Determining C executable name
Solution 1
Most C compilers provide the -o
option for this, such as:
gcc -o gentext gentext.c
cc -o mainprog -Llib -lmymath firstbit.c secondbit.o
xlc -o coredump coredump.c
Solution 2
-ofilename
will make filename
instead of a.out
.
Solution 3
According to the manual:
-o <file> Place the output into <file>
Solution 4
With the -o
option.
gcc main.c -o myCoolExecutable.o
This is ok if your program consists of a single file. If you have more files I suggest using make
: create a Makefile
and then run the command make
.
A Makefile
is a file containing some rules for compilation.
An example can be the following (#
means the line is a comment):
CXX = gcc
#CXXFLAGS = -std=c++11
#INC_PATH = ...
#LIBS = ...
SOURCEDIR := yourSourceFolder
SOURCES := $(wildcard $(SOURCEDIR)/*.c)
OBJDIR=$(SOURCEDIR)/obj
OBJECTS := $(patsubst $(SOURCEDIR)/%.c,$(OBJDIR)/%.o, $(SOURCES))
DEPENDS := $(patsubst $(SOURCEDIR)/%.c,$(OBJDIR)/%.d, $(SOURCES))
# ADD MORE WARNINGS!
WARNING := -Wall -Wextra
# .PHONY means these rules get executed even if
# files of those names exist.
.PHONY: all clean
# The first rule is the default, ie. "make",
# "make all" and "make parking" mean the same
all: yourExecutableName
clean:
$(RM) $(OBJECTS) $(DEPENDS) yourExecutableName
# Linking the executable from the object files
# $^ # "src.c src.h" (all prerequisites)
yourExecutableName: $(OBJECTS)
$(CXX) $(WARNING) $^ -o $@
#$(CXX) $(WARNING) $(CXXFLAGS) $(INC_PATH) $^ -o $@ $(LIBS)
-include $(DEPENDS)
$(OBJDIR):
mkdir -p $(OBJDIR)
$(OBJDIR)/%.o: $(SOURCEDIR)/%.c Makefile | $(OBJDIR)
$(CXX) $(WARNING) -MMD -MP -c $< -o $@
Shortly CXX
variable defines your compiler (gcc, g++), with CXXFLAGS
you can define flags for your compilation (i.e. -std=c++11
). Then you can include and define custom (INC_PATH
and LIBS
: not set in the example). With SOURCEDIR
you can specify your source code directory (where *.c
files are).Then SOURCES
is basically telling that the source files for the compilation are all the files having extension *.c
.
The Makefile
contains a set of rules whose structure is the following:
output: inputs
commandToExecute
The rule to generate your executable file is
yourExecutableName: $(OBJECTS)
$(CXX) $(WARNING) $^ -o $@
which is equivalent to gcc -Wall -Wextra $(OBJECTS) -o yourExecutableName
.
$(OBJECTS)
are the object file resulting from the compilation. When the above rule is executed, if they are not found make will continue scanning the file to find a rule to generate them. In this case the rule to generate these files is:
$(OBJDIR)/%.o: $(SOURCEDIR)/%.c Makefile | $(OBJDIR)
$(CXX) $(WARNING) -MMD -MP -c $< -o $@
If further information is needed let me know.
Solution 5
If foo
will be your executable and bar.c
is your source file then the command is:
gcc -o foo bar.c
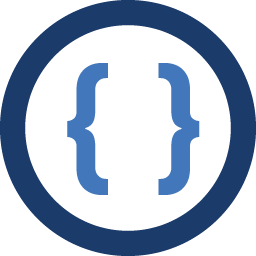
Admin
Updated on May 03, 2021Comments
-
Admin about 3 years
When we are compiling a C program the output is stored in a.out. How can we redirect the compiled output to another file?