Flutter: Fatal Error: Callback lookup failed! (with audioplayers package)
Solution 1
Looks like a bug where "Fatal Error: Callback lookup failed!" is printed in the logs if no callback method was defined via monitorNotificationStateChanges
.
By reading the source code it seems like this doesn't have any incidence.
But a way to avoid it is indeed to set that callback, and it has to be defined outside your class like so:
import 'package:audioplayers/audioplayers.dart';
class YourStatefulWidget extends StatefulWidget {
@override
_YourStatefulWidgetState createState() => _YourStatefulWidgetState();
}
class _YourStatefulWidgetState extends State<YourStatefulWidget> {
...
@override
void initState() {
super.initState();
if (Platform.isIOS) {
// to avoid getting "Fatal Error: Callback lookup failed!"
audioPlayer.monitorNotificationStateChanges(audioPlayerHandler);
}
}
...
}
// must be defined globally
void audioPlayerHandler(AudioPlayerState value) => null;
Solution 2
I faced the same issue, so this works for my game code, I hope this works for you, first, create a Controller Class like this:
import 'package:audioplayers/audio_cache.dart';
import 'package:audioplayers/audioplayers.dart';
void audioPlayerHandler(AudioPlayerState value) => print('state => $value');
class GameController {
static AudioPlayer audioPlayer = AudioPlayer();
static AudioCache audioCache = AudioCache();
static void play(String sound) {
if (!kIsWeb && Platform.isIOS) {
audioPlayer.monitorNotificationStateChanges(audioPlayerHandler);
}
audioCache.play(sound);
}
}
Then use your code by this way:
FlatButton(
onPressed: () => {
GameController.play('note1.wav');
}
child: Text('Click to ding!'),
),
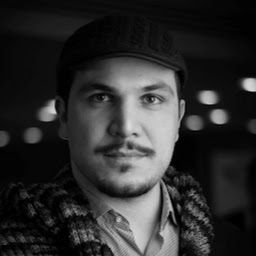
Amir Noureddini
Updated on November 25, 2022Comments
-
Amir Noureddini over 1 year
The following snippet is the button by clicking which, a short beep plays:
FlatButton( onPressed: () => { final player = new AudioCache(); player.play('note1.wav'); } child: Text('Click to ding!'), ),
The problem I'm facing is whenever I click this button, although sound plays correctly, I get this error in terminal:
flutter: Fatal Error: Callback lookup failed!
I wanna know what it is and what I should do?I checked the package's issue page but didn't find anything helpful.
Thanks
-
buckleyJohnson over 3 yearsThanks, that fixed it. Although I needed to remove the curly braces and the semi colon in the flat button.