How to find the nearest number between flutter variables?
154
The code is a bit verbouse, but I hope it helps you understand, how you can approach writing logic in dart.
If you feel like optimizing this code, check out the different ways you can find a minimum value inside a list and combine it with the "lowest-difference to a number"-logic :)
Note: If you want a playground to check out code snippets like this, use the Dart Pad!
import 'dart:math';
void main() {
List numbersList = [-10, 22]; // play around with it
List<int> differenceList = []; // helper list
int compareNumber = -11; // play around with it
// 1. Check if identical number is in numbersList:
bool checkForIdenticalNumber() {
if(numbersList.contains(compareNumber)) {
print("The number $compareNumber is identical to the compareNumber");
return true;
}
return false;
}
// 2. Define checking logic:
void checkDifference(int number) {
if (number > compareNumber) {
int difference = number - compareNumber;
differenceList.add(difference);
} else {
int difference = compareNumber - number;
differenceList.add(difference);
}
}
// 3. Use Check forEach element on numbersList:
void findNearestNumber() {
numbersList.forEach(
(number) => checkDifference(number)
);
}
// 4. Identify the solution:
void checkForSolution() {
int index = differenceList.indexWhere((e) => e == differenceList.reduce(min));
print("The closest number is: ${numbersList[index]}");
}
// 5. Only execute logic, if the number to compare is not inside the numbersList:
bool isIdentical = checkForIdenticalNumber();
if (!isIdentical) {
findNearestNumber();
// print(numbersList);
// print(differenceList);
checkForSolution();
}
}
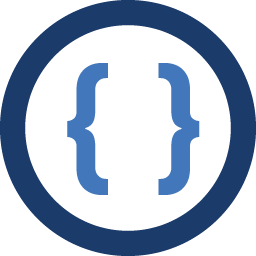
Author by
Admin
Updated on January 02, 2023Comments
-
Admin over 1 year
I have a number in an int variable (call it "a"), and i have 12 another int variables which contains a number. How could i find the nearest number to "a" from other variables? Should i use a List, or other compare method?