Find Missing items from arraylist in java
Solution 1
You can use boolean removeAll(Collection<?> c)
method.
Just note that this method modifies the List
on which you invoke it. In case you to keep the original List
intact then you would have to make a copy of the list first and then the method on the copy.
e.g.
List<Employee> additionalDataInListA = new ArrayList<Employee>(listA);
additionalDataInListA.removeAll(listB);
Solution 2
Override equals
in Employee
to test for equality between ids (and remember that when you override equals
you should also override hashCode
). Then:
List<Employee> additionalDataInListA = new ArrayList<Employee>(listA);
additionalDataInListA.removeAll(listB);
List<Employee> additionalDataInListB = new ArrayList<Employee>(listB);
additionalDataInListB.removeAll(listA);
Relevant documentation:
Solution 3
You can use lambdaj (download here, website) and hamcrest (download here, website), this libraries are very powerfull for managing collections, the following code is very simple and works perfectly. With this libraries you can solve your problem in one line. You must add to your project: hamcrest-all-1.3.jar and lambdaj-2.4.jar Hope this help serve.
import static ch.lambdaj.Lambda.filter;
import static ch.lambdaj.Lambda.having;
import static ch.lambdaj.Lambda.on;
import java.util.Arrays;
import java.util.List;
import static org.hamcrest.Matchers.isIn;
import static org.hamcrest.Matchers.not;
public class Test2{
public static void main(String[] args) {
List<String> oldNames = Arrays.asList("101","102","103","104","105");
List<String> newNames = Arrays.asList("101","102","106","107","108");
List<String> newList = filter(not(having(on(String.class), isIn(oldNames))),newNames);
List<String> newList2 = filter(not(having(on(String.class), isIn(newNames))),oldNames);
System.out.println(newList);
System.out.println(newList2);
/*out
[106, 107, 108]
[103, 104, 105]
*/
}
}
This example work with a simple List of Strings but can can be adapted for your object Employee.
Solution 4
You can use the Collection
interface's removeAll
method as shown in the top answer to this question (How can I calculate the difference between two ArrayLists?).
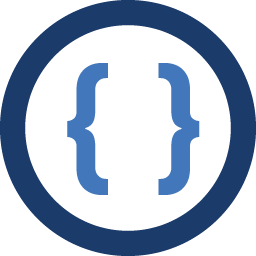
Admin
Updated on June 23, 2022Comments
-
Admin almost 2 years
Hi I am newbie to java and was trying my hand on collections part, I have a simple query I have two array list of say employee object
class Employee { private int emp_id; private String name; private List<String> mobile_numbers; //.....getters and setters }
say
listA
andlistB
have the following dataList<Employee> listA = new ArrayList<Employee>(); List<Employee> listB = new ArrayList<Employee>(); listA.add(new Employee("101", "E1", listOfMobileNumbers)); listA.add(new Employee("102", "E2", listOfMobileNumbers1)); listA.add(new Employee("103", "E3", listOfMobileNumbers2)); listA.add(new Employee("104", "E4", listOfMobileNumber4)); listA.add(new Employee("105", "E5", listOfMobileNumbers5)); listB.add(new Employee("101", "E1", listOfMobileNumbers1)); listB.add(new Employee("102", "E2", listOfMobileNumbers2)); listB.add(new Employee("106", "E6", listOfMobileNumber6)); listB.add(new Employee("107", "E7", listOfMobileNumber7)); listB.add(new Employee("108", "E8", listOfMobileNumbers8));
where
listOfMobileNumbers
is aList<String>
Now I want to find the additional elements from the individuals list. i.e
List<Employee> additionalDataInListA = new ArrayList<Employee>(); // this list should contain 103, 104 and 105 List<Employee> additionalDataInListB= new ArrayList<Employee>(); // this list should contain 106, 107 and 108
How do i achieve this?
Your help is appreciated.
Edit:
I don't want to use any internal functions i want to achieve it writing some manual function kind of comparison function.
I also cant override the equals function because the in my usecase i have FieldNo which is int and Values which is a
List<String>
.the occurrences of field is 'n' times and every time the Values associated with that field will different.
For example : FieldNo=18 Values=["A"];
FieldNo=18 Values=["B"]; and so on...
The employee id that I had used was for only illustration purposes where it makes sense to override equals and hash code.