ERROR org.apache.velocity : ResourceManager : unable to find resource 'xxx.html.vm' in any resource loader
Solution 1
Add the following row to application.properties
:
spring.velocity.view-names=xxx,yyy,zzz
Solution 2
This happens because spring boot configures various ViewResolvers based on what is available in classpath If velocity dependency is found in classpath, then spring would configure a VelocityViewResolver, but along with that it configures other view resolvers too, ContentNegotiatingViewResolver being one of them.
ContentNegotiatingViewResolver tries to match the view name and MIME type to determine the best possible view automatically. In this process it tries to find the XXX.vm.html and hence throws the exception.
To fix this configure your view resolvers manually. Refer: http://docs.spring.io/spring-boot/docs/current/reference/html/howto-spring-mvc.html#howto-switch-off-default-mvc-configuration
I did configure my viewResolvers manually by introducing following class, and the problem disappeared.
@Configuration
@EnableWebMvc
public class MvcConfiguration extends WebMvcConfigurerAdapter{
@Autowired
private final ResourceLoader resourceLoader = new DefaultResourceLoader();
@Bean
public VelocityConfig velocityConfig() {
VelocityConfigurer cfg = new VelocityConfigurer();
cfg.setResourceLoader(resourceLoader);
cfg.setResourceLoaderPath("classpath:/templates/")
return cfg;
}
@Bean
public ViewResolver viewResolver() {
VelocityViewResolver resolver = new VelocityViewResolver();
resolver.setViewClass(VelocityToolboxView.class);
resolver.setPrefix("");
resolver.setSuffix(".vm");
return resolver;
}
@Override
public void addResourceHandlers(ResourceHandlerRegistry registry) {
final String[] CLASSPATH_RESOURCE_LOCATIONS = [
"classpath:/META-INF/resources/", "classpath:/resources/",
"classpath:/static/", "classpath:/public/" ];
registry.addResourceHandler("/**").addResourceLocations(
CLASSPATH_RESOURCE_LOCATIONS);
}
}
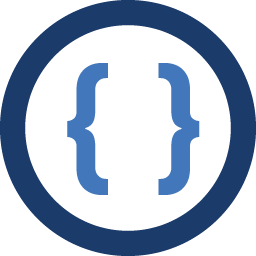
Admin
Updated on July 29, 2022Comments
-
Admin almost 2 years
I'm using Velocity templates along with Spring boot.
When there is a file named 'xxx.vm' in templates directory, Spring Boot successfully loads 'xxx.vm'. But an ERROR message below is logged.
"ERROR org.apache.velocity : ResourceManager : unable to find resource 'xxx.html.vm' in any resource loader."
I don't understand why the system looks for 'xxx.html.vm' because the suffix in the application.properties is set to ".vm"
Here is configuration in application.properties
spring.velocity.enabled=true spring.velocity.resource-loader-path=classpath:/templates/ spring.velocity.suffix=.vm
There is no problem with running my application, but I'd like to know what causes this Error message. Could you please help me deal with this? Thank you in advance.