Cross-browser method for detecting the scrollTop of the browser window
Solution 1
function getScrollTop(){
if(typeof pageYOffset!= 'undefined'){
//most browsers except IE before #9
return pageYOffset;
}
else{
var B= document.body; //IE 'quirks'
var D= document.documentElement; //IE with doctype
D= (D.clientHeight)? D: B;
return D.scrollTop;
}
}
alert(getScrollTop())
Solution 2
Or just simple as:
var scrollTop = document.body.scrollTop || document.documentElement.scrollTop;
Solution 3
If you don't want to include a whole JavaScript library, you can often extract the bits you want from one.
For example, this is essentially how jQuery implements a cross-browser scroll(Top|Left):
function getScroll(method, element) {
// The passed in `method` value should be 'Top' or 'Left'
method = 'scroll' + method;
return (element == window || element == document) ? (
self[(method == 'scrollTop') ? 'pageYOffset' : 'pageXOffset'] ||
(browserSupportsBoxModel && document.documentElement[method]) ||
document.body[method]
) : element[method];
}
getScroll('Top', element);
getScroll('Left', element);
Note: you'll notice that the above code contains a browserSupportsBoxModel
variable which is undefined. jQuery defines this by temporarily adding a div to the page and then measuring some attributes in order to determine whether the browser correctly implements the box model. As you can imagine this flag checks for IE. Specifically, it checks for IE 6 or 7 in quirks mode. Since the detection is rather complex, I've left it as an exercise for you ;-), assuming you have already used browser feature detection elsewhere in your code.
Edit: If you haven't guessed already, I strongly suggest you use a library for these sorts of things. The overhead is a small price to pay for robust and future-proof code and anyone would be much more productive with a cross-browser framework to build upon. (As opposed to spending countless hours banging your head against IE).
Solution 4
I've been using window.scrollY || document.documentElement.scrollTop
.
window.scrollY
covers all browsers except IEs.
document.documentElement.scrollTop
covers IE.
Solution 5
function getSize(method) {
return document.documentElement[method] || document.body[method];
}
getSize("scrollTop");
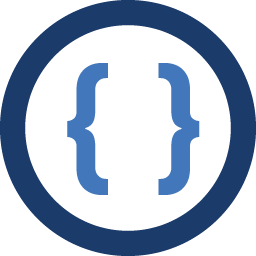
Admin
Updated on July 08, 2022Comments
-
Admin almost 2 years
What is the best cross-browser way to detect the scrollTop of the browser window? I prefer not to use any pre-built code libraries because this is a very simple script, and I don't need all of that deadweight.
-
iancrowther about 11 yearsFYI - The question states "I prefer not to use any pre-built code libraries". You got marked down as mooTools is still a pre-built code library. Thanks for taking the time to contribute, just please read the question a bit more thoroughly or your rep will take a hit
-
hypervisor666 almost 11 yearsThis method doesn't seem to work with Firefox browsers on Mac or Linux.
-
jave.web almost 11 yearsugly alert! use console and console.log instead! , anyway good answer, but what are the browser support of the "//most browsers" - or better IEwhatnumber+ is supporting that ?
-
Neil Monroe almost 11 yearsAlso, another problem with this approach that if you are trying to detect it for the main window scrollbar, non-IE browsers will scroll the <body> element and IE will scroll <html>. So, you have to figure out what 'element' is first.
-
Yeti over 10 yearsWouldn't work on iPad and possibly other devices/browsers (Chrome?), you will need
window.pageYOffset
there. -
Progo almost 10 yearsThis isn't cross browser.
-
flu almost 10 yearsWorks for me in mobile Safari 5 and 7, FireFox 31, Chrome 37, Opera 24, IE 5-11. According to quirksmode.org/dom/w3c_cssom.html#t35 this is so cross-browser. So thank you! Short'n'easy.
-
Roman over 9 yearsis this short version correct enough?
var scrollTop = pageYOffset || (document.documentElement.clientHeight ? document.documentElement.scrollTop : document.body.scrollTop);
-
fjaguero almost 9 yearsThis is the most useful answer here, without even needing a function (in terms of style).
-
Manngo over 7 yearsThe first expression can also be
window.pageYOffset
. I mention this becausepageYOffset
seems to be more historical, and I don’t seescrollY
quite so often. According to MDN, they’re the same. In any case, this is by far the cleanest and most direct solution, and should be preferred because it addresses modern browsers first, thus not punishing them for being up-to-date. -
Manngo over 7 years@Jason: I meant to add that I was talking about your answer to the question being the cleanest & most direct, rather than my own comment above.
-
John almost 6 years@Roman I think it would only fail if pageYOffset were 0 which would probably mean the other part would be undefined judging by other comments on this page, so if you just add an or 0 at the end like so it should work. Like so,
var scrollTop = pageYOffset || (document.documentElement.clientHeight ? document.documentElement.scrollTop : document.body.scrollTop) || 0;
-
Harti almost 6 yearsWhen the scroll position is 0, this will return
<body></body>
when logging this to the console. Most likely this is happening due to this being a one-liner and a document.body.scrollTop of 0 equals false, thus returning the scrollingElement. -
Loren about 4 yearsUse window.pageYOffset instead of just pageYOffset for Brave Browser to avoid intermittent undefined errors when called before window is available.
-
blackr1234 almost 4 yearsShould the last item be
document.scrollingElement.scrollTop
instead ofdocument.scrollingElement
?