(Flutter) Curved edge to a CustomPaint Widget
2,733
Solution 1
This isn't perfect, but you can fiddle with the numbers a bit more:
class LogoPainter extends CustomPainter {
@override
void paint(Canvas canvas, Size size) {
Paint paint = Paint();
paint.color = Colors.blue;
var path = Path();
path.lineTo(0, size.height - size.height / 5);
path.lineTo(size.width / 1.2, size.height);
//Added this line
path.relativeQuadraticBezierTo(15, 3, 30, -5);
path.lineTo(size.width, size.height - size.height / 5);
path.lineTo(size.width, 0);
path.close();
canvas.drawPath(path, paint);
}
@override
bool shouldRepaint(CustomPainter oldDelegate) {
return true;
}
}
Solution 2
You can do it this way
class LogoPainter extends CustomPainter {
@override
void paint(Canvas canvas, Size size) {
Paint paint = Paint();
paint.color = Colors.blue;
Path path = new Path();
path.lineTo(0, size.height - size.height / 5);
//Use the method conicTo
path.conicTo(size.width / 1.2, size.height, size.width,
size.height - size.height / 5, 15);
path.lineTo(size.width, 0);
path.close();
canvas.drawPath(path, paint);
}
@override
bool shouldRepaint(covariant CustomPainter oldDelegate) {
return true;
}
}
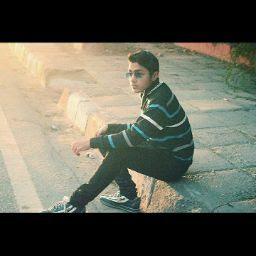
Author by
Aman Kataria
Updated on November 21, 2022Comments
-
Aman Kataria over 1 year
This is what I want to build:
(Just see the shape of the
appBar
and not the contents)This is what I have:
I want the edge to be curved, and not so sharp.
Here is my code for the
CustomPaint
:class LogoPainter extends CustomPainter { @override void paint(Canvas canvas, Size size) { Paint paint = Paint(); paint.color = Colors.blue; var path = Path(); path.lineTo(0, size.height - size.height / 5); path.lineTo(size.width / 1.2, size.height); path.lineTo(size.width, size.height - size.height / 5); path.lineTo(size.width, 0); path.close(); canvas.drawPath(path, paint); } @override bool shouldRepaint(CustomPainter oldDelegate) { return true; } }
How do I achieve this curved edge?
I have tried
point.arcToPoint()
andpoint.quadraticBezierTo()
, but no success.