Firebase messaging-handle background message in kotlin
6,493
Solution 1
Here is the working background notification kotlin code:
package com.example.yourapp
import io.flutter.app.FlutterApplication
import io.flutter.plugin.common.PluginRegistry
import io.flutter.plugin.common.PluginRegistry.PluginRegistrantCallback
import io.flutter.plugins.GeneratedPluginRegistrant
import io.flutter.plugins.firebasemessaging.FlutterFirebaseMessagingService
class Application : FlutterApplication(), PluginRegistrantCallback {
override fun onCreate() {
super.onCreate()
FlutterFirebaseMessagingService.setPluginRegistrant(this);
}
override fun registerWith(registry: PluginRegistry?) {
io.flutter.plugins.firebasemessaging.FirebaseMessagingPlugin.registerWith(registry?.registrarFor("io.flutter.plugins.firebasemessaging.FirebaseMessagingPlugin"));
}
}
Solution 2
Here is the Kotlin code for the new firebase cloud messaging version:
package id.your.app
import io.flutter.app.FlutterApplication
import io.flutter.plugin.common.PluginRegistry
import io.flutter.plugin.common.PluginRegistry.PluginRegistrantCallback
import io.flutter.plugins.firebase.messaging.FlutterFirebaseMessagingBackgroundService
// import io.flutter.plugins.firebase.messaging.FlutterFirebaseMessagingPlugin
class Application : FlutterApplication(), PluginRegistrantCallback {
override fun onCreate() {
super.onCreate()
FlutterFirebaseMessagingBackgroundService.setPluginRegistrant(this)
}
override fun registerWith(registry: PluginRegistry?) {
// FlutterFirebaseMessagingPlugin.registerWith(registry?.registrarFor("io.flutter.plugins.firebase.messaging.FlutterFirebaseMessagingPlugin"))
}
}
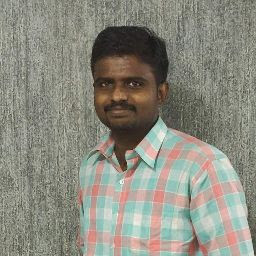
Author by
MSARKrish
I Interested in working on Android, Now i'm fall in love with Flutter. I have two Apps in Google Play Store. CGPA Calculator New Hand Cricket New
Updated on December 17, 2022Comments
-
MSARKrish over 1 year
I'm using firebase_messaging in my flutter application. To handle background messages with firebase messaging in pub they suggested to create new Application.java file and replace java file name in AndroidManifest file.
In my application i'm using kotlin and i already implemented some native code in MainActivity.kt
So how to write this code in kotlin.
package io.flutter.plugins.firebasemessagingexample; import io.flutter.app.FlutterApplication; import io.flutter.plugin.common.PluginRegistry; import io.flutter.plugin.common.PluginRegistry.PluginRegistrantCallback; import io.flutter.plugins.GeneratedPluginRegistrant; import io.flutter.plugins.firebasemessaging.FlutterFirebaseMessagingService; public class Application extends FlutterApplication implements PluginRegistrantCallback { @Override public void onCreate() { super.onCreate(); FlutterFirebaseMessagingService.setPluginRegistrant(this); } @Override public void registerWith(PluginRegistry registry) { GeneratedPluginRegistrant.registerWith(registry); } }
it is mandatory to replace MainActivity to Application in AndroidManifest file?
-
Isac Moura over 3 yearsYou also can do this way:
FirebaseMessagingPlugin.registerWith(registry?.registrarFor("io.flutter.plugins.firebasemessaging.FirebaseMessagingPlugin"))
-
Mahesh Jamdade about 3 yearsdo we have to add this in MainActivity.kt or create a separate file?
-
Xcode almost 3 yearsCreate a seperate file called Application.kt, then proceed to your AndroidManifest file in the application section use android:name=".Application"