(Flutter) How to launch static html page instead of URL if there is no internet connection?
Solution 1
The solution provided by @Mazin Ibrahim in the comments above worked for me.
So I am posting the solution here:
FutureBuilder(
future: check(), // a previously-obtained Future or null
builder: (BuildContext context, AsyncSnapshot<dynamic> snapshot) {
if (connectionStatus == true) {
//if Internet is connected
return SafeArea(
child: WebviewScaffold(
url: "http://www.duevents.in"))}
else{
//If internet is not connected
return SafeArea(
child: WebviewScaffold(
url: Uri.dataFromString('<html><body>hello world</body></html>',
mimeType: 'text/html').toString()) }})
Solution 2
I would suggest you to change the check()
method to return the URL directly.
Future<String> getURL() async {
try {
final result = await InternetAddress.lookup('google.com');
if (result.isNotEmpty && result[0].rawAddress.isNotEmpty) {
return "http://www.duevents.in";
}
} on SocketException catch (_) {
return Uri.dataFromString('<html><body>hello world</body></html>', mimeType: 'text/html').toString();
}
}
So then in the FutureBuilder
you could use the URL returned straight away.
FutureBuilder(
future: getURL(), // a previously-obtained Future or null
builder: (BuildContext context, String url) {
return SafeArea(
child: WebviewScaffold(
url: url))}
})
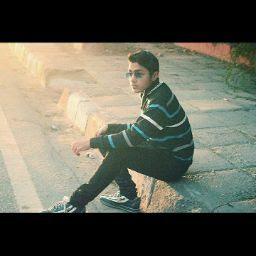
Aman Kataria
Updated on December 10, 2022Comments
-
Aman Kataria over 1 year
I have flutter_webview_plugin installed. I am trying to launch a custom static html page instead of my URL(my website 'wwww.duevents.in') if there is no internet connection, since the "Web Page not available" page doesn't look very professional.
I am using this to check the internet on the device and it is working fine ('connectionStatus ==true' when the internet is connected and vice versa):
Future check() async { try { final result = await InternetAddress.lookup('google.com'); if (result.isNotEmpty && result[0].rawAddress.isNotEmpty) { connectionStatus = true; print("connected $connectionStatus"); } } on SocketException catch (_) { connectionStatus = false; print("not connected $connectionStatus"); }
}
This is the code where I have alternate URLs to load if there is no internet connection:
WebviewScaffold( url: connectionStatus == true ?"http://www.duevents.in" : Uri.dataFromString('<html><body>hello world</body></html>', mimeType: 'text/html').toString())
Somehow it always shows me the HTML Page with this code no matter if the device has an internet connection or not. Please tell me what is wrong here.
-
Mazin Ibrahim about 5 yearsDid you try using a
FutureBuilder
? -
Aman Kataria about 5 years@Mazin No. where do I need it here?
-
Mazin Ibrahim about 5 yearsthis solution will help you implement
FutureBuilder
so you can check for connection status only after it is fetched stackoverflow.com/questions/54634418/… -
Aman Kataria about 5 yearswhat should I assign to 'future:'? P.S. I apologize for asking a lot of questions, I am new to this stuff, so i Know very little.
-
Mazin Ibrahim about 5 yearsYour
check()
method. So it'll befuture: check(),
-