creating a reverse method for a python list from scratch
Solution 1
You are changing the list that you iterate on it (data_list) because of that it's not working , try like this:
def reverse(data_list):
length = len(data_list)
s = length
new_list = [None]*length
for item in data_list:
s = s - 1
new_list[s] = item
return new_list
Solution 2
def reverse(data_list):
return data_list[::-1]
>> reverse([1,2,3,4,5]) [5, 4, 3, 2, 1]
Solution 3
By the time you are half-way through the list, you have swapped all the items; as you continue through the second half, you are swapping them all back to their original locations again.
Instead try
def reverse(lst):
i = 0 # first item
j = len(lst)-1 # last item
while i<j:
lst[i],lst[j] = lst[j],lst[i]
i += 1
j -= 1
return lst
This can be used in two ways:
a = [1,2,3,4,5]
reverse(a) # in-place
print a # -> [5,4,3,2,1]
b = reverse(a[:]) # return the result of reversing a copy of a
print a # -> [5,4,3,2,1]
print b # -> [1,2,3,4,5]
Solution 4
an easy way in python (without using the reverse function) is using the [] access operator with negative values such as (print and create a new list in reverse order):
x = [1, 2 ,3, 4, 5]
newx = []
for i in range(1, len(x)+1):
newx.append(x[-i])
print x[-i]
the function would be:
def reverse(list):
newlist = []
for i in range(1, len(list)+1):
newlist.append(list[-1])
return newlist
Solution 5
There are two simple ways to solve the problem : First using a temp variable :
maList = [2,5,67,8,99,34]
halfLen = len(maList) // 2
for index in range(halfLen):
temp = maList[index]
maList[index] = maList[len(maList) - 1 - index]
maList[len(maList) - 1 - index] = temp
print(maList)
Second is using a new list where to store the reversed values :
newList = []
for index,value in enumerate(maList):
newList.append(maList[len(maList) - 1 - index])
print(newList)
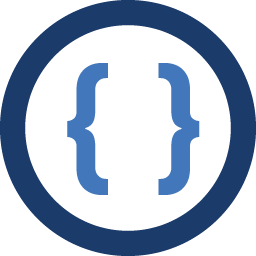
Admin
Updated on August 22, 2022Comments
-
Admin over 1 year
I want to create a reverse method for a list. I know there already is such a method built into python but I want to try it from scratch. Here's what I have and it seems to make sense to me but it just returns the list in the same order. My understanding was that lists are mutable and I could just reassign values in the loop.
def reverse(data_list): length = len(data_list) s = length for item in data_list: s = s - 1 data_list[s] = item return data_list