event is not defined in mozilla firefox for javascript function?
Solution 1
In FF/Mozilla the event is passed to your event handler as a parameter. Use something like the following to get around the missing event argument in IE.
function onlyNumeric(e)
{
if (!e) {
e = window.event;
}
...
}
You'll find that there are some other differences between the two as well. This link has some information on how to detect which key is pressed in a cross-browser way.
Solution 2
Or quite simply, name the parameter event and it will work in all browsers. Here is a jQueryish example:
$('#' + _aYearBindFlds[i]).on('keyup', function(event) {
if(! ignoreKey({szKeyCodeList: gvk_kcToIgnore, nKeyCode: event.keyCode })) this.value = this.value.replace(/\D/g, '');
});
This example allows digits only to be entered for year fields (inside a for each loop selector) where ingoreKey() takes a keyCode list/array and compares the event keyCode and determines if it should be ignored before firing the bind event.
Keys I typically ingore for masks/other are arrow, backspace, tabs, depending on context/desired behaviour.
You can also typically use event.which instead of event.keyCode in most browsers, at least when you are using jQuery which depends on event.which to normalize the key and mouse events.
I don't know for sure what happens under the covers in the js engines, but it seems Mozilla FF respects a stricter scope, wherein, other browsers may be automatically addressing the window.event.keyCode scope on their own when the event is not explicitly passed in to a function or closure.
In FF, you can also address the event by window.event (as shown in some examples here) which would support this thought.
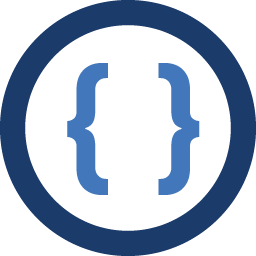
Admin
Updated on October 31, 2020Comments
-
Admin over 3 years
function onlyNumeric() { if (event.keyCode < 48 || event.keyCode > 57) { event.returnValue = false; } } onkeypress=onlyNumneric();
In IE, this code is working fine. However, in Mozilla Firefox, the event is an undefined error.