Detecting some text has been selected in a textbox
Solution 1
To find out selection
if (textbox1.SelectionLength > 0)
{
}
For clipboard content, use
System.Windows.Forms.Clipboard.getText();
Check clipboard content by,
IDataObject iData = Clipboard.GetDataObject();
// Is Data Text?
if (iData.GetDataPresent(DataFormats.Text))
label1.Text = (String)iData.GetData(DataFormats.Text);
else
label1.Text = "Data not found.";
This is implemented in the code. You can use it directly as above
Most important, don't forget
public virtual string SelectedText { get; set; }
This is the complete code with menu item
private void Menu_Copy(System.Object sender, System.EventArgs e)
{
// Ensure that text is selected in the text box.
if(textBox1.SelectionLength > 0)
// Copy the selected text to the Clipboard.
textBox1.Copy();
}
private void Menu_Cut(System.Object sender, System.EventArgs e)
{
// Ensure that text is currently selected in the text box.
if(textBox1.SelectedText.Length > 0)
// Cut the selected text in the control and paste it into the Clipboard.
textBox1.Cut();
}
Private void Menu_Paste(System.Object sender, System.EventArgs e)
{
// Determine if there is any text in the Clipboard to paste into the text box.
if(Clipboard.GetDataObject().GetDataPresent(DataFormats.Text))
{
// Determine if any text is selected in the text box.
if(textBox1.SelectionLength > 0)
{
// Ask user if they want to paste over currently selected text.
if(MessageBox.Show("Do you want to paste over current selection?", "Cut Example", MessageBoxButtons.YesNo) == DialogResult.No)
// Move selection to the point after the current selection and paste.
textBox1.SelectionStart = textBox1.SelectionStart + textBox1.SelectionLength;
}
// Paste current text in Clipboard into text box.
textBox1.Paste();
}
}
private void Menu_Undo(System.Object sender, System.EventArgs e)
{
// Determine if last operation can be undone in text box.
if(textBox1.CanUndo == true)
{
// Undo the last operation.
textBox1.Undo();
// Clear the undo buffer to prevent last action from being redone.
textBox1.ClearUndo();
}
}
Solution 2
Worked for me.
private void button2_Click(object sender, EventArgs e)
{
if (textBox1.SelectedText != String.Empty)
{
label1.Text = textBox1.SelectedText;
}
if (Clipboard.ContainsText())
{
label2.Text = Clipboard.GetText();
}
}
Solution 3
Well, in my opinion the easiest way to go with this is to define enabling/disabling method:
private void editMenuItemOpened(object sender, EventArgs e)
{
//enable copy and cut only if some text is selected
copyMenuItem.Enabled = cutMenuItem.Enabled = textBox1.SelectionLength > 0;
//enable paste only if there's some text in the clipboard to paste
pasteMenuItem.Enabled = Clipboard.ContainsText();
}
and attach it to the editMenuItem.DropDownOpened
event (when using Forms) or editMenuItem.SubmenuOpened
event (when using WPF; You may also want to use RoutedEventArgs
instead of EventArgs
in this case).
Alternatively, if You're using WPF, You could make use of the textBox1.SelectionChanged
event. It's not present in Forms, so in that case You probably should use a combination of textBox1.MouseUp
and textBox1.KeyUp
events.
Solution 4
For the Second half of your question:
textbox1.TextChanged += new TextChangedEventHandler(textbox1_TextChanged);
private void textbox1_TextChanged(object sender, EventArgs e)
{
if (textbox1.Text.Length > 0)
{
// enable delete, copy & paste functions
}
else
{
// disable delete, copy & paste functions
}
}
Related videos on Youtube
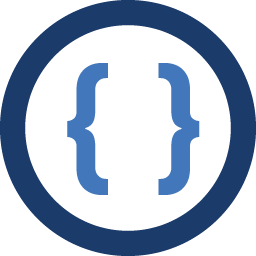
Admin
Updated on September 16, 2022Comments
-
Admin over 1 year
I've implemented a notepad application in c#,all the feaures work perfectly,there is only one thing which I can't implement exactly.there are some menuitems in the edit dropdown menu,but their enabled property must change according to the situation of the textbox,I have a problem with two situations and I'm looking for an event in order to change their enabled property in this event's eventhandler,here is the problem:
2)When some text is selected in the textbox,delete,copy and paste options should get enabled.how should I detect it?I've tested texchanged event an I've written a condition like the code below but it didn't work,just the clipboard works well:
private void textBox1_TextChanged(object sender, EventArgs e) { if (textBox1.SelectionLength> 0) button1.Enabled = false; if (Clipboard.ContainsText()) button2.Enabled = false; }
How should I solve my problem,by the way I have to use a textbox not a richtextbox. Any suggestions will be appreciated. Thanks a lot
-
Admin about 11 yearsI told that when the user selects some text I want to enable copy and paste ...!the code you've written does not match my requirement!
-
Admin about 11 yearsThanks a lot!but
if (textbox1.SelectedText.Length > 0) { }
did not work for me!and would you please tell me where should I write them in textchanged event? -
Admin about 11 yearsI've checked it before!Should I write it somewhere else in my code?
-
Linga about 11 yearsNot necessary. But try this with text_changed event
-
Admin about 11 yearsThanks,but nothing happens when I select a text!check my question again for the code again please
-
Admin about 11 yearsI know your code works well but the problem is that I don't know where to write
if(textBox1.SelectionLength > 0) // Copy the selected text to the Clipboard. textBox1.Copy();
I dont want to have any extra buttons or controls.Thanks. -
Linga about 11 yearsYou should create menu items with popup and shortcut keys and write the code under the menus. If you don't want the menu item then set visible=false. So obviously you can work with shortcut keys
-
Scruffy about 11 yearsOk well I'm sorry that you clarified your question after I had answered. If you want an event to fire after a text selection instead of a text change, then change the TextChangedEventHandler to a RoutedEventHandler: textbox1.SelectionChanged += new RoutedEventHandler(textbox1_SelectionChanged); As for the if statement: if(textbox1.SelectionLength > 0)
-
Avery almost 6 yearsPlease add some context to your answer. Just raw code doesn't explain why this works. An explanation would make your answer more useful