Delete files from directory inside Document directory?
Solution 1
Two things, use the temp
directory and second pass an error to the fileManager.removeItemAtPath
and place it in a if to see what failed. Also you should not be checking if the error is set but rather whether a methods has return data.
func clearAllFilesFromTempDirectory(){
var error: NSErrorPointer = nil
let dirPath = NSSearchPathForDirectoriesInDomains(.DocumentDirectory, .UserDomainMask, true)[0] as! String
var tempDirPath = dirPath.stringByAppendingPathComponent("Temp")
var directoryContents: NSArray = fileManager.contentsOfDirectoryAtPath(tempDirPath, error: error)?
if directoryContents != nil {
for path in directoryContents {
let fullPath = dirPath.stringByAppendingPathComponent(path as! String)
if fileManager.removeItemAtPath(fullPath, error: error) == false {
println("Could not delete file: \(error)")
}
}
} else {
println("Could not retrieve directory: \(error)")
}
}
To get the correct temporary directory use NSTemporaryDirectory()
Solution 2
In case anyone needs this for the latest Swift / Xcode versions: here is an example to remove all files from the temp folder:
Swift 2.x:
func clearTempFolder() {
let fileManager = NSFileManager.defaultManager()
let tempFolderPath = NSTemporaryDirectory()
do {
let filePaths = try fileManager.contentsOfDirectoryAtPath(tempFolderPath)
for filePath in filePaths {
try fileManager.removeItemAtPath(tempFolderPath + filePath)
}
} catch {
print("Could not clear temp folder: \(error)")
}
}
Swift 3.x and Swift 4:
func clearTempFolder() {
let fileManager = FileManager.default
let tempFolderPath = NSTemporaryDirectory()
do {
let filePaths = try fileManager.contentsOfDirectory(atPath: tempFolderPath)
for filePath in filePaths {
try fileManager.removeItem(atPath: tempFolderPath + filePath)
}
} catch {
print("Could not clear temp folder: \(error)")
}
}
Solution 3
Swift 3:
func clearTempFolder() {
let fileManager = FileManager.default
let tempFolderPath = NSTemporaryDirectory()
do {
let filePaths = try fileManager.contentsOfDirectory(atPath: tempFolderPath)
for filePath in filePaths {
try fileManager.removeItem(atPath: NSTemporaryDirectory() + filePath)
}
} catch let error as NSError {
print("Could not clear temp folder: \(error.debugDescription)")
}
}
Solution 4
Swift 4.0 example that removes all files from an example folder "diskcache
" in the documents directory. I found the above examples unclear because they used the NSTemporaryDirectory() + filePath
which is not "url" style. For your convenience:
func clearDiskCache() {
let fileManager = FileManager.default
let myDocuments = fileManager.urls(for: .documentDirectory, in: .userDomainMask).first!
let diskCacheStorageBaseUrl = myDocuments.appendingPathComponent("diskCache")
guard let filePaths = try? fileManager.contentsOfDirectory(at: diskCacheStorageBaseUrl, includingPropertiesForKeys: nil, options: []) else { return }
for filePath in filePaths {
try? fileManager.removeItem(at: filePath)
}
}
Solution 5
Remove all files From Document Directory: Swift 4
func clearAllFile() {
let fileManager = FileManager.default
let myDocuments = fileManager.urls(for: .documentDirectory, in: .userDomainMask).first!
do {
try fileManager.removeItem(at: myDocuments)
} catch {
return
}
}
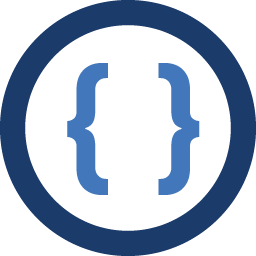
Admin
Updated on July 21, 2022Comments
-
Admin almost 2 years
I have created a Temp directory to store some files:
//MARK: -create save delete from directory func createTempDirectoryToStoreFile(){ var error: NSError? let paths = NSSearchPathForDirectoriesInDomains(NSSearchPathDirectory.DocumentDirectory, NSSearchPathDomainMask.UserDomainMask, true) let documentsDirectory: AnyObject = paths[0] tempPath = documentsDirectory.stringByAppendingPathComponent("Temp") if (!NSFileManager.defaultManager().fileExistsAtPath(tempPath!)) { NSFileManager.defaultManager() .createDirectoryAtPath(tempPath!, withIntermediateDirectories: false, attributes: nil, error: &error) } }
It's fine, now I want to delete all the files that are inside the directory... I tried as below:
func clearAllFilesFromTempDirectory(){ var error: NSErrorPointer = nil let dirPath = NSSearchPathForDirectoriesInDomains(.DocumentDirectory, .UserDomainMask, true)[0] as! String var tempDirPath = dirPath.stringByAppendingPathComponent("Temp") var directoryContents: NSArray = fileManager.contentsOfDirectoryAtPath(tempDirPath, error: error)! if error == nil { for path in directoryContents { let fullPath = dirPath.stringByAppendingPathComponent(path as! String) let removeSuccess = fileManager.removeItemAtPath(fullPath, error: nil) } }else{ println("seomthing went worng \(error)") } }
I notice that files are still there... What am I doing wrong?