Dart compare unordered Lists with Objects
Solution 1
As you said , those are different objects, you need to override the equal operator of your class, like this:
class Article {
final String name;
final String id;
const Article({this.id,this.name});
@override
bool operator ==(Object other) =>
identical(this, other) ||
other is Article &&
runtimeType == other.runtimeType &&
id == other.id;
@override
int get hashCode => id.hashCode;
}
Then the result will be true, because the ids are the same.
Also you can take a look at this package
https://pub.dev/packages/equatable
Solution 2
As my solution I used the "equatable"- package: https://pub.dev/packages/equatable
For checking an unordered List, I use DeepCollectionEquality.unordered().equals instead of listEquals
var a = List<Article>();
var b = List<Article>();
var article1 = Article(id: "1", name: "Beer");
var article2 = Article(id: "2", name: "Tequilla");
var article3 = Article(id: "3", name: "VodkaBull");
var article4 = Article(id: "1", name: "Beer");
var article5 = Article(id: "2", name: "Tequilla");
var article6 = Article(id: "3", name: "VodkaBull");
a.add(article1);
a.add(article2);
a.add(article3);
b.add(article4);
b.add(article5);
b.add(article6);
print(listEquals(a, b));
print(DeepCollectionEquality.unordered().equals(a, b));
The Code for my Article looks as following:
import 'package:equatable/equatable.dart';
class Article extends Equatable {
String id;
String name;
Article({this.id, this.name});
@override
// TODO: implement props
List<Object> get props => [id, name];
}
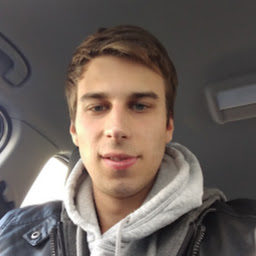
max
Updated on December 15, 2022Comments
-
max over 1 year
I have following problem. There are two Lists with Articles in it. In the following example the result prints me false. But the Articles are the same. I think thats, because these are different objects, if I add in both lists article1, it will be true.
I also tried DeepCollectionEquality.unordered().equals from this issue: How can I compare Lists for equality in Dart?
But it is also giving me FALSE back. In my real project, I have two Lists with Articles in it. These lists are not sorted, so one Article can be the first in one list, and an article with the same id and name can be the last one in the other list. But if both lists have the same articles (with the same name and id) it should result with true.
var a = List<Article>(); var b = List<Article>(); var article1 = Article(id: "1", name: "Beer"); var article2 = Article(id: "1", name: "Beer"); a.add(article1); b.add(article2); print(listEquals(a, b));