Declaring an iterator in Java
Solution 1
By saying it can't be instantiated, what they mean is that you can't do Iterator i = new Iterator();
. Since Iterator is an interface, it has no implementation by itself. When you do
Iterator flavoursIter = aFlavours.iterator();
what you're doing is calling a method called "iterator" on the aFlavours
object that returns an instance of some class that implements the Iterator interface (you don't really know what exact class it is, nor should you care, all that you're guaranteed is that it supports all the methods defined in the Iterator interface).
Solution 2
As a complement to the other answers:
Many classes implement interfaces such as Iterator
as a private inner class, then return instances of that hidden class whenever an instance is requested. You can read the source code of the Collections framework if you want an example.
The main advantage of doing things this way is that you hide implementation details. A user of a List
, for example, does not have to know what concrete class is returned when calling someList.iterator ()
, nor does he need to know how many different implementations of Iteraor
are available in the Collections framework. This allows devellopers of this framework to create as many implementations as needed, based on their needs (concurency, efficiency, etc.), and you, as a user of the framework, do not have to learn zillion of classes.
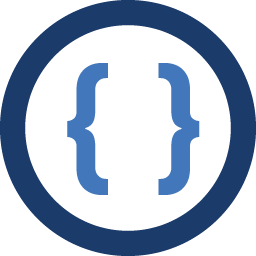
Admin
Updated on June 16, 2022Comments
-
Admin almost 2 years
I'm very confused about the iterator in Java.
The tutorial is was reading said this:
In the Java programming language, an interface is a reference type, similar to a class, that can contain only constants, method signatures, and nested types. There are no method bodies. Interfaces cannot be instantiated—they can only be implemented by classes or extended by other interfaces. Extension is discussed later in this lesson.
Then I saw another tutorial with this:
Iterator<String> flavoursIter = aFlavours.iterator();
I understand that aFlavours has inheritated the iterator functions which returns the iterator interface. I just don't understand what is actually happening in this line. I thought you couldn't instantiated?
Maybe I'm not making sense, but please tell me we're I'm going off the track.