Firebase (FCM) registration token in Flutter
Solution 1
Add this to your package's pubspec.yaml file:
dependencies:
firebase_messaging: ^6.0.16
You can install packages from the command line:
with Flutter:
$ flutter packages get
Now in your Dart code, you can use:
import 'package:firebase_messaging/firebase_messaging.dart';
Implementation:
FirebaseMessaging _firebaseMessaging = FirebaseMessaging();
@override
void initState() {
super.initState();
firebaseCloudMessaging_Listeners();
}
void firebaseCloudMessaging_Listeners() {
if (Platform.isIOS) iOS_Permission();
_firebaseMessaging.getToken().then((token){
print(token);
});
_firebaseMessaging.configure(
onMessage: (Map<String, dynamic> message) async {
print('on message $message');
},
onResume: (Map<String, dynamic> message) async {
print('on resume $message');
},
onLaunch: (Map<String, dynamic> message) async {
print('on launch $message');
},
);
}
void iOS_Permission() {
_firebaseMessaging.requestNotificationPermissions(
IosNotificationSettings(sound: true, badge: true, alert: true)
);
_firebaseMessaging.onIosSettingsRegistered
.listen((IosNotificationSettings settings)
{
print("Settings registered: $settings");
});
}
For more details step by information please refer this link
Hope this helps you
Solution 2
With firebase_messaging: ^10.0.0
, you can directly get the token using
String? token = await FirebaseMessaging.instance.getToken();
or
FirebaseMessaging.instance.getToken().then((value) {
String? token = value;
});
Solution 3
As you can the use the firebase Messaging Plugin to send the Notification. Through this code you can print the Token in Console.
final FirebaseMessaging _firebaseMessaging = FirebaseMessaging();
_firebaseMessaging.configure(
onLaunch: (Map<String, dynamic> message) {
print('onLaunch called');
},
onResume: (Map<String, dynamic> message) {
print('onResume called');
},
onMessage: (Map<String, dynamic> message) {
print('onMessage called');
},
);
_firebaseMessaging.subscribeToTopic('all');
_firebaseMessaging.requestNotificationPermissions(IosNotificationSettings(
sound: true,
badge: true,
alert: true,
));
_firebaseMessaging.onIosSettingsRegistered
.listen((IosNotificationSettings settings) {
print('Hello');
});
_firebaseMessaging.getToken().then((token) {
print(token); // Print the Token in Console
});
}
Solution 4
We need to add this package in pubspec.yaml file
firebase_messaging: ^4.0.0+1
Perform packages get
Now import this in your code
import 'package:firebase_messaging/firebase_messaging.dart';
Create instance of FirebaseMessaging
FirebaseMessaging _firebaseMessaging = FirebaseMessaging();
Now we just to add the function which I have created in the answer in the link below
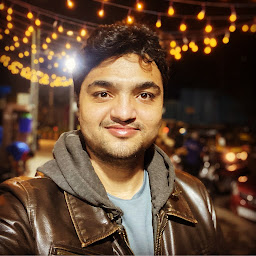
ajay datla
Updated on June 30, 2021Comments
-
ajay datla almost 3 years
I am trying to send notification from Java Rest Api (using Firebase Admin sdk) to my Flutter application and it seems it requires device token to send notification and I cannot find how to get that token. I am new to Flutter and android and may be missing any of the crucial step. Please help me if you can. Thanks.