Does every Python function have to return at the end?
Solution 1
So I was making a diagram of the recursive function to wrap my head around recursion, and I noticed that apparently every function executes return at the end?
A return itself simply means that the call frame is popped from the call stack, the program counter is set back to state where it was before you made the call, etc. Based on your comment it looks a bit like "backtracking" but mind that - in contrast to backtracking - side effects the functions have, are not reverted/undone. For instance appending to a list, drawing a line, writing to a file, etc. are not reverted. This is the desired behavior in recursion.
Now sometimes it is useful to return a certain value. For instance:
def foo(x,y):
return x+y
here foo
does not only go back to the caller, but first evaluates x+y
and the result is returned, such that you can state z = foo(2,3)
. So here foo
is called, 2+3
is evaluated and 5
is returned and assigned to z
.
Another question, what exactly does a function return? All of parameters passed to it (assuming multiple parameters)?
In Python all functions return a value. If you specify it like:
return <expression>
the <expression>
part is evaluated and that value is returned. If you specify:
return
or no return
at all (but you reach the end of the function), None
will be returned.
Solution 2
Quick answer: No
Long answer: The return
statement is not required in Python, and reaching the end of the function's code without a return will make the function automatically return None
Additional question: What does a function return?
It returns the objects provided to the return
statement if found one. If no return
statement is found, None
is returned.
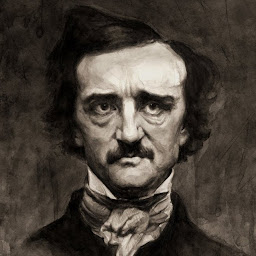
Max
Updated on June 04, 2022Comments
-
Max almost 2 years
So I was making a diagram of the recursive function to wrap my head around recursion, and I noticed that apparently every function executes return at the end?
Another question, what exactly does a function return? All parameters passed to it (assuming multiple parameters)? Or some kind of value?
(t is just an entity that performs actual drawing)
def koch(t, n): """Draws a koch curve with length n.""" if n<30: fd(t, n) return m = n/3.0 koch(t, m) lt(t, 60) koch(t, m) rt(t, 120) koch(t, m) lt(t, 60) koch(t, m)