EF Code First Parent-Child insertions with identity columns
Solution 1
You shouldn't worry about what value the Id of Parent
will get in order to insert Child
rows. This should be enough:
var parent = new Parent
{
// fill other properties
Children = new List<Child>()
}
parent.Children.add(new Child { /*fill values */);
dbContext.Parents.Add(parent); // whatever your context is named
dbContext.SaveChanges();
For the record, the ID's will be assigned after calling SaveChanges()
, so if you really need the ID before inserting a Child
entity you can always call SaveChanges()
twice.
Again, this shouldn't be necessary though.
By the way, I would recommend making the Foreign Key property from Child
to Parent
a navigation property, so the Child
class would look like:
class Child
{
public int ChildId { get; set; } // identity column
public string ChildName { get; set; }
public virtual Parent Parent { get ; set; } //foreign key to Parent
}
That way you can always access the Child's parent directly without having to retrieve it explicitly from the database yourself (it will be lazy loaded).
Solution 2
For an example of how to do this, see the new EF Code First MVC tutorial series. The 6th one in the series has an example of this. The first one in the series of 10 is here: http://www.asp.net/entity-framework/tutorials/creating-an-entity-framework-data-model-for-an-asp-net-mvc-application
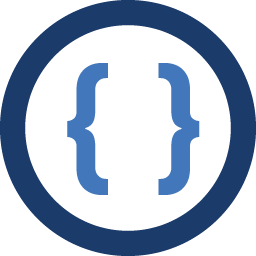
Admin
Updated on August 26, 2020Comments
-
Admin over 3 years
i have the following model.
class Parent { int ParentId (identity column) { get; set; } string ParentName { get; set; } virtual ICollection<Child> Children { get; set; } } class Child { int ChildId (identity column) { get; set; } string ChildName { get; set; } int ParentID { get ; set; } //foreign key to Parent(ParentID) }
How do i insert few rows to my parent and child in single transaction? Basically i want to get the identity generated on the parent(say i insert a row in parent) and insert child rows with that value? How this can be achieved using Code First?