Data location must be "memory" for return parameter in function, but none was given
Solution 1
You should add memory keyword for string parameter, which was introduced in solidity version 0.5.0
As per the documentation:
Explicit data location for all variables of struct, array or mapping types is now mandatory. This is also applied to function parameters and return variables. For example, change uint[] x = m_x to uint[] storage x = m_x, and function f(uint[][] x) to function f(uint[][] memory x) where memory is the data location and might be replaced by storage or calldata accordingly. Note that external functions require parameters with a data location of calldata.
Corrected code
contract MyContract {
string value;
function get() public view returns (string memory) {
return value;
}
function set(string memory _value) public {
value = _value;
}
constructor() public {
value = "myValue";
}
}
Refer to official documentation on breaking changes made in version 0.5.0
Solution 2
Values of reference type can be modified through multiple different names. Contrast this with value types where you get an independent copy whenever a variable of value type is used. Because of that, reference types have to be handled more carefully than value types. Currently, reference types comprise structs, arrays and mappings. If you use a reference type, you always have to explicitly provide the data area where the type is stored: memory (whose lifetime is limited to an external function call), storage (the location where the state variables are stored, where the lifetime is limited to the lifetime of a contract) or calldata (special data location that contains the function arguments).
Warning
Prior to version 0.5.0 the data location could be omitted, and would default to different locations depending on the kind of variable, function type, etc., but all complex types must now give an explicit data location.
https://docs.soliditylang.org/en/latest/types.html#reference-types
so you have to put memory
or calldata
after String as follows:
contract MyContract {
string value;
function get() public view returns (string memory) {
return value;
}
function set(string memory _value) public {
value = _value;
}
constructor() {
value = "myValue";
}
}
another thing to notice that you dont have to put public in the constructor any more:
Warning: Prior to version 0.7.0, you had to specify the visibility of constructors as either internal or public.
https://docs.soliditylang.org/en/latest/contracts.html?highlight=constructor#constructors
Solution 3
In the case of returning address array, you can declare memory
after your return address type
.
function getAllPlayers() public view returns(address[] memory){
return players;
}
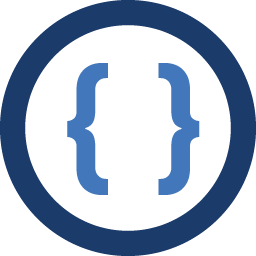
Admin
Updated on July 09, 2022Comments
-
Admin almost 2 years
I tried solidity example like as above in remix, solidity version > 0.5.0 But I am getting this error now. What is the way to solve this error?
contract MyContract { string value; function get() public view returns (string) { return value; } function set(string _value) public { value = _value; } constructor() public { value = "myValue"; } }
-
agentp almost 2 yearsCan you help to explain difference between
memory
andcalldata
and there use cases? -
Ahmad Altayeb almost 2 yearsThe simplest explanation is:
calldata
is a non-modifiable, non-persistent area where function arguments are stored, and behaves mostly like memory, it must be used when declaring an external function's dynamic parameters.memory
is mutable, non-persistent and used for both function declaration parameters, its ifetime is limited to a function call and it should be used when declaring variables (both function parameters as well as inside the logic of a function) that you want stored in memory (temporary)