Flutter: How to Refresh token when token expires during http call?
7,013
Solution 1
You can use refresh token as follows:
User getUser(){
final response = http.post(Uri.https(BASE_URL, '/api/user'),
headers: {'Authorization: Bearer $token'});
if(response.statusCode == 200){
return User.fromJson(jsonDecode(response.body)['user']);
}
else if(response.statusCode == 401){
//refresh token and call getUser again
final response = http.post(Uri.https(BASE_URL, '/api/[YourAuthorizationEndpoint]'),
headers: {'grant_type': 'refresh_token', 'refresh_token': '$refresh_token'});
token = jsonDecode(response.body)['token'];
refresh_token = jsonDecode(response.body)['refresh_token'];
return getUser();
}
}
Solution 2
You can use dart's http/retry
package:
import 'package:http/http.dart' as http;
import 'package:http_retry/http_retry.dart';
final client = RetryClient(
http.Client(),
retries: 1,
when: (response) {
return response.statusCode == 401 ? true : false;
},
onRetry: (req, res, retryCount) {
if (retryCount == 0 && res?.statusCode == 401) {
// refresh token
}
},
);
try {
final response = await client.get('http://www.example.com');
} finally {
client.close();
}
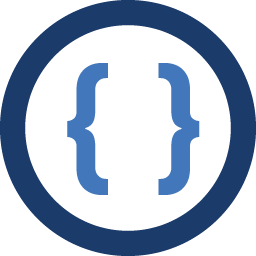
Author by
Admin
Updated on December 29, 2022Comments
-
Admin over 1 year
I am new to flutter and I am using http package for network call. I want to refresh token and call the request again if the response code of the request 200. How can I acheive this using http package? I heard about dio package but it is complicated for me.
User getUser(){ final response = http.post(Uri.https(BASE_URL, '/api/user'), headers: {'Authorization: Bearer $token'}); if(response.statusCode == 200){ return User.fromJson(jsonDecode(response.body)['user']); } else if(response.statusCode == 401){ //refresh token and call getUser again } }
-
Naveed Jamali over 2 yearsError 401 is for Un-authorize, but it does not only means that the Token is expired. What if the Token is not expired BUT the user is not authorized to access the resources due to some other reasons? Then what strategy should be applied to know if 401 was returned due to Token expiry or some other reason?
-
Jeremy over 2 yearsYou have access to the
response.body
so you can examine it to check the response. Then you would just modify the code to check for the body response criteria. -
Lemayzeur over 2 yearsWhat about when the refresh endpoint also returns Unauthorized 401? that'll be an infinite loop