anaconda3\envs\tf\lib\site-packages\tensorflow_core\python\eager\def_function.py", line 457, in __call__ result = self._call(*args, **kwds) File "C:\Users\lotfi\Anaconda3\envs\tf\lib\site-packages\tensorflow_core\python\eager\def_function.py", line 503, in _call self._initialize(args, kwds, add_initializers_to=initializer_map) File "C:\Users\lotfi\Anaconda3\envs\tf\lib\site-packages\tensorflow_core\python\eager\def_function.py", line 408, in _initialize *args, **kwds)) File "C:\Users\lotfi\Anaconda3\envs\tf\lib\site-packages\tensorflow_core\python\eager\function.py", line 1848, in _get_concrete_function_internal_garbage_collected graph_function, _, _ = self._maybe_define_function(args, kwargs) File "C:\Users\lotfi\Anaconda3\envs\tf\lib\site-packages\tensorflow_core\python\eager\function.py", line 2150, in _maybe_define_function graph_function = self._create_graph_function(args, kwargs) File "C:\Users\lotfi\Anaconda3\envs\tf\lib\site-packages\tensorflow_core\python\eager\function.py", line 2041, in _create_graph_function capture_by_value=self._capture_by_value), File "C:\Users\lotfi\Anaconda3\envs\tf\lib\site-packages\tensorflow_core\python\framework\func_graph.py", line 915, in func_graph_from_py_func func_outputs = python_func(*func_args, **func_kwargs) File "C:\Users\lotfi\Anaconda3\envs\tf\lib\site-packages\tensorflow_core\python\eager\def_function.py", line 358, in wrapped_fn return weak_wrapped_fn().__wrapped__(*args, **kwds) File "C:\Users\lotfi\Anaconda3\envs\tf\lib\site-packages\tensorflow_core\python\keras\engine\training_v2_utils.py", line 73, in distributed_function per_replica_function, args=(model, x, y, sample_weights)) File "C:\Users\lotfi\Anaconda3\envs\tf\lib\site-packages\tensorflow_core\python\distribute\distribute_lib.py", line 760, in experimental_run_v2 return self._extended.call_for_each_replica(fn, args=args, kwargs=kwargs) File "C:\Users\lotfi\Anaconda3\envs\tf\lib\site-packages\tensorflow_core\python\distribute\distribute_lib.py", line 1787, in call_for_each_replica return self._call_for_each_replica(fn, args, kwargs) File "C:\Users\lotfi\Anaconda3\envs\tf\lib\site-packages\tensorflow_core\python\distribute\distribute_lib.py", line 2132, in _call_for_each_replica return fn(*args, **kwargs) File "C:\Users\lotfi\Anaconda3\envs\tf\lib\site-packages\tensorflow_core\python\autograph\impl\api.py", line 292, in wrapper return func(*args, **kwargs) File "C:\Users\lotfi\Anaconda3\envs\tf\lib\site-packages\tensorflow_core\python\keras\engine\training_v2_utils.py", line 162, in _predict_on_batch return predict_on_batch(model, x) File "C:\Users\lotfi\Anaconda3\envs\tf\lib\site-packages\tensorflow_core\python\keras\engine\training_v2_utils.py", line 370, in predict_on_batch return model(inputs) # pylint: disable=not-callable File "C:\Users\lotfi\Anaconda3\envs\tf\lib\site-packages\tensorflow_core\python\keras\engine\base_layer.py", line 847, in __call__ outputs = call_fn(cast_inputs, *args, **kwargs) File "C:\Users\lotfi\Anaconda3\envs\tf\lib\site-packages\tensorflow_core\python\keras\engine\sequential.py", line 270, in call outputs = layer(inputs, **kwargs) File "C:\Users\lotfi\Anaconda3\envs\tf\lib\site-packages\tensorflow_core\python\keras\engine\base_layer.py", line 847, in __call__ outputs = call_fn(cast_inputs, *args, **kwargs) File "C:\Users\lotfi\Anaconda3\envs\tf\lib\site-packages\tensorflow_core\python\keras\saving\saved_model\utils.py", line 57, in return_outputs_and_add_losses outputs, losses = fn(inputs, *args, **kwargs) File "C:\Users\lotfi\Anaconda3\envs\tf\lib\site-packages\tensorflow_core\python\eager\def_function.py", line 457, in __call__ result = self._call(*args, **kwds) File "C:\Users\lotfi\Anaconda3\envs\tf\lib\site-packages\tensorflow_core\python\eager\def_function.py", line 494, in _call results = self._stateful_fn(*args, **kwds) File "C:\Users\lotfi\Anaconda3\envs\tf\lib\site-packages\tensorflow_core\python\eager\function.py", line 1822, in __call__ graph_function, args, kwargs = self._maybe_define_function(args, kwargs) File "C:\Users\lotfi\Anaconda3\envs\tf\lib\site-packages\tensorflow_core\python\eager\function.py", line 2150, in _maybe_define_function graph_function = self._create_graph_function(args, kwargs) File "C:\Users\lotfi\Anaconda3\envs\tf\lib\site-packages\tensorflow_core\python\eager\function.py", line 2041, in _create_graph_function capture_by_value=self._capture_by_value), File "C:\Users\lotfi\Anaconda3\envs\tf\lib\site-packages\tensorflow_core\python\framework\func_graph.py", line 915, in func_graph_from_py_func func_outputs = python_func(*func_args, **func_kwargs) File "C:\Users\lotfi\Anaconda3\envs\tf\lib\site-packages\tensorflow_core\python\eager\def_function.py", line 358, in wrapped_fn return weak_wrapped_fn().__wrapped__(*args, **kwds) File "C:\Users\lotfi\Anaconda3\envs\tf\lib\site-packages\tensorflow_core\python\saved_model\function_deserialization.py", line 262, in restored_function_body "\n\n".join(signature_descriptions)))
Error message continued:
ValueError: Could not find matching function to call loaded from the SavedModel. Got: Positional arguments (1 total): * Tensor("inputs:0", shape=(None, 28), dtype=float32) Keyword arguments: {} Expected these arguments to match one of the following 1 option(s): Option 1: Positional arguments (1 total): * TensorSpec(shape=(None, 28, 28), dtype=tf.float32, name='inputs') Keyword arguments: {}