Flutter Webview map
You can try my plugin flutter_inappbrowser (EDIT: it has been renamed to flutter_inappwebview). You can load an url using initialUrl
, a file inside assets folder (see more here) using initialFile
or load an html string using initialData
directly.
An example of a google map inside the InAppWebView
is presented below:
...
child: InAppWebView(
initialFile: "assets/index.html",
initialHeaders: {},
initialOptions: InAppWebViewWidgetOptions(
inAppWebViewOptions: InAppWebViewOptions(
debuggingEnabled: true,
),
),
onWebViewCreated: (InAppWebViewController controller) {
webView = controller;
},
onLoadStart: (InAppWebViewController controller, String url) {
},
onLoadStop: (InAppWebViewController controller, String url) {
},
onConsoleMessage: (InAppWebViewController controller, ConsoleMessage consoleMessage) {
print("console message: ${consoleMessage.message}");
},
),
...
The index.html
file inside the assets folder (example taken from here):
<!DOCTYPE html>
<html>
<head>
<style>
/* Set the size of the div element that contains the map */
#map {
height: 400px; /* The height is 400 pixels */
width: 100%; /* The width is the width of the web page */
}
</style>
</head>
<body>
<h3>My Google Maps Demo</h3>
<!--The div element for the map -->
<div id="map"></div>
<script>
// Initialize and add the map
function initMap() {
// The location of Uluru
var uluru = { lat: -25.344, lng: 131.036 };
// The map, centered at Uluru
var map = new google.maps.Map(document.getElementById("map"), {
zoom: 4,
center: uluru
});
// The marker, positioned at Uluru
var marker = new google.maps.Marker({ position: uluru, map: map });
}
</script>
<!--Load the API from the specified URL
* The async attribute allows the browser to render the page while the API loads
* The key parameter will contain your own API key (which is not needed for this tutorial)
* The callback parameter executes the initMap() function
-->
<script async defer src="https://maps.googleapis.com/maps/api/js?key=YOUR_API_KEY&callback=initMap"></script>
</body>
</html>
where YOUR_API_KEY
is your google map key.
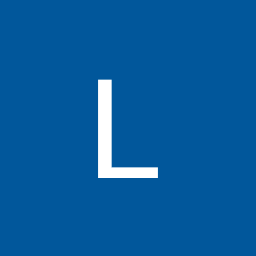
LukasCodes
Updated on December 15, 2022Comments
-
LukasCodes 5 months
I'm having a big map issue when I clicked the image button in the application bar. It looks like the webview has lost focus and is somehow locked and when I move the map, it acts like a zooming, but I only use one finger and want to move the map. I also have it in Stack view. But it works well on an Android phone / tablet.
I'm using webview_flutter: ^0.3.15+1.
It work well again if I reload map via controller, but I lost all map settings which I made before. Is there some focus-again widget or something like this?
Thanks a lot for help, I've been stuck with this for days.