How do I check Internet Connectivity using HTTP requests(Flutter/Dart)?
Solution 1
You should surround it with try catch block, like so:
import 'package:http/http.dart' as http;
int timeout = 5;
try {
http.Response response = await http.get('someUrl').
timeout(Duration(seconds: timeout));
if (response.statusCode == 200) {
// do something
} else {
// handle it
}
} on TimeoutException catch (e) {
print('Timeout Error: $e');
} on SocketException catch (e) {
print('Socket Error: $e');
} on Error catch (e) {
print('General Error: $e');
}
Socket exception will be raised immediately if the phone is aware that there is no connectivity (like both WiFi and Data connection are turned off).
Timeout exception will be raised after the given timeout, like if the server takes too long to reply or users connection is very poor etc.
Also don't forget to handle the situation if the response code isn't = 200.
Solution 2
You don't need to use http to check the connectivity yourself, simply use connectivity library
Solution 3
You can use this plugin https://pub.dev/packages/data_connection_checker
So you can check prior if you have the connection, if not give a alert to the user that no internet connection. And if you have the internet connection then just proceed to your fetching part.
I will just link some resources below where it has been explained perfectly:
https://www.youtube.com/watch?v=u_Xyqo6lhFE
This is all things will be done prior to making an http call, but what if while making an http call the internet goes off then you can use the try catch block which @uros has mentioned.
Let me know if it works.
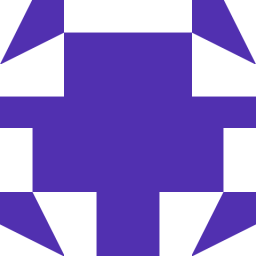
TB_98
Updated on November 21, 2022Comments
-
TB_98 6 months
This is probably a noob question, but how do I make my response throw an exception if the user does not have an internet connection or if it takes too long to fetch the data?
Future<TransactionModel> getDetailedTransaction(String crypto) async { //TODO Make it return an error if there is no internet or takes too long! http.Response response = await http.get(crypto); return parsedJson(response); }
-
TB_98 about 3 yearsThat solution is good if you want to check data connection throughout your app, but I just wanted connection to be checked within that one fetch method. Thanks for the help!
-
TB_98 about 3 yearsSorry for the long wait. Thanks a lot it works perfectly!
-
TB_98 about 3 yearsThanks for the input! I'm using the BLoC design pattern for this project, so this solution did not end up being compatible w/ my project.
-
Fabio Pagano about 2 yearsThe original poster asked how to check for internet connection, but the connectivity library package page in pub.dev/packages/connectivity expressely states that "on Android, this does not guarantee connection to Internet. For instance, the app might have wifi access but it might be a VPN or a hotel WiFi with no access.". Therefore this answer doesn't solve the poster question.
-
s.j about 2 years@Uros but phone connected to wifi still getting socket exception error.
-
Ehsan Askari over 1 yearHaving no internet connection is only one of the reasons why the
SocketException
thrown. I mean there are some other problems that my cause theSocketException
to be thrown. Is there any way to precisely identify if the cause is having no internet connection?