Flutter The argument type 'List<int>' can't be assigned to the parameter type 'Uint8List'
157
You can use Uint8List.fromList
to construct a Uint8List
from a List<int>
.
You alternatively could try using an explicit cast (e.g. list as Uint8List
) since a lot of code is declared to return List<int>
but actually returns Uint8List
objects. (In your case, assuming that you're using package:image
, after skimming through the documentation, encodePng
does appear to be one such case.) Using a cast would avoid creating an unnecessary copy (which could be potentially expensive).
I normally combine the approaches.
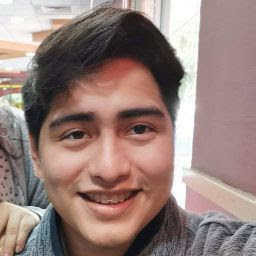
Author by
Daniel Camaja
Updated on December 19, 2022Comments
-
Daniel Camaja over 1 year
I have a code (not originally written by me) and, while trying to update it, I'm getting this error:
ui.encodePng(temp) "The argument type 'List<int>' can't be assigned to the parameter type 'Uint8List'"
This is the base code:
// create crop image for each block ui.Image temp = ui.copyCrop( fullImage, xAxis.round(), yAxis.round(), widthPerBlockTemp.round(), heightPerBlockTemp.round(), ); // get offset for each block show on center base later Offset offset = Offset(size.width / 2 - widthPerBlockTemp / 2, size.height / 2 - heightPerBlockTemp / 2); ImageBox imageBox = new ImageBox( image: Image.memory( ui.encodePng(temp), fit: BoxFit.contain, ), isDone: false, offsetCenter: offsetCenter, posSide: jigsawPosSide, radiusPoint: minSize, size: Size(widthPerBlockTemp, heightPerBlockTemp), ); images[y].add( new BlockClass( jigsawBlockWidget: JigsawBlockWidget( imageBox: imageBox, ), offset: offset, offsetDefault: Offset(xAxis, yAxis)), );
EDIT: okey, this is a code complete
Future<void> generaJigsawCropImage() async { // 1st we need create a class for block image // ignore: deprecated_member_use images = <List<BlockClass>>[]; // get image from out boundary if (fullImage == null) fullImage = await _getImageFromWidget(); // split image using crop int xSplitCount = 2; int ySplitCount = 2; // i think i know what the problom width & height not correct! double widthPerBlock = fullImage.width / xSplitCount; // change back to width double heightPerBlock = fullImage.height / ySplitCount; for (var y = 0; y < ySplitCount; y++) { // temporary images // ignore: deprecated_member_use //List tempImages = List<BlockClass>[]; images.add(<BlockClass>[]); for (var x = 0; x < xSplitCount; x++) { int randomPosRow = math.Random().nextInt(2) % 2 == 0 ? 1 : -1; int randomPosCol = math.Random().nextInt(2) % 2 == 0 ? 1 : -1; Offset offsetCenter = Offset(widthPerBlock / 2, heightPerBlock / 2); // make random jigsaw pointer in or out ClassJigsawPos jigsawPosSide = new ClassJigsawPos( bottom: y == ySplitCount - 1 ? 0 : randomPosCol, left: x == 0 ? 0 : -images[y][x - 1] .jigsawBlockWidget .imageBox .posSide .right, // ops.. forgot to dclare right: x == xSplitCount - 1 ? 0 : randomPosRow, top: y == 0 ? 0 : -images[y - 1][x].jigsawBlockWidget.imageBox.posSide.bottom, ); double xAxis = widthPerBlock * x; double yAxis = heightPerBlock * y; // this is culprit.. haha // size for pointing double minSize = math.min(widthPerBlock, heightPerBlock) / 15 * 4; offsetCenter = Offset( (widthPerBlock / 2) + (jigsawPosSide.left == 1 ? minSize : 0), (heightPerBlock / 2) + (jigsawPosSide.top == 1 ? minSize : 0), ); // change axis for posSideEffect xAxis -= jigsawPosSide.left == 1 ? minSize : 0; yAxis -= jigsawPosSide.top == 1 ? minSize : 0; // get width & height after change Axis Side Effect double widthPerBlockTemp = widthPerBlock + (jigsawPosSide.left == 1 ? minSize : 0) + (jigsawPosSide.right == 1 ? minSize : 0); double heightPerBlockTemp = heightPerBlock + (jigsawPosSide.top == 1 ? minSize : 0) + (jigsawPosSide.bottom == 1 ? minSize : 0); // create crop image for each block ui.Image temp = ui.copyCrop( fullImage, xAxis.round(), yAxis.round(), widthPerBlockTemp.round(), heightPerBlockTemp.round(), ); // get offset for each block show on center base later Offset offset = Offset(size.width / 2 - widthPerBlockTemp / 2, size.height / 2 - heightPerBlockTemp / 2); ImageBox imageBox = new ImageBox( image: Image.memory( ui.encodePng(temp), fit: BoxFit.contain, ), isDone: false, offsetCenter: offsetCenter, posSide: jigsawPosSide, radiusPoint: minSize, size: Size(widthPerBlockTemp, heightPerBlockTemp), ); images[y].add( new BlockClass( jigsawBlockWidget: JigsawBlockWidget( imageBox: imageBox, ), offset: offset, offsetDefault: Offset(xAxis, yAxis)), ); } } blocksNotifier.value = images.expand((image) => image).toList(); // let random a bit so blok puzzle not in incremet order // ops..bug .. i found culprit.. seem RepaintBoundary return wrong width on render..fix 1st using height // as well blocksNotifier.value.shuffle(); blocksNotifier.notifyListeners(); // _index = 0; setState(() {}); }
I'm new here so I didn't know how to edit until now,
-
Ujjawal Maurya about 2 yearsI think you are not decoding it. You should be more clear.
-
Mariano Zorrilla about 2 yearsThat code is old. Could you show what ImageBox needs as parameters? Also, Image.memory receives a Uint8List and not a List<int> like encodePng does.
-
-
Daniel Camaja about 2 yearsExcuse me, how can I implement it in my code, I just edited it to share the full function
-
jamesdlin about 2 yearsIn your case, you'd use
ui.encodePng(temp).asUint8List()
.