Error: declaration of C function conflicts with previous declaration
Solution 1
You have two main
functions. You should only have one main
function.
Solution 2
Besides the fact you have two main
functions,
I think you're misunderstanding how multiple files are used in C++. One .cpp file shouldn't see the contents of another .cpp during compilation.
You can define a function (or class, etc) in a .cpp file, however you also have to declare this in a .h (or .hpp/.hh) file which you then use to reference in another file.
For example if you had a function called int Test()
in a file called Utils.cpp. To use this in your main.cpp
file you will need to create a file called something along the lines of Utils.h
(The actual name is irrelevent but you want something that is understandable). Inside Utils.h
you should put something like:
#pragma once
int Test();
This file simply tells the compiler that a function called Test is declared somewhere in my program, accept the fact it exists and move the compilation along (it is the linker's job, not the compilers, to sort out these issues).
Then in your main.cpp file, include Utils.h
. Whilst you can include .cpp files it's generally considered a bad idea since you end up increasing compile times, object file sizes and you may encounter linker issues if something is parsed twice (I'm not so sure on the last point)
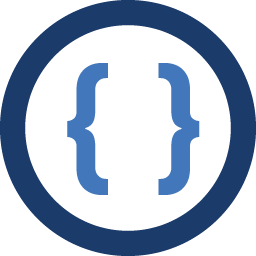
Admin
Updated on June 14, 2022Comments
-
Admin almost 2 years
I'm a bit of a beginner and I'm starting to get the hang of things but I'm adding another part to this calculator for this guy.
I have this as the
main.cpp
, I have added the bit forselection == 5
:#include <iostream> #include <string.h> using namespace std; // Function includes // I try to keep them in the order they appear in the // output below for organization purposes #include "calc.m.xy12plugin.cpp" #include "calc.b.xymplugin.cpp" #include "calc.m.xybplugin.cpp" #include "calc.point.xymplugin.cpp" #include "calc.parallelplugin.cpp" // The above one would be here, too int main(int argc, const char* argv[]) { int i; i = 0; cout << "Linear Equation Calculator" << endl << "Copyright (c) 2011 Patrick Devaney" << endl << "Licensed under the Apache License Version 2" << endl; // This loop makes the code a bit messy, // but it's worth it so the program doesn't // crash if one enters random crap such as // "zrgxvd" or "54336564358" while(i < 1) { cout << "Type:" << endl << "0 to calculate a slope (the M value) based on two points on a line" << endl << "1 to calculate the Y-intercept (the B value) based on two points and a slope" << endl << "2 to calculate the slope (the M value) based on the Y-intercept and X and Y" << endl << "plug-ins" << endl << "3 to find the next point up or down a line based on the slope (M) and X and Y" << endl << "plug-ins" << endl << "4 to find a point x positions down the line based on the slope (M) and X and Y" << endl << "plug-ins" << endl << "5 to find the equation of a parallel line in form y=mx+c" << endl << "plug-ins" << endl; string selection; cin >> selection; if(selection == "0") { mcalcxyplugin(); i++; } else if(selection == "1") { calcbxymplugin(); i++; } else if(selection == "2") { calcmxybplugin(); i++; } else if(selection == "3") { calcpointxymplugin(1); i++; } else if(selection == "4") { int a; cout << "How many points up/down the line do you want? (Positive number for points" << endl << "further up, negative for previous points" << endl; cin >> a; calcpointxymplugin(a); i++; } else if(selection == "5"){ calcparallelplugin(); i++; } else { i = 0; } // End of that loop below } return 0; }
I then created this file that i linked in to the
else if(selection == "5"...
This is the
cal.parallelplugin.cpp
file#include <iostream> using namespace std; int main(){ cout <<"Welcome to the Parallel Line Calculator \n" << endl; cout << "Here you will find the equation of the line parallel to a line passing through \na point (x,y) in the form y=mx+c \n\n" << endl; float x,y, c, x1, y1, gradient, c1; cout << "NOTE: Equation must be in form y=mx+c \n" << endl; cout <<"Please enter the number of Xs:" <<endl; cin >> x; cout <<"Please enter the number of Ys:" <<endl; cin >> y; cout <<"Please enter the number of Cs:" <<endl; cin >> c; cout <<"Please enter the x co-ordinate:" <<endl; cin >> x1; cout <<"Please enter the y co-ordinate:" <<endl; cin >> y1; gradient= x/y; c1 = y1 + (gradient*x1); cout << "Equation of parallel line through (" << x1 << ", " << y1 << ") is " << "y=" << gradient << "x+" << c1 << endl; }
I don't get errors when compiling this but when I compile the main.cpp I get the following errors and I can't spend my all life working out what is wrong :(
C:\Users\George\Desktop\linear_equation_calc\main.cpp||In function 'int main(int, const char**)':| C:\Users\George\Desktop\linear_equation_calc\main.cpp|51|error: declaration of C function 'int main(int, const char**)' conflicts with| C:\Users\George\Desktop\linear_equation_calc\calc.parallelplugin.cpp|9|error: previous declaration 'int main()' here| C:\Users\George\Desktop\linear_equation_calc\main.cpp||In function 'int main(int, const char**)':| C:\Users\George\Desktop\linear_equation_calc\main.cpp|100|error: 'calcparallelplugin' was not declared in this scope| ||=== Build finished: 3 errors, 0 warnings ===|
HELP!
-
Oliver Charlesworth about 12 years@georgeherby: Also, you shouldn't be
#include
-ing .cpp files; in general, you should only#include
header files.