ElementClickInterceptedException: Message: element click intercepted Element is not clickable error clicking a radio button using Selenium and Python
This error message...
selenium.common.exceptions.ElementClickInterceptedException: Message: element click intercepted:
Element <input type="radio" name="docTypes" ng-model="$ctrl.documentTypes.selected" id="documentType-0" ng-change="$ctrl.onChangeDocumentType()" ng-value="documentType" tabindex="0" class="ng-pristine ng-untouched ng-valid ng-empty" value="[object Object]" aria-invalid="false">
is not clickable at point (338, 202).
Other element would receive the click:
<label translate-attr="{title: 'fulfillment.documentAction.createNew.modal.documentType.document.title'}" translate-values="{documentName: documentType.name}" for="documentType-0" translate="ASN - DSD" tabindex="0" title="Select ASN - DSD document type">...</label>
...implies that the desired element wasn't clickable as some other element obscures it.
The desired element is a Angular element so to invoke click()
on the element you have to induce WebDriverWait for the element_to_be_clickable()
and you can use either of the following Locator Strategies:
-
Using
CSS_SELECTOR
:WebDriverWait(driver, 20).until(EC.element_to_be_clickable((By.CSS_SELECTOR, "label[for='documentType-0']"))).click()
-
Using
XPATH
:WebDriverWait(driver, 20).until(EC.element_to_be_clickable((By.XPATH, "//label[@for='documentType-0']"))).click()
-
Note : You have to add the following imports :
from selenium.webdriver.support.ui import WebDriverWait from selenium.webdriver.common.by import By from selenium.webdriver.support import expected_conditions as EC
Update
As an alternative you can use the execute_script()
method as follows:
-
Using
CSS_SELECTOR
:driver.execute_script("arguments[0].click();", WebDriverWait(driver, 20).until(EC.element_to_be_clickable((By.CSS_SELECTOR, "label[for='documentType-0']"))))
-
Using
XPATH
:driver.execute_script("arguments[0].click();", WebDriverWait(driver, 20).until(EC.element_to_be_clickable((By.XPATH, "//label[@for='documentType-0']"))))
References
You can find a couple of relevant discussions in:
- Element MyElement is not clickable at point (x, y)… Other element would receive the click
- Selenium Web Driver & Java. Element is not clickable at point (x, y). Other element would receive the click
- ElementClickInterceptedException: Message: element click intercepted: Element is not clickable with Selenium and Python
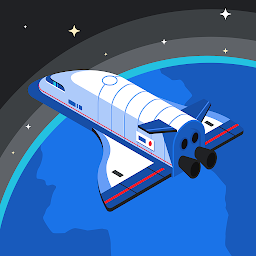
Ryan Bobber
Updated on July 22, 2022Comments
-
Ryan Bobber almost 2 years
I am trying to click on the first box (ASN / DSD)
But I get this error message:
selenium.common.exceptions.ElementClickInterceptedException: Message: element click intercepted: Element <input type="radio" name="docTypes" ng-model="$ctrl.documentTypes.selected" id="documentType-0" ng-change="$ctrl.onChangeDocumentType()" ng-value="documentType" tabindex="0" class="ng-pristine ng-untouched ng-valid ng-empty" value="[object Object]" aria-invalid="false"> is not clickable at point (338, 202). Other element would receive the click: <label translate-attr="{title: 'fulfillment.documentAction.createNew.modal.documentType.document.title'}" translate-values="{documentName: documentType.name}" for="documentType-0" translate="ASN - DSD" tabindex="0" title="Select ASN - DSD document type">...</label> (Session info: chrome=83.0.4103.116)
I know I have entered the right iframe because it can find the element, just not click on it. My code is
driver.switch_to.default_content() iframes = driver.find_elements_by_tag_name("iframe") driver.switch_to.frame(iframes[0]) time.sleep(5) driver.find_element_by_xpath('//*[@id="documentType-0"]').click()
I saw that DebanjanB answered a similar question on here: link
I am trying to do his third solution of using execute script. I don't know what CSS selector to use for this model. The model looks like this
WebDriverWait(driver, 20).until(EC.invisibility_of_element((By.CSS_SELECTOR, "span.taLnk.ulBlueLinks"))) driver.execute_script("arguments[0].click();", WebDriverWait(driver, 20).until(EC.element_to_be_clickable((By.XPATH, "//div[@class='loadingWhiteBox']"))))
My question is what css selector do I need to use on the first line, and then is it just initial xpath I was using on the second line?
Here is the HTML for reference. I get the click intercept error when I try to click on the input section. If use xpath to click on the label tag, it does not error out but also does not click on it. It just moves on to the next section of code without doing anything.
<li ng-repeat="documentType in selectDocumentType.documentTypes.displayedList | orderBy:selectDocumentType.formOrder"> <input type="radio" name="docTypes" ng model="selectDocumentType.documentTypes.selected" id="documentType-0" ng-value="documentType" tabindex="0" class="ng-valid ng-not-empty ng-dirty ng-valid-parse ng-touched" value="[object Object]" aria-invalid="false"> <label translate-attr="{title:'fulfillment.documentAction.createNew.modal.documentType.document.title'}" translate-values={documentName: documentType.name}" for="documentType-0" translate="ASN - DSD" tabindex="0" title= "Select ASN - DSD document type"><span>ASN - DSD</span></label> </li>
Any suggestions on how to stop having the click intercepted?