Display Array as a Table
Solution 1
Do not remove your foreach loop. Without seeing your code where you build your array, my guess would be you are doing this:
$res = array('id' => 1, 'first_name'=>'mike', 'last_name'=>'lastname');
when in fact what you probably want to be doing is this:
$res[] = array('id' => 1, 'first_name'=>'mike', 'last_name'=>'lastname');
the brackets [ ] add an array of all the values to your array. This way you can loop through each as a collection of values, not individual values.
Solution 2
<? Php
echo "<table border="2">";
foreach($ array as $ values)
{
echo "<tr><td>";
echo "$ values";
echo "</td></tr>";
}
?>
I wrote this for printing single array values into a table
Solution 3
You data structure is not matrix but an array:
Array ( [id] => 1 [first_name] => mike [last_name] => lastname )
You need something like this to do what you want:
Array(
[0] => Array ( [id] => 1 [first_name] => mike [last_name] => lastname ),
[1] => Array ( [id] => 1 [first_name] => mike [last_name] => lastname )
........
);
Apart from that, code seems fine...
Solution 4
This is how I solved a similar problem I had with my webpage. I wanted the page to display an array of all classes a specific teacher teaches using session variables. I hope that, even though the subject might not be relevant to you, the general gist will help people out.
<?php
echo "<table border='1' style='border-collapse:
collapse;border-color: silver;'>";
echo "<tr style='font-weight: bold;'>";
echo "<td width='150' align='center'>Class</td>";
echo "</tr>";
foreach ($query_class_array as $row)
{
echo '<td width="150" align=center>' . $row['Class'] . '</td>';
echo '</tr>';
}
?>
Solution 5
If the array is of std object type, you can't use [], instead use -> to get specific entities
<!DOCTYPE html>
<html>
<body>
<table>
<tr>
<th>id</th>
<th>First name</th>
<th>Last name</th>
<th>email</th>
</tr>
<?php
foreach($data as $item) {?>
<tr>
<td><?php echo $item->id; ?></td>
<td><?php echo $item->first_name; ?></td>
<td><?php echo $item->last_name ;?></td>
<td><?php echo $item->email;?></td>
</tr>
<?php }?>
</table>
</body>
</html>
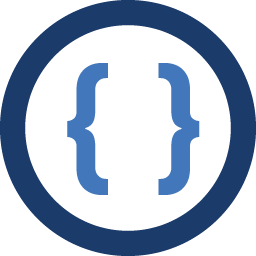
Admin
Updated on February 11, 2022Comments
-
Admin over 2 years
I am trying to print a table using PHP/HTML. Data stored inside array like this:
Array ( [id] => 1 [first_name] => mike [last_name] => lastname )
My code is as follow. It runs and there are no error however the output is not as expected. Here is the PHP/HTML code:
<table> <tr> <th>1</th> <th>2</th> <th>3</th> </tr> <?php foreach ($res as $item): ?> <tr> <td><?php echo $item['id'] ?></td> <td><?php echo $item['first_name'] ?></td> <td><?php echo $item['last_name'] ?></td> </tr> <?php endforeach; ?> </table>
The result I get is the first character of the items:
1 2 3 1 1 1 m m m l l l
Not sure what I am doing wrong? I would really appreciate an explanation.
UPDATE:
PHP CODE that has no errors:
<?php foreach ($result as $row) { echo '<tr>'; echo '<td>' . $row['id'] . '</td>'; echo '<td>' . $row['first_name'] . '</td>'; echo '<td>' . $row['last_name'] . '</td>'; echo '</tr>'; } ?>
And this is my array with only one "row" in it:
Output of $result variable using print_r($result) wrapped with < PRE > tags
Array ( [id] => 3 [first_name] => Jim [last_name] => Dude )
Here is the result I am getting:
Actual Table result:
ID First Name Last Name 3 3 3 J J J D D D
However if I have 0 in array or more than 1 (meaning 2 or more) it works perfectly. IT JUST DOES NOT WORK WHEN I HAVE ONLY ONE "ROW" OF ELEMENTS INSIDE ARRAY. For example this array, works perfectly:
Array ( [0] => Array ( [id] => 3 [first_name] => Jim [last_name] => Dude ) [1] => Array ( [id] => 4 [first_name] => John [last_name] => Dude2 ) )
I get this result:
ID First Name Last Name 3 Jim Dude 4 John Dude2
I am not really sure what I am doing wrong. The idea is not knowing how many items is in a Table read it into
$result
variable and then using this variable print all elements inside HTML table. Table could contain O elements, 1 row of elements, 1 or more rows of elements.