Flutter : how to show next index after complete a specific logic in Swiper, where GridView also set in Swiper?
so first thing you should do is changing your onTap function in your gesture detector into a simpler code You shouldn't verify every single number for the index because the index is already that number
To be more clear when you call list[index] the index here is an integer so if the index==1 you're calling list[1] and if the index==5 you're calling list[5] you don't have to test if index==1 or something like that
so your code should be something like this
onTap: () async{
if (gameButtonList[index] == oneValueRandomGet){
_answer = true;
colorList[index] = Colors.green;
inLastPage = false;
setState((){});
_showCorrectAndIncorrectDialog("Congratulation", "asset/icon_images/ok_emoji.png", "Correct answer", Colors.green);
}else{
colorList[index] = Colors.red;
inLastPage = true;
setState((){});
}
},
And next for the problem of testing if the answer is correct or not and color changing and going to next screen
First thing please move these lines in your item builder function into a function you can call from anywhere like this for example
void newQuestion(){
gameButtonList.clear();
var fourValueRandom = new Random();
for (var i = 0; i < 4; i++) {
final fourGameBtnRandom = word_data.drink[fourValueRandom.nextInt(word_data.drink.length)];
gameButtonList.add(fourGameBtnRandom);
}
oneValueRandomGet = gameButtonList[fourValueRandom.nextInt(gameButtonList.length)];
wordDataReplace = oneValueRandomGet.replaceAll(" ", "_").toLowerCase();
}
and you can call this question after calling your dialogAlert if you change the line when you call _showCorrectAndIncorrectDialog(...) into
_showCorrectAndIncorrectDialog("Congratulation", "asset/icon_images/ok_emoji.png", "Correct answer", Colors.green);
newQuestion() //**Add this line also**
Notes :
-Remember to declare the variables you need in your class so they get changed in newQuestion function
-First time you lunch the app the variables like " oneValueRandomGet " are gonna be null so you can't show any data, call oneQuestion() in your initState so that when launching the app you get your first question ready directly.
I hope all this doesn't confuse you I tried my best to simplify and give you the simplest edit and answer possible, please if you're still unable to fix your problem I would really advice you to try to rewrite your code and try to make it as simple as possible.
Thank you.
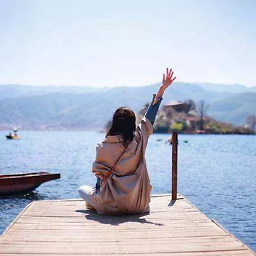
Emma Scarlett
Updated on December 17, 2022Comments
-
Emma Scarlett 6 months
I'm trying to make a word game. First of all, the index will be white. if user Click the correct answer then index will be to green color and go to next screen, also index will be white in next screen.. again if user click incorrect answer then index will be to red color and don't let go to the next page until user put correct answer...
i set a GridView in Swiper (Swiper took by importing, import 'package:flutter_swiper/flutter_swiper.dart';). and i want to show next index of Swiper after completing a logic in GridView. suppose, i have a long string list(array) and took four value string from this list(array) by random, this four values string set in GridView index.
Again i make a new string list(array) by this four value string and took a value from this new string list(array) by random, this single string set in Swiper. finally if user can select the Swiper index value to the GridView four index value correctly, then user can see next screen in Swiper. but output is not working properly. problem is - at first i set white color in GridView index, if it is correct answer should be green color in GridView index and incorrect will be red color in GridView index. here is my logic made it messy.
import 'package:flutter_swiper/flutter_swiper.dart'; import 'dart:math'; class GameWordRoute extends StatefulWidget { @override _GameWordRouteState createState() => _GameWordRouteState(); } class _GameWordRouteState extends State<GameWordRoute> { SwiperController _controller = SwiperController(); SwiperControl _control = SwiperControl(color: Colors.white); double get _width => MediaQuery.of(context).size.width; double get _height => MediaQuery.of(context).size.height; bool inLastPage = true; bool _answer = false; List<Color> colorList = <Color>[Colors.white, Colors.white, Colors.white, Colors.white,]; List<String> gameButtonList = <String>[]; FlutterTts flutterTts = FlutterTts(); @override Widget build(BuildContext context) { var sizeDevice = MediaQuery.of(context).size; final orientation = MediaQuery.of(context).orientation; final double itemHeight = sizeDevice.width / 6; final double itemWidth = sizeDevice.width / 2; return Scaffold( backgroundColor: Colors.purple, // white body: SafeArea( child: Column( children: <Widget>[ Expanded( flex: 1, child: Container( color: Colors.lightBlueAccent, )), Expanded( flex: 8, child: Container( color: Colors.cyan, child: Swiper( controller: _controller, loop: false, scrollDirection: Axis.horizontal, itemCount: word_data.drink.length, onIndexChanged: (value) { if (value == word_data.drink.length - 1) setState(() { inLastPage = true; }); else { setState(() { inLastPage = true; // false }); } }, itemBuilder: (BuildContext context, int index) { gameButtonList.clear(); var fourValueRandom = new Random(); for (var i = 0; i < 4; i++) { final fourGameBtnRandom = word_data.drink[fourValueRandom.nextInt(word_data.drink.length)]; gameButtonList.add(fourGameBtnRandom); } var oneValueRandom = new Random(); var oneValueRandomGet = gameButtonList[oneValueRandom.nextInt(gameButtonList.length)]; var wordDataReplace = oneValueRandomGet.replaceAll(" ", "_").toLowerCase(); return Container( child: Column( children: <Widget>[ Expanded( flex: 8, child: Container( color: Colors.purple, width: _width, alignment: Alignment.center, child: Padding( padding: const EdgeInsets.all(10.0), child: Image.asset("asset/drink_images/" + wordDataReplace + ".png", fit: BoxFit.contain, ), ), )), Expanded( flex: 1, child: Container( color: Colors.yellow, width: _width, alignment: Alignment.center, // "${categoryTitleArray[index]}" child: Text("What is this?"), )), Expanded( flex: 4, child: Container( color: Colors.yellow[200], width: _width, alignment: Alignment.center, child: GridView.builder( padding: EdgeInsets.all(8), itemCount: 4, gridDelegate: SliverGridDelegateWithFixedCrossAxisCount( crossAxisCount: (orientation == Orientation.portrait) ? 2 : 4, crossAxisSpacing: 5, mainAxisSpacing: 5, childAspectRatio: (itemWidth / itemHeight), ), itemBuilder: (BuildContext context, int index) { return GestureDetector( onTap: () { if (index == 0) { if (gameButtonList[0] == oneValueRandomGet){ _answer = true; inLastPage = false; colorList[0] = Colors.green; setState((){}); _showCorrectAndIncorrectDialog("Congratulation", "asset/icon_images/ok_emoji.png", "Correct answer", Colors.green); }else{ colorList[0] = Colors.red; inLastPage = true; setState((){}); } } else if (index == 1) { if (gameButtonList[1] == oneValueRandomGet){ _answer = true; colorList[1] = Colors.green; setState((){}); _showCorrectAndIncorrectDialog("Congratulation", "asset/icon_images/ok_emoji.png", "Correct answer", Colors.green); inLastPage = false; }else{ colorList[1] = Colors.red; inLastPage = true; setState((){}); } } else if (index == 2) { if (gameButtonList[2] == oneValueRandomGet){ _answer = true; colorList[2] = Colors.green; setState((){}); _showCorrectAndIncorrectDialog("Congratulation", "asset/icon_images/ok_emoji.png", "Correct answer", Colors.green); inLastPage = false; }else{ colorList[2] = Colors.red; inLastPage = true; setState((){}); } } else if (index == 3) { if (gameButtonList[3] == oneValueRandomGet){ _answer = true; colorList[3] = Colors.green; inLastPage = false; setState((){}); _showCorrectAndIncorrectDialog("Congratulation", "asset/icon_images/ok_emoji.png", "Correct answer", Colors.green); }else{ colorList[3] = Colors.red; inLastPage = true; setState((){}); } } }, child: Container( child: new Card( elevation: 0, color: colorList[index], //Colors.transparent, child: Center( child: Text( "${gameButtonList[index]}", textAlign: TextAlign.center, style: TextStyle(color: Colors.black), ), ), ), ), ); }), )), ], ), ); }, ), ), ), Expanded( flex: 1, child: Container( color: Colors.lightBlueAccent, )), ], ), ), ); } void _showCorrectAndIncorrectDialog(String _title, String _image, String _subTitle, Color _color){ showDialog( context: context, builder: (BuildContext context){ Future.delayed(Duration(milliseconds: 500), () { Navigator.of(context).pop(true); }); return AlertDialog( title: Text(_title, textAlign: TextAlign.center, style: TextStyle(color: _color),), content: Container( height: _width/1.1, child: Column( children: <Widget>[ Expanded( flex: 4, child: Container( // color: Colors.cyan[100], child: Image.asset(_image, fit: BoxFit.cover, ), ), ), SizedBox(height: 8), Expanded( flex: 1, child: Container( color: Colors.cyan, child: Center( child: Text( _subTitle, style: TextStyle( // fontSize: 24, ), ), ), ), ), ], ), ), ); } ); } }
-
Zain Ur Rehman over 2 yearsunable to understand your logics, simplify your code and add comments. Also add details about the flow of this page.
-
Emma Scarlett over 2 years@ZainUrRehman i try to make a word game. if user Click the correct answer then index will green and go to next screen, this one is ok... again if user click incorrect then index will be to red, but problem is , when user click incorrect then it also go to next screen.... here i just want to go to next screen when user will click correct index.
-
-
Emma Scarlett over 2 yearsmissing question image, it automatically go to next screen in both false and true. also color does back to white, color should be white in next screen.
-
Oussama Belabbas over 2 yearsfor the color add this to newQuestion() for (var i = 0; i < colorsList; i++) { colorsList[index]=Colors.white; } setState((){}); //In last line of newQuestion()
-
Emma Scarlett over 2 yearssorry i am not realizing, can i send you the project? please give me you email address
-
Oussama Belabbas over 2 yearsOkay you can send me an email on [email protected] I'll try to rewrite your project and help you fixing it
-
Emma Scarlett over 2 yearsi sent you email brother