Error - already defines a member called 'InitializeComponent' with the same parameter types
Solution 1
WPF Window
class created in Visual Studio usually has InitializeComponent
method that is used to initialize its properties and contents - What does InitializeComponent() do, and how does it work in WPF?.
It is generated from your XAML markup and is not contained in your code-behind .cs file, but for the compiler(and msbuild.exe) it is still a valid intrinsic part of the Window class - if you create new empty Window and click on InitializeComponent()
call then a *.g.i.cs
temporary file with initialization code will be opened.
So, when you put another InitializeComponent
method into the code behind file it causes ambiguous method definition.
SOLUTION:
Either rename your custom method to InitializeComponentsCustom
and call it in the constructor:
public MainWindow()
{
InitializeComponent();
InitializeComponentsCustom();
}
private void InitializeComponentsCustom()
{
// ...
}
or just put the entire code from book method into the constructor(just do not remove the original InitializeComponent
call).
Solution 2
InitializeComponent
method is generated automatically in MainWindow.g.cs when you define MainWindow.xaml.
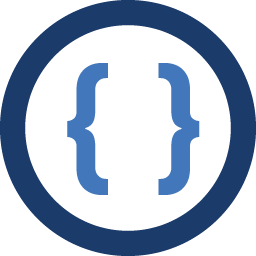
Admin
Updated on June 05, 2022Comments
-
Admin almost 2 years
I tried to make an example of the book that shows exactly
private Button button1; public MainWindow() { InitializeComponent(); } private void InitializeComponent() { // Configure the form. this.Width = this.Height = 285; this.Left = this.Top = 100; this.Title = "Code-Only Window"; // Create a container to hold a button. DockPanel panel = new DockPanel(); // Create the button. button1 = new Button(); button1.Content = "Please click me."; button1.Margin = new Thickness(30); // Attach the event handler. button1.Click += button1_Click; // Place the button in the panel. IAddChild container = panel; container.AddChild(button1); // Place the panel in the form. container = this; container.AddChild(panel); } private void button1_Click(object sender, RoutedEventArgs e) { button1.Content = "Thank you."; }
But it gives me an error:
"Type 'WpfApplication1.MainWindow' already defines a member called 'InitializeComponent' with the same parameter types"