for loop, iteration through alphabet? java
Solution 1
You need to add the char alphabet
to a string.
String output = "";
for(char alphabet = 'a'; alphabet <='z'; alphabet++ )
{
output += alphabet;
System.out.println(output);
}
This should work for you ;)
Solution 2
I will go with StringBuffer or StringBuilder. Something like:
StringBuffer
StringBuffer sb = new StringBuffer();
for (char alphabet = 'a'; alphabet <= 'z'; alphabet++) {
sb.append(alphabet);
System.out.println(sb.toString());
}
StringBuilder
StringBuilder sb = new StringBuilder();
for (char alphabet = 'a'; alphabet <= 'z'; alphabet++) {
sb.append(alphabet);
System.out.println(sb.toString());
}
String vs StringBuffer vs StringBuilder:
String: It is immutable, so when you do any modification in the string, it will create new instance and will eatup memory too fast.
StringBuffer: You can use it to create dynamic String and at the sametime only 1 object will be there so very less memory will be used. It is synchronized (which makes it slower).
StringBuilder: It is similar to StringBuffer. The olny difference is: it not synchronized and hence faster.
So, better choice would be StringBuilder. Read more.
Using Java 8
StringBuilder sb = new StringBuilder();
IntStream.range('a', 'z').forEach(i -> {
sb.append((char) i);
System.out.println(sb.toString());
});
Solution 3
I'd suggest using a StringBuilder
:
// Using StringBuilder
StringBuilder buf = new StringBuilder();
for (char c = 'a'; c <= 'z'; c++)
System.out.println(buf.append(c).toString());
You could also do it slightly faster by using a char[]
, however StringBuilder
is more obvious and easier to use:
// Using char[]
char[] arr = new char[26];
for (int i = 0; i < 26; i++) {
arr[i] = (char)('a' + i);
System.out.println(new String(arr, 0, i + 1));
}
Alternatives that you shouldn't use:
-
StringBuffer
: Same asStringBuilder
, but synchronized, so slower. -
s = s.concat(new String(c))
: Allocates 2 Strings per iteration, instead of only 1. -
s += c
: Internally+=
is compiled tos = new StringBuilder().append(s).append(c).toString()
, so horrendously slow with exponential response times.
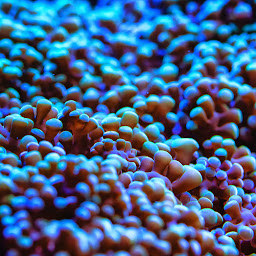
pewpew
Newby, only know a little html, css, and python. I will probably be asking lots of questions. :)
Updated on October 07, 2021Comments
-
pewpew over 2 years
I can iterate through the alphabet, but I'm trying to keep the last iteration and add on the next letter. this is my code.
for(char alphabet = 'a'; alphabet <='z'; alphabet ++ ) { System.out.println(alphabet); }
I want it to print out something that looks like this.
a
ab
abc
abcd
abcde..... and so forth. How is this possible?
-
Andreas over 8 yearsNever use
String += String
in a loop. UseStringBuilder
. Sure, this is a small inconsequential loop, but start doing it the right way, so it becomes second nature. -
pewpew over 8 yearsi edited like 2 seconds after i posted lol! such fast response time haha. One thing to note. the output += alphabet needs to be inside the print statement System.out.println(output += alphabet);
-
Andreas over 8 years
StringBuffer
is obsolete. UseStringBuilder
. Quoting javadoc: "As of release JDK 5,StringBuffer
has been supplemented with an equivalent class designed for use by a single thread,StringBuilder
." -
Stefan over 8 yearsI don't think that has to be in the System.out. Why do you think so?
-
Ambrish over 8 yearsI have added both in my solution.
-
Andreas over 8 years@pewpew Sure that's shorter, but "needs to be"? No.
-
Rahman over 8 yearsI am assuming he will declare it outside of that for loop :). Anyways edited the answer
-
Andreas over 8 years
StringBuffer
is synchronized, which makes it slower. Unless you're in an extremely rare situation where multiple threads append to the same buffer, you should never use it. Always useStringBuilder
. Make it a habit. I'd suggest removingStringBuffer
from answer, so as not to misguide any newbie Java developers coming here. -
singhakash over 8 years@Andreas I dont suggest removing I would suggest the Ambrish should clarify the difference so that OP can choose any method accoding the requirement
-
pewpew over 8 yearsOk i see what you did there ;)
-
Ambrish over 8 years@Andreas I have added details and also better solution: Java8.
-
Andreas over 8 yearsJava 8 stream is a nice touch, but I disagree with calling it a "better solution". To me, it's actually slightly worse, but I'd be good with simply calling it "another" way of doing it.
-
Andreas over 8 years@pewpew Of all the answers, you accept the one with the worst performance of them all. It is a bit simpler to write than the rest, I'll give you that. It's a good thing there's only 26 letters in the alphabet.
-
Vogel612 over 8 yearsWhile this may theoretically answer the question, it's not really a good answer, since it doesn't teach the OP. Instead it gives an alternative solution without explanation. This will usually lead to OP not learning, and coming back for asking a new question when a similar problem occurs. Would you mind adding some explanation?