Firebase Expandable Notification Show image when app is in background
Solution 1
FCM notification messages
don't support the largeIcon or bigPicture.
if you need them while in background you can use a FCM data message
.
For data messages the onMessageReceived(message)
method is always called, so you can use the message.getData()
method and create your custom notification.
Read more about notification messages vs data messages here: https://firebase.google.com/docs/cloud-messaging/concept-options#notifications_and_data_messages
Solution 2
See my FirebaseMessagingService
public class MyFirebaseMessagingService extends FirebaseMessagingService {
private static final String TAG = "FirebaseMessageService";
Bitmap bitmap;
/**
* Called when message is received.
*
* @param remoteMessage Object representing the message received from Firebase Cloud Messaging.
*/
@Override
public void onMessageReceived(RemoteMessage remoteMessage) {
// There are two types of messages data messages and notification messages. Data messages are handled
// here in onMessageReceived whether the app is in the foreground or background. Data messages are the type
// traditionally used with GCM. Notification messages are only received here in onMessageReceived when the app
// is in the foreground. When the app is in the background an automatically generated notification is displayed.
// When the user taps on the notification they are returned to the app. Messages containing both notification
// and data payloads are treated as notification messages. The Firebase console always sends notification
// messages. For more see: https://firebase.google.com/docs/cloud-messaging/concept-options
//
Log.d(TAG, "From: " + remoteMessage.getFrom());
// Check if message contains a data payload.
if (remoteMessage.getData().size() > 0) {
Log.d(TAG, "Message data payload: " + remoteMessage.getData());
}
// Check if message contains a notification payload.
if (remoteMessage.getNotification() != null) {
Log.d(TAG, "Message Notification Body: " + remoteMessage.getNotification().getBody());
}
//The message which i send will have keys named [message, image, AnotherActivity] and corresponding values.
//You can change as per the requirement.
//message will contain the Push Message
String message = remoteMessage.getData().get("message");
//imageUri will contain URL of the image to be displayed with Notification
String imageUri = remoteMessage.getData().get("image");
//If the key AnotherActivity has value as True then when the user taps on notification, in the app AnotherActivity will be opened.
//If the key AnotherActivity has value as False then when the user taps on notification, in the app MainActivity will be opened.
String TrueOrFlase = remoteMessage.getData().get("AnotherActivity");
//To get a Bitmap image from the URL received
bitmap = getBitmapfromUrl(imageUri);
sendNotification(message, bitmap, TrueOrFlase);
}
/**
* Create and show a simple notification containing the received FCM message.
*/
private void sendNotification(String messageBody, Bitmap image, String TrueOrFalse) {
Intent intent = new Intent(this, MainActivity.class);
intent.addFlags(Intent.FLAG_ACTIVITY_CLEAR_TOP);
intent.putExtra("AnotherActivity", TrueOrFalse);
PendingIntent pendingIntent = PendingIntent.getActivity(this, 0 /* Request code */, intent,
PendingIntent.FLAG_ONE_SHOT);
Uri defaultSoundUri = RingtoneManager.getDefaultUri(RingtoneManager.TYPE_NOTIFICATION);
NotificationCompat.Builder notificationBuilder = new NotificationCompat.Builder(this)
.setLargeIcon(image)/*Notification icon image*/
.setSmallIcon(R.drawable.firebase_icon)
.setContentTitle(messageBody)
.setStyle(new NotificationCompat.BigPictureStyle()
.bigPicture(image))/*Notification with Image*/
.setAutoCancel(true)
.setSound(defaultSoundUri)
.setContentIntent(pendingIntent);
NotificationManager notificationManager =
(NotificationManager) getSystemService(Context.NOTIFICATION_SERVICE);
notificationManager.notify(0 /* ID of notification */, notificationBuilder.build());
}
/*
*To get a Bitmap image from the URL received
* */
public Bitmap getBitmapfromUrl(String imageUrl) {
try {
URL url = new URL(imageUrl);
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
connection.setDoInput(true);
connection.connect();
InputStream input = connection.getInputStream();
Bitmap bitmap = BitmapFactory.decodeStream(input);
return bitmap;
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
return null;
}
}
}
Solution 3
In case some lands here in 2019, you can simply add an image field to the notification object:
{
notification: {
title: title,
body: body,
image: "http://path_to_image"
},
data: {
click_action: "FLUTTER_NOTIFICATION_CLICK",
your_data: ...,
},
token: token
}
I have tested it using Flutter on Android and I would assume it works on native Android since they both probably use the same native SDK.
Solution 4
If your problem is related to showing Big Image i.e. if you are sending push notification with an image from firebase console and it displays the image only if the app in the foreground. The solution for this problem is to send a push message with only data field.
{ "data": { "image": "https://static.pexels.com/photos/4825/red-love-romantic-flowers.jpg", "message": "Firebase Push Message Using API" "AnotherActivity": "True" }, "to" : "device id Or Device token" }
This definitely solves the problem.
Solution 5
Messages, that contain both notification and data payload (like your example sent with Postman) are automatically displayed to end-user devices by the FCM library. And this does not include (big) images.
I guess there are two possibilities for you:
Try what Rashmi Jain suggested. However, this solution could work right now and stop working tomorrow, if the Firebase library is updated (and thus the implementation of the message handling)
Send a data message with Postman. You may not fill the notification object in the JSON therefore, so it could look something like this:
{ "message": { "token":"bk3RNwTe3H0:CI2k_HHwgIpoDKCIZvvDMExUdFQ3P1...", "data":{ "title" : "Awesome title", "body" : "Your awesome push notification body", "image" : "your_image_url" } } }
I would prefer the 2nd option. Good luck!
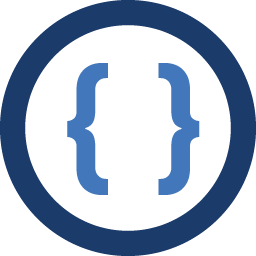
Admin
Updated on February 02, 2022Comments
-
Admin over 2 years
I am implementing FCM notifications in Android, but how does notifications differ depending on the app status (background vs. foreground)?
I am sending the notification using the FCM API with Postman and this is the notification structure:
{ "notification": { "title": "Notification title", "body": "Notification message", "sound": "default", "color": "#53c4bc", "click_action": "MY_BOOK", "icon": "ic_launcher" }, "data": { "main_picture": "URL_OF_THE_IMAGE" }, "to" : "USER_FCM_TOKEN" }
The image to render is taken from
data.main_picture
.I have implemented my own
FirebaseMessagingService
which makes the notifications display perfectly in foreground state. The notification code is the next:NotificationCompat.BigPictureStyle notiStyle = new NotificationCompat.BigPictureStyle(); notiStyle.setSummaryText(messageBody); notiStyle.bigPicture(picture); Uri defaultSoundUri= RingtoneManager.getDefaultUri(RingtoneManager.TYPE_NOTIFICATION); NotificationCompat.Builder notificationBuilder = (NotificationCompat.Builder) new NotificationCompat.Builder(this) .setSmallIcon(R.drawable.ic_launcher) .setLargeIcon(bigIcon) .setContentTitle(title) .setContentText(messageBody) .setAutoCancel(true) .setSound(defaultSoundUri) .setContentIntent(pendingIntent) .setStyle(notiStyle); code here NotificationManager notificationManager = (NotificationManager) getSystemService(Context.NOTIFICATION_SERVICE); notificationManager.notify(0, notificationBuilder.build());
However, in background, the service is not even executed. In the
AndroidManifest.xml
, Firebase services are declared as follow:<service android:name=".MyFirebaseMessagingService"> <intent-filter> <action android:name="com.google.firebase.MESSAGING_EVENT"/> </intent-filter> </service> <service android:name=".MyFirebaseInstanceIDService"> <intent-filter> <action android:name="com.google.firebase.INSTANCE_ID_EVENT"/> </intent-filter> </service>
My problem is not the
LargeIcon
orSmallIcon
but displaying the big picture.Thanks for your support.
-
Anirudh over 7 yearsonMessageReceived(message) is called only when the app is in foreground.
-
Parth Anjaria over 7 yearsonMessageReceived(message) is not called in background
-
Mayura Devani over 7 yearsonMessageReceived(message) is called only when the app is in foreground. Then how will sending only data field will work when app is in background?
-
Arun over 7 years@MayuraDevani I have not read the internal files of firebase, but i have tested it, it works if sent with only data field, even customised intents working fine.
-
Mayura Devani over 7 yearsIn my case, If I use only data field, then i get push message only when application is in foreground, but not when app in background or killed.
-
Arun over 7 years@MayuraDevani You need to change the code in FirebaseMessagingService, there would be a check for app in foreground, remove that. Same code will be executed for all the scenarios. BTW was notification coming for sending notification with firebase console ?
-
Mayura Devani over 7 yearsYes, Notifiation is coming when sending from firebase console. Hey, Is your code working when app is killed? I mean if you force stop your application, and then send notification, Is it working ?
-
Arun over 7 yearsYes it is working, notification is coming even when we close the app from task manager.
-
Mayura Devani over 7 yearsOhh, In my case it is not working. What can be the problem? I am sending only data message from server, and i am simply displaying notification in method onMessageReceived in FirebaseMessagingService without check for app in foreground or background. I am tetsing in OnePlus, Letv and Gionee devices.
-
Arun over 7 yearsPlease test the same on Nexus emulator, is it working ? Also please check if there is any check in the notification utils for app in foreground/background, if you have notification utils.
-
Arun over 7 yearsLet us continue this discussion in chat.
-
Krishan over 7 yearsAll of you are partially correct. I was facing exactly same issue when i was sending message using firebase console. onMessageReceived doesn't call when app is in background or killed. But i send using API onMessageReceived is always called. i downloaded Advanced Rest client for Chrome and started sending notification data. onMessageReceived is called every time. Thanks to below tutorial androidbash.com/firebase-push-notification-android
-
Milos Ivanovic over 6 yearsYes, @Krishan is right. The documentation is clear once you're looking in the right place. When a FCM message contains a "notification" payload, and the app is in the background, then onMessageReceived does not get called. If it contains a "data" payload, then it is called, even if it's in the background (in case it's killed or not running, it's started, and then onMessageReceived is invoked). Here is the official explanation (this should be included in the answer): firebase.google.com/docs/cloud-messaging/android/receive
-
Suresh over 6 yearsCheck this post for image notifications in background sureshsala.blogspot.in/2018/01/…
-
Swapnil over 5 yearsThis is the correct answer and should be marked as correct.
-
Rahul Khurana over 5 yearsI have tested the same Using Messaging API from AdvancedREST Client. See this blog
-
A2N about 5 yearsthis is not working all the times. seems service gets finished
-
Satish Gupta almost 5 yearswhat is post url?
-
afe over 4 yearsThis does not work for me using node-gcm for backend and Ionic 5 for frontend.